Question: What is the difference between Redis sharding and clustering?
Answer
Redis sharding and clustering are both methods used to distribute data, but they serve different purposes and have contrasting functionalities.
Sharding is a technique that involves partitioning the data into smaller parts, or shards, which are then spread across multiple Redis instances. Each shard acts as an independent database, and the distribution of these shards can be based on various strategies such as key range or consistent hashing. Sharding allows for horizontal scaling by distributing load and storage capacity across many servers. However, managing sharded data can be complex because each shard is independent and there's no built-in mechanism to handle failures or resharding when needed.
Here's a simple example of how you might implement sharding manually in Python using redis-py
:
import redis
import hashlib
def get_redis_connection(key):
shard_id = int(hashlib.md5(key.encode('utf-8')).hexdigest(), 16) % num_shards
return redis.Redis(host=shard_hosts[shard_id], port=6379)
# Assume we have two shards
num_shards = 2
shard_hosts = ["127.0.0.1", "127.0.0.2"]
key = "my_key"
r = get_redis_connection(key)
r.set(key, "my_value")
On the other hand, Clustering is a feature built into Redis starting from version 3.0 that partitions data across multiple Redis nodes. Unlike sharding, Redis Cluster provides automatic sharding and comes with built-in support for replication, failure detection, and failover. It's designed to survive nodes failing without data loss or interruption of service, making it more resilient and reliable than manual sharding.
Here's an example of how you might use Redis Cluster in Python:
from rediscluster import RedisCluster
# Assumes a cluster has been set up with nodes on these three ports
startup_nodes = [{"host": "127.0.0.1", "port": "7000"}, {"host": "127.0.0.1", "port": "7001"}, {"host": "127.0.0.1", "port": "7002"}]
rc = RedisCluster(startup_nodes=startup_nodes, decode_responses=True)
rc.set("my_key", "my_value")
In summary, while both sharding and clustering are used to distribute data in Redis, sharding is a general technique that can be manually implemented, but lacks features such as automatic resharding and failure handling. Clustering, however, is a specific feature built into Redis that automatically handles sharding and provides fault tolerance.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common Redis Questions (and Answers)
- Is Redis free?
- How to install Redis on Mac?
- How to check if Redis is running?
- How to restart Redis?
- Does Redis persist data?
- How to install Redis on Ubuntu?
- How to stop Redis server?
- How to see Redis version?
- How long does Redis store data?
- How is Redis so fast?
- How to connect to Redis server?
- Is Redis Multithreaded?
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
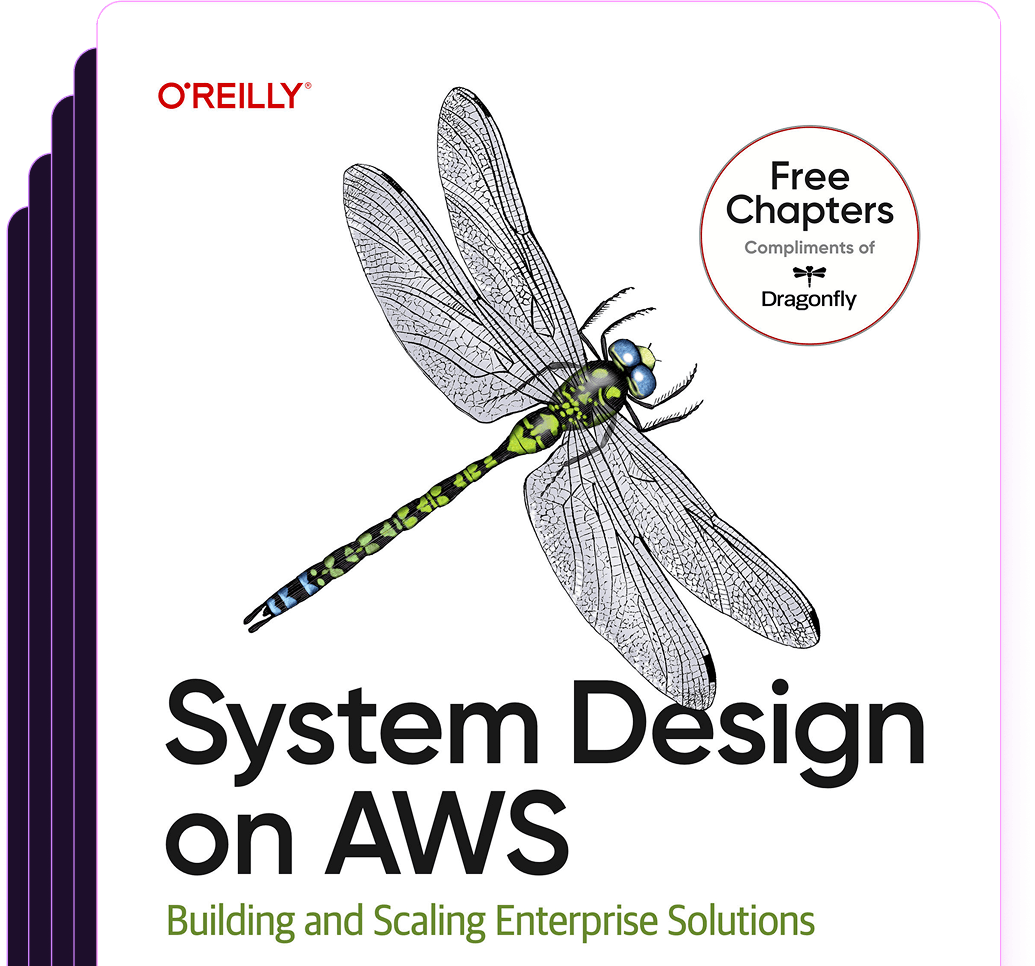
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost