Golang Memcached Get (Detailed Guide w/ Code Examples)
Use Case(s)
The golang memcached get
is generally used when there's a need to retrieve data that has been stored in the Memcached caching system using Go (Golang). For instance, web applications often store results of complex database queries or HTML renderings in Memcached and fetch them using this method to improve performance.
Code Examples
Let's consider you're using the "github.com/bradfitz/gomemcache/memcache"
package for Memcached client. Here's how you can use memcached get
:
- Basic Example CODE_BLOCK_PLACEHOLDER_0
In this example, we first connect to our Memcached server running at localhost:11211. We then set a key-value pair {'foo': 'my value'} and then retrieve ('get') the value using the same key ('foo'). If the key is found, we print the key and its corresponding value, else we log the error.
Best Practices
- Always handle errors returned by the
Get
function. These errors could be due to connectivity issues or because the key doesn't exist. - Close the connection to the Memcached server when it's no longer needed. Although the Go Memcached client may handle this automatically, it's good practice to explicitly close connections.
Common Mistakes
- One common mistake is trying to retrieve a key that has not been set or has expired. The
Get
function will return an error in such cases. - Another common mistake is not handling potential errors when calling
Get
. Always check for errors and handle them appropriately.
FAQs
Q: What happens if I try to get a key that doesn't exist? A: If you attempt to get a value with a key that does not exist in the cache, the Get
function will return an error. It's important to handle these errors in your code.
Q: Can I store any type of value in Memcached? A: Memcached stores everything as byte arrays. So while you can technically store anything that can be represented as bytes, it's most commonly used to store strings or serializable objects.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
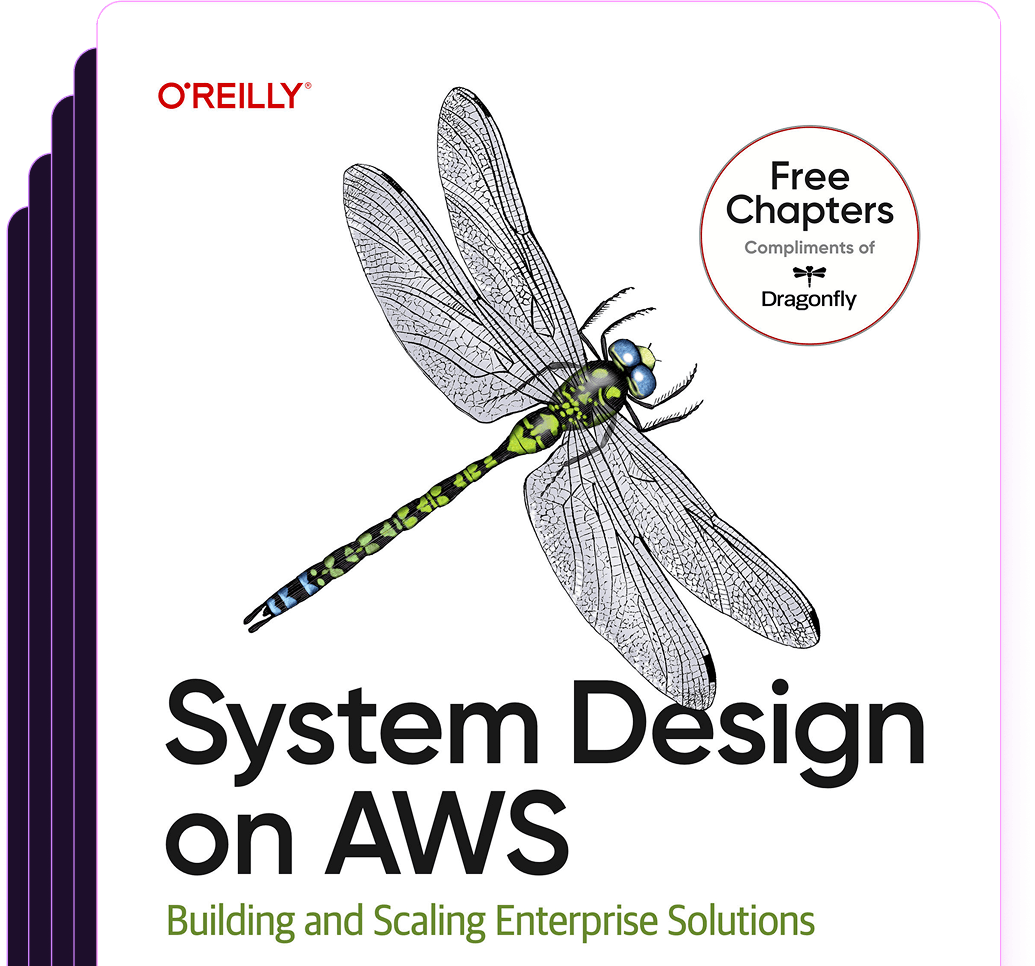
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost