Java Memcached CAS (Check And Set) (Detailed Guide w/ Code Examples)
Use Case(s)
The CAS (Check and Set) operation is a way to handle concurrent updates in Memcached. It provides a mechanism to ensure that the value of a key hasn't changed before updating it. This is useful when multiple threads or processes may be updating the same key, to avoid lost updates.
Code Examples
Let's look at a few examples in Java using the "spymemcached" client library:
- Retrieving a CAS value CODE_BLOCK_PLACEHOLDER_0
In this example, we first set a key, then retrieve it along with its CAS value using the gets
method. The CAS value can be used later to perform a CAS update.
- Performing a CAS update CODE_BLOCK_PLACEHOLDER_1
Here we use the cas
method to attempt a CAS update. If the value has not been modified by another process since we retrieved it, the update will succeed. Otherwise, it will fail.
Best Practices
- Always handle CAS failures. A failure could mean that another process has updated the value, so you'll need to decide how to handle that - possibly by retrying the operation or failing gracefully.
- Use Memcached's CAS operations judiciously. Overuse of CAS can lead to performance degradation as it requires more processing and network bandwidth.
Common Mistakes
- Not checking the result of a CAS operation. If you don't check whether the CAS update was successful or not, you may inadvertently work with stale data.
- Using CAS when it's not needed. Remember, CAS is about concurrency control. If you're sure that a key will only be updated by a single thread or process at any given time, you don't need to use CAS.
FAQs
1. Can CAS entirely prevent lost updates? Not completely. If two processes retrieve the same CAS value for a key, and then both try to update it, one will succeed and the other will fail. The second process will need to handle this failure, possibly by retrying the operation.
2. What happens if the key being updated with CAS doesn't exist? If you attempt a CAS operation on a key that doesn't exist, Memcached treats it as an add operation and adds the key-value pair.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
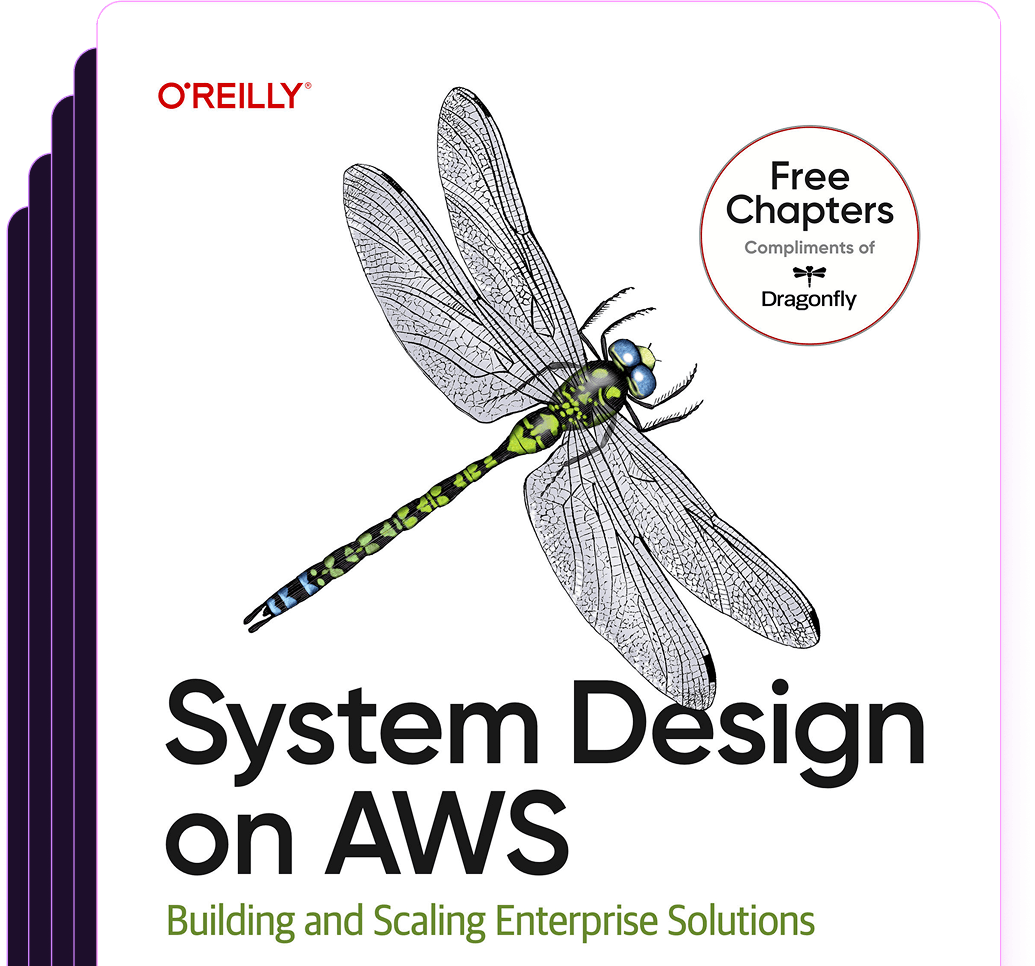
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost