Redis XADD in Java (Detailed Guide w/ Code Examples)
Use Case(s)
Java Redis XADD command is commonly used to append a new entry into a stream. It's especially useful when you're dealing with real-time data processing tasks where data order and time-based sorting are crucial, such as:
- Real-time analytics
- Logging system
- Chat applications
- Time-series data
Code Examples
The Java client for Redis is Jedis. Here's an example of using XADD in Java with the Jedis library.
import redis.clients.jedis.Jedis;
import redis.clients.jedis.StreamEntryID;
public class Main {
public static void main(String[] args) {
// Connect to Redis server
Jedis jedis = new Jedis("localhost");
// Create a HashMap to store field-value pairs
Map<String, String> fields = new HashMap<>();
fields.put("temperature", "25");
fields.put("humidity", "50");
// Add data to Redis stream
StreamEntryID entryID = jedis.xadd("weatherData", null, fields);
System.out.println("Entry added with ID: " + entryID);
// Close the connection
jedis.close();
}
}
In this example, we create a Redis connection using Jedis, then we define a HashMap to hold our field-value pairs - in this case, temperature
and humidity
. We then use the xadd
method to add these fields to the weatherData
stream.
Best Practices
- Always close the Jedis instance after usage to free up resources.
- Use meaningful names for your streams to not confuse them with other data structures.
- Try to limit the size of entries in your streams, large entries might affect the performance.
Common Mistakes
- Not handling exceptions: Jedis might throw an exception if Redis server isn't available or some other network error occurs. Always use try-catch blocks to handle such situations.
- Confusing stream IDs with normal keys: Stream IDs are unique and hold their own data, they can't be used interchangeably with keys.
FAQs
1. When should I use XADD?
XADD is useful when you have real-time data that needs to be processed in order, and you want to store it in a time-series format.
2. What happens if I call XADD on a non-existent stream?
Redis will create a new stream if it doesn't exist already.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
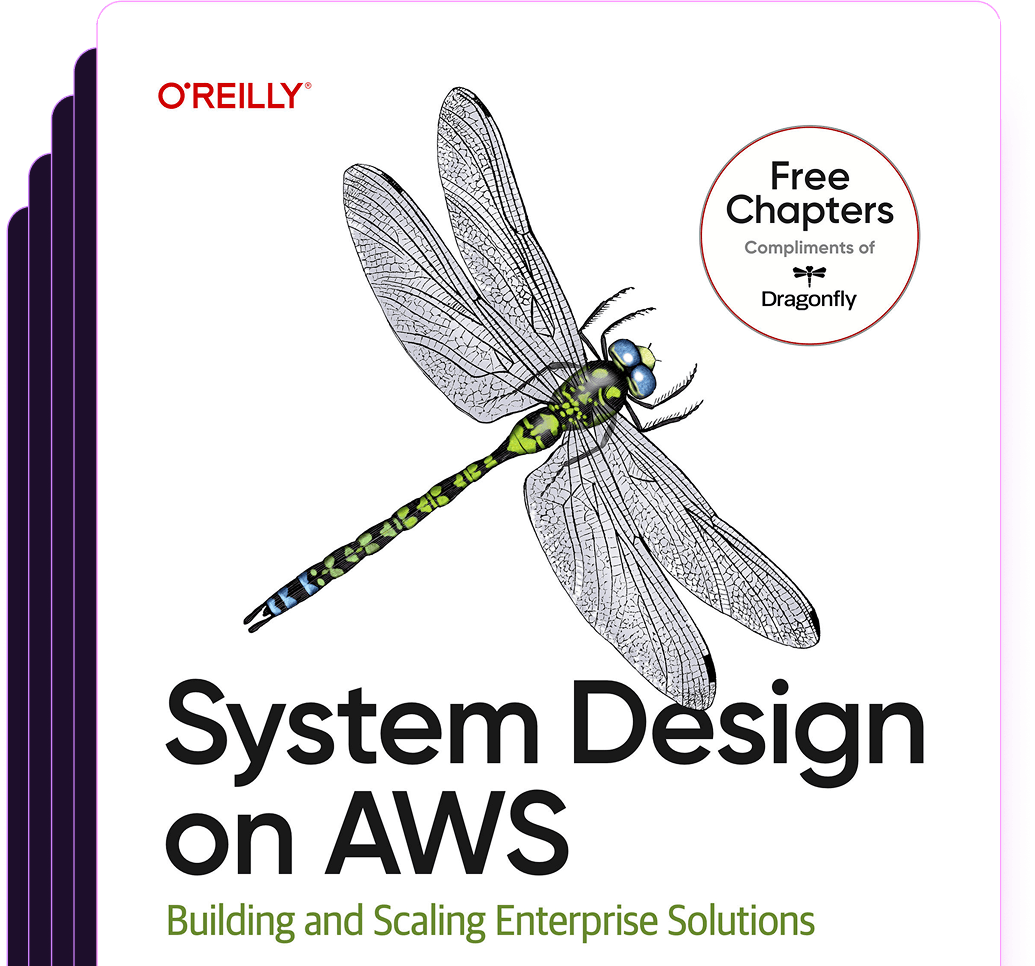
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost