PHP Memcached Set (Detailed Guide w/ Code Examples)
Use Case(s)
The Memcached::set()
function in PHP is used when there's a need to store an item on the memcached server. This could be for caching results of a database query, storing session data, or temporary storage of frequently accessed data.
Code Examples
Example 1 - Basic usage:
<?php
$mem = new Memcached();
$mem->addServer("localhost", 11211);
$key = "my_key";
$value = "Hello, World!";
$expiration = 3600; // Expire after 1 hour
$result = $mem->set($key, $value, $expiration);
if ($result) {
echo "Data has been stored successfully!";
} else {
echo "Failed to store data!";
}
?>
In this example, we first create a new Memcached object and add a server to it. We then define a key-value pair that we want to store in the cache. The expiration parameter specifies the duration (in seconds) the item should remain in the cache. If the set()
method returns true, it means our data has been successfully stored; otherwise, it failed.
Example 2 - Storing an array:
<?php
$mem = new Memcached();
$mem->addServer("localhost", 11211);
$data = array(
"name" => "John Doe",
"email" => "john@example.com"
);
$mem->set('user_data', $data, 600);
?>
In this example, instead of storing a simple string, we're storing an associative array with multiple pieces of information about a user.
Best Practices
- Always check if the
set()
operation was successful. Memcached server may not always be available or might run out of memory, causing the set operation to fail. - Use meaningful and unique keys. This helps to easily identify the data and prevent overwriting of data.
- Be mindful of the expiration times. An excessively long duration might lead to unnecessary usage of memory, while very short durations might defeat the purpose of caching by requiring frequent updates.
Common Mistakes
- Not handling failures: The
set()
method may fail for various reasons such as network issues, Memcached crashing, etc. Always handle these potential failures in your code. - Misunderstanding expiration time: It's easy to confuse the expiration time with a Unix timestamp. Remember, if you give a value less than 30 days (in seconds), it will be treated as relative time. Anything above that is treated as an absolute Unix timestamp.
FAQs
Q: What kind of data can I store using PHP's Memcached set? A: You can store all types of data including strings, numbers, boolean values, objects, and arrays.
Q: Can I overwrite an existing key using the set method? A: Yes, if you use the same key with the set()
function, the previous value will be overwritten.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
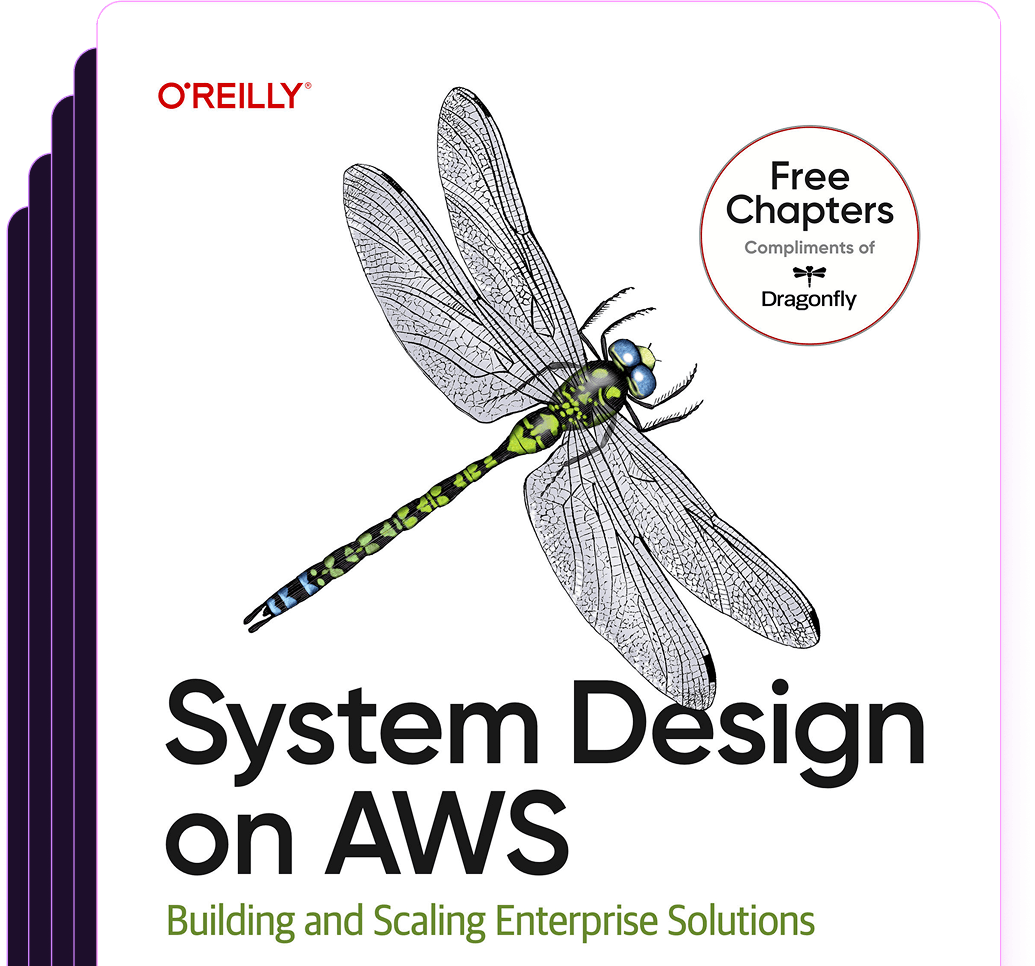
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost