Redis HDEL in PHP (Detailed Guide w/ Code Examples)
Use Case(s)
The HDEL
command in Redis is used when you need to delete one or more fields from a hash stored at a key. It's often used in situations where you have structured data represented as a Hash in Redis and you want to remove specific fields from the Hash.
Code Examples
Here is an example of using the HDEL
command in PHP:
<?php
$redis = new Redis();
$redis->connect('127.0.0.1', 6379);
$redis->hSet('user:1', 'name', 'John Doe');
$redis->hSet('user:1', 'email', 'john.doe@example.com');
// Delete the 'email' field from the hash 'user:1'
$redis->hDel('user:1', 'email');
?>
In this code, we first connect to the Redis server and then set two fields ('name' and 'email') in the hash identified by the key 'user:1'. We then use the hDel
method to delete the 'email' field from the hash.
If you need to delete multiple fields at once, you can pass an array of field names:
<?php
$redis = new Redis();
$redis->connect('127.0.0.1', 6379);
$redis->hMSet('user:1', [
'name' => 'John Doe',
'email' => 'john.doe@example.com',
'phone' => '123-456-7890'
]);
// Delete the 'email' and 'phone' fields from the hash 'user:1'
$redis->hDel('user:1', ['email', 'phone']);
?>
This time, we are setting three fields in the hash and then deleting two of them using the hDel
method with an array argument.
Best Practices
- Be careful when using HDEL as it permanently removes the field from the hash. There is no way to undo this action.
- Use hashes whenever possible to group related data as they are very memory efficient in Redis.
Common Mistakes
- One common mistake is trying to use
HDEL
command on a key that holds a non-hash value. This will result in an error.
FAQs
Q: What happens if I use HDEL
on a field which does not exist in the hash?
A: Redis will consider it a successful operation and return 0 indicating that no fields were removed.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
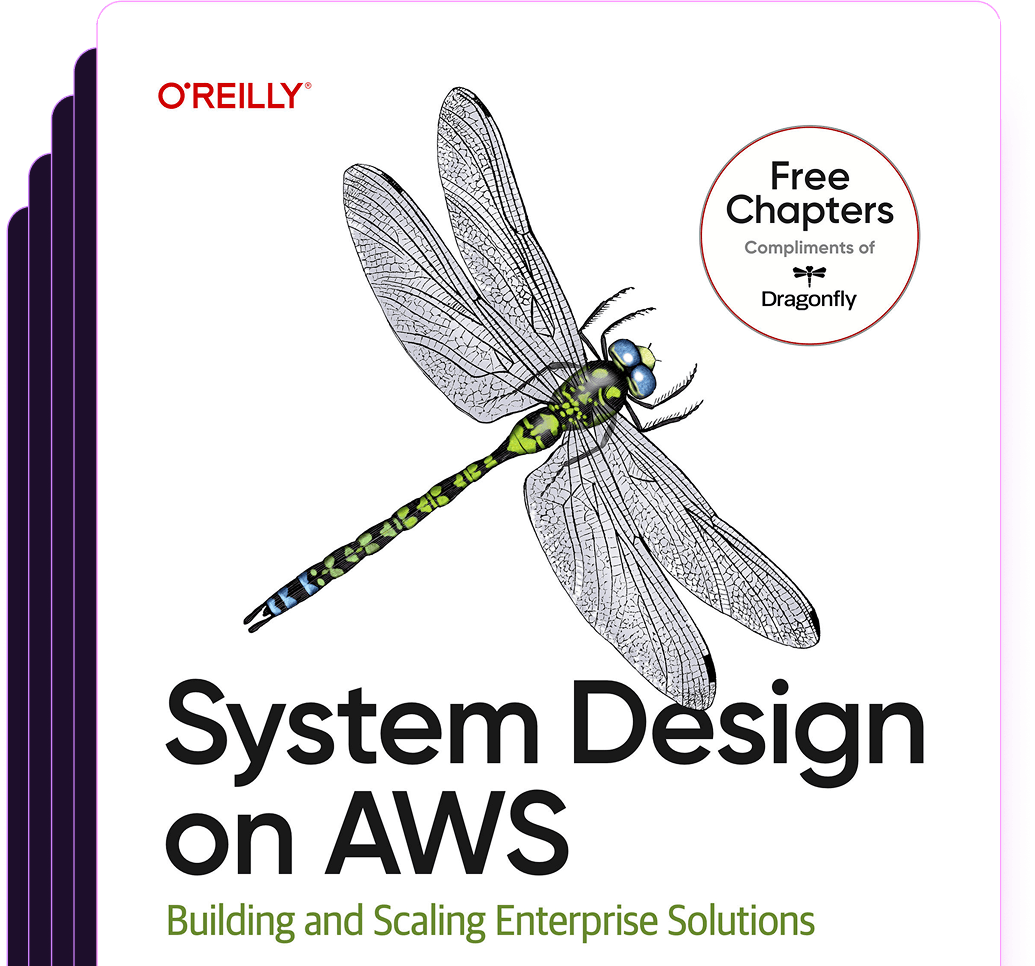
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost