Get All Keys Starting With a Specific Prefix in Redis using Golang (Detailed Guide w/ Code Examples)
Use Case(s)
Redis is often used to store key-value pairs where keys may have a common prefix that denotes some logical grouping. For instance, you might store user-related data with keys like user:1:name
, user:1:email
, user:2:name
, etc.
Sometimes you need to retrieve all keys starting with a specific prefix, such as user:1
in this case, to perform operations on related data.
Code Examples
Golang's go-redis
client provides powerful commands to interact with Redis. The Keys
command gets all keys matching a pattern. CODE_BLOCK_PLACEHOLDER_0
This code connects to a local Redis instance, and retrieves all keys starting with user:1
. Please replace "user:1" with the actual prefix you are using.
Best Practices
- Avoid using the
Keys
command in production environments for large databases because it may degrade performance. UseScan
instead. - Always handle errors returned from Redis commands.
Common Mistakes
- Overusing the
Keys
command in production which could lead to performance issues. - Not handling potential errors when executing Redis commands.
FAQs
Q: Can I use this method to get keys using other patterns, not just prefixes? A: Yes, the pattern argument in the Keys
can be any glob-style pattern supported by Redis.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
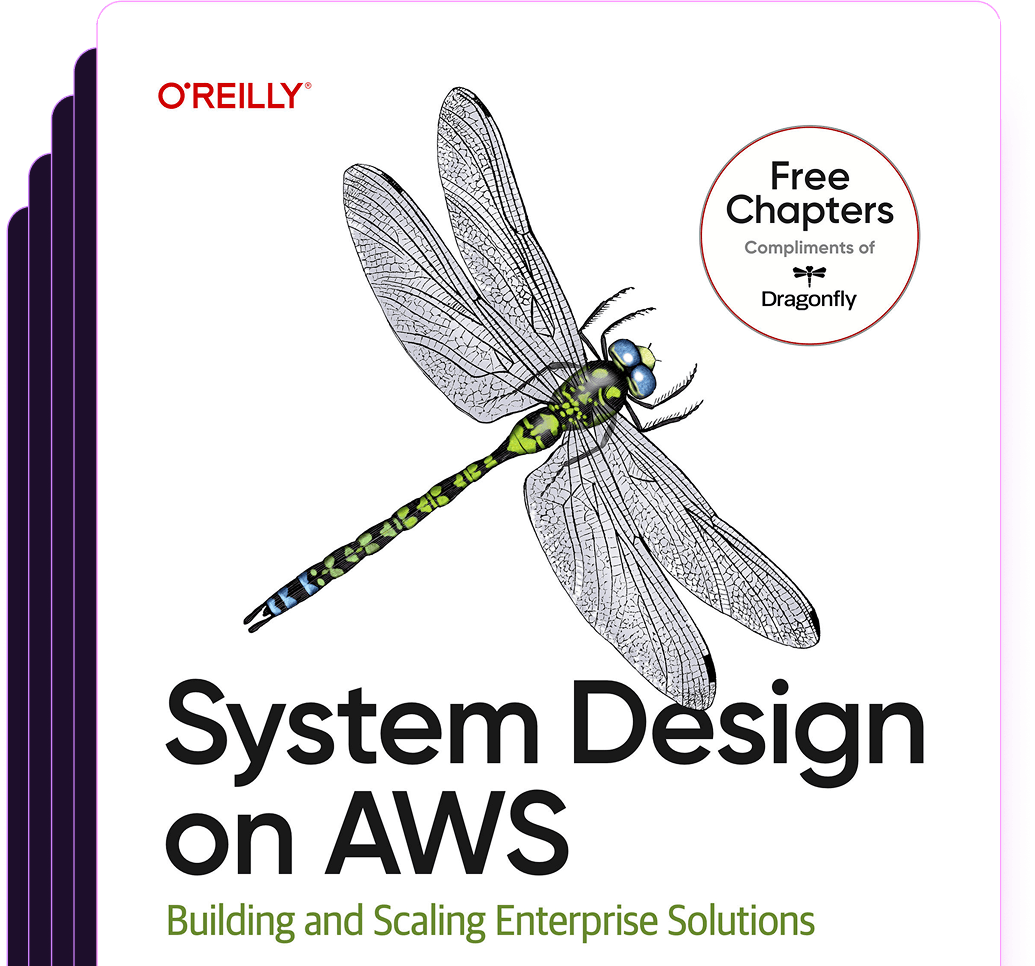
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost