Delete Redis Cache in Java (Detailed Guide w/ Code Examples)
Use Case(s)
Deleting Redis cache is a common operation in applications where you need to manage the cache lifecycle. This usually happens when data becomes obsolete or irrelevant, and you want to ensure your application uses the most up-to-date data.
Code Examples
Below is an example of how to delete keys from Redis using Jedis, a popular Java client for Redis:
```
import redis.clients.jedis.Jedis;
public class Main {
public static void main(String[] args) {
Jedis jedis = new Jedis("localhost");
// Set some keys
jedis.set("key1", "value1");
jedis.set("key2", "value2");
// Delete single key
jedis.del("key1");
// Delete multiple keys
jedis.del("key2", "key3");
}
}
```
In this example, we first create a Jedis object connected to Redis running on localhost. Then we set values for key1
and key2
. The jedis.del()
function is used to delete keys from Redis. You can pass one or more key names to this function.
Best Practices
Use the wildcard search cautiously when deleting keys, as it may affect performance on large databases. Also, always ensure that the keys you are trying to delete exist to avoid unnecessary operations.
Common Mistakes
A common mistake is not handling exceptions while interacting with Redis. It's crucial to handle exceptions such as connection issues or operations on non-existing keys appropriately.
FAQs
Q: Can I delete keys based on a pattern in Java?
A: Yes, you can delete keys based on a pattern by using the keys
method to retrieve keys matching a pattern and then deleting them. However, use this with caution as the keys
command can be heavy on the server. It's recommended to use scan
operation for large databases.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
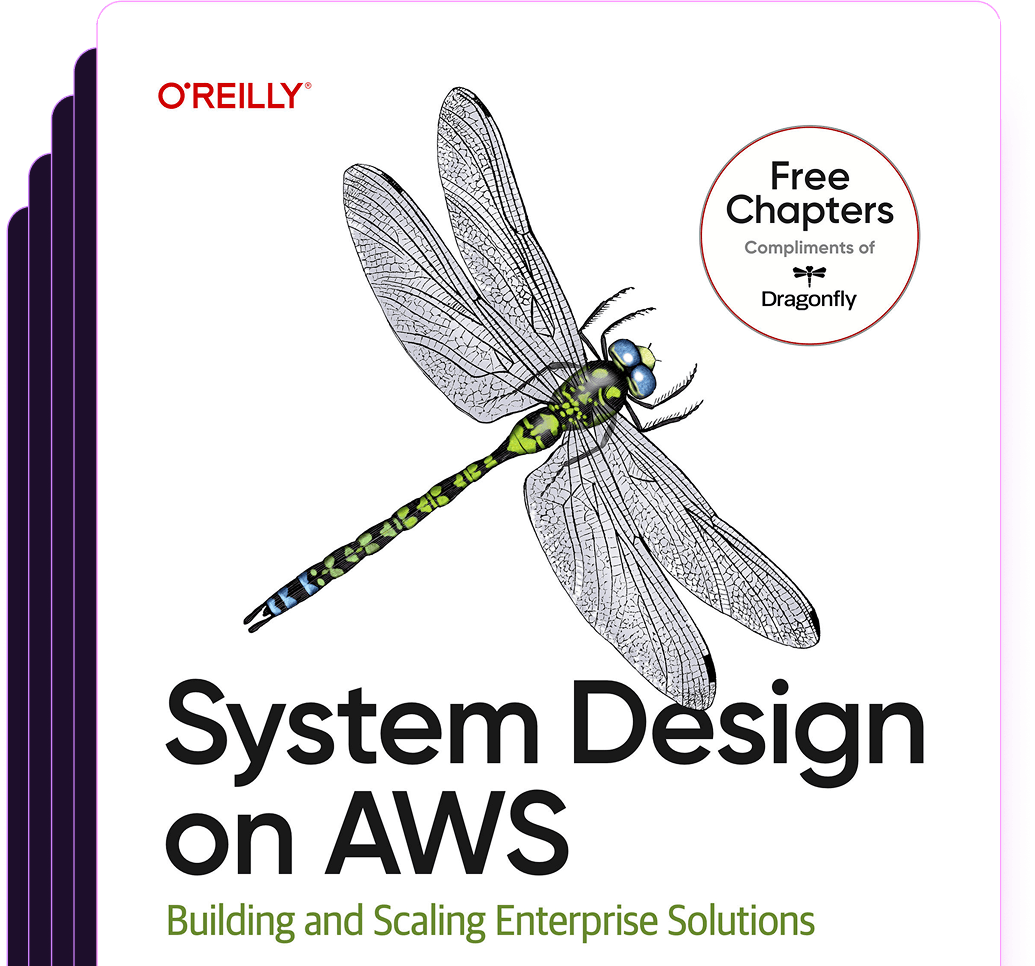
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost