Memcached Set in Python (Detailed Guide w/ Code Examples)
Use Case(s)
The set
command in Memcached is used to store a value on the server with an associated key. This operation is idempotent, meaning it can be repeated without changing the result beyond the initial application.
Common use cases include:
- Storing session information such as user preferences and shopping cart details.
- Caching database query results or frequently accessed static data.
Code Examples
Here are a couple of examples using the pymemcache client library for Python.
Example 1: Basic 'set' operation
from pymemcache.client import base
# Initialize memcached client
client = base.Client(('localhost', 11211))
# Set a key-value pair
client.set('key', 'value')
In this example, we connect to a Memcached server running locally, then set a key-value pair ('key', 'value').
Example 2: Setting multiple keys at once
from pymemcache.client import base
# Initialize memcached client
client = base.Client(('localhost', 11211))
# Set multiple key-value pairs
client.set_multi({'key1': 'value1', 'key2': 'value2'})
In this second example, we use the set_multi
method to set multiple key-value pairs at once.
Best Practices
- It's important to remember that Memcached is not persistent storage; it's a caching solution. Ensure that any data stored in Memcached is also stored permanently elsewhere.
- Keep your keys as descriptive as possible, which helps with debugging when necessary.
- Avoid storing large objects in Memcached. The maximum size of a value you can store is 1MB.
Common Mistakes
- A common mistake is ignoring the return value of the
set
command. It returns a boolean indicating success or failure, which can be useful for error handling. - Not understanding that Memcached evicts older data as it runs out of memory. If your application depends on certain data always being in the cache, you might run into unexpected behavior.
FAQs
1. Can I store Python objects in Memcached?
Yes, Memcached clients usually serialize objects before storing them, so you can store complex Python objects.
2. What happens when Memcached runs out of memory?
When Memcached runs out of memory, it starts evicting older items to make space for new ones.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
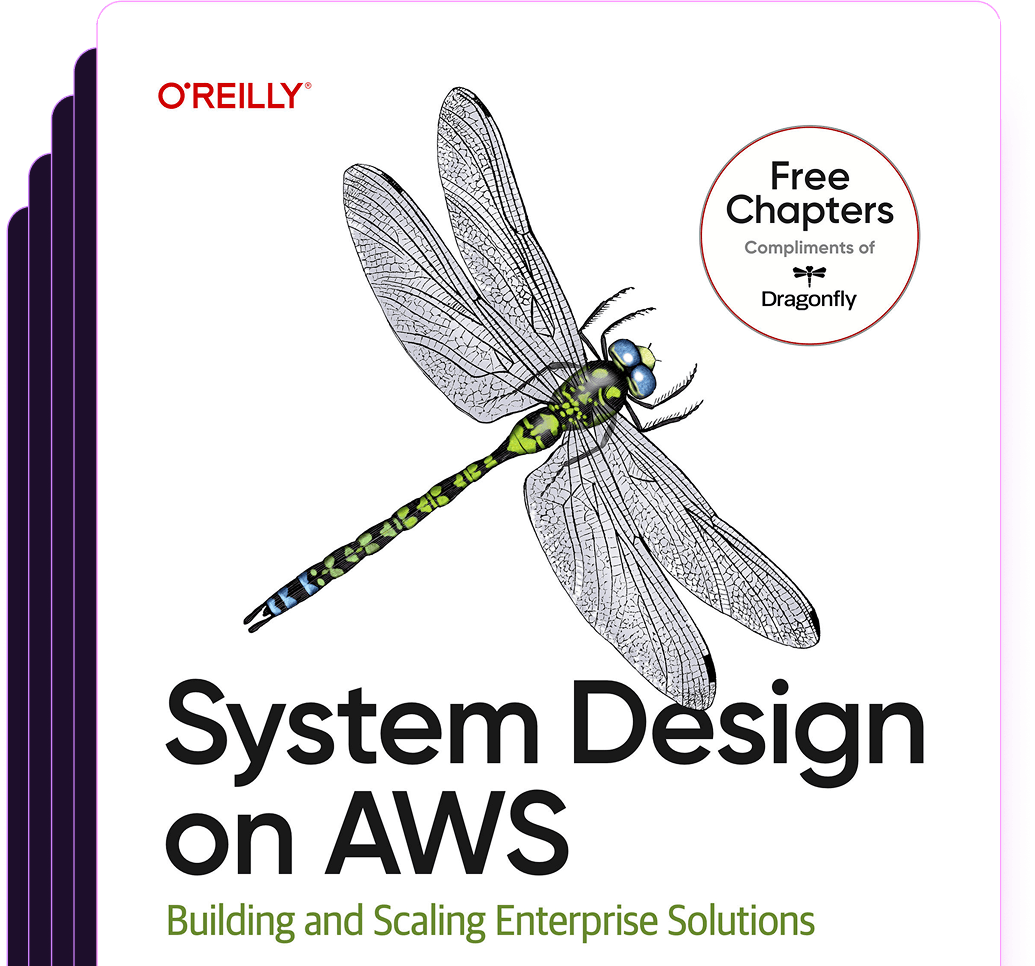
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost