Redis Update Cache in Python (Detailed Guide w/ Code Examples)
Use Case(s)
- Refreshing frequently accessed data: Update cache with new data to ensure users get updated information without hitting the database.
- Session management: Update session information in the cache for user activity tracking and timeout management.
- Configuration settings: Dynamically update configuration settings stored in the cache for application components.
Code Examples
Example 1: Updating a Cached User Profile
import redis
# Connect to Redis
client = redis.StrictRedis(host='localhost', port=6379, db=0)
# Function to update cached user profile
def update_user_profile(user_id, new_profile_data):
key = f"user:{user_id}"
# Update the user's cached profile
client.hmset(key, new_profile_data)
print(f"User profile for {user_id} updated.")
# Example usage
new_profile = {
'name': 'Jane Doe',
'email': 'jane.doe@example.com',
'age': 30
}
update_user_profile('12345', new_profile)
Explanation: This example shows how to update a user's profile data in the Redis cache using the hmset
command. The profile is stored as a hash.
Example 2: Updating Cached Configuration Settings
import redis
import json
# Connect to Redis
client = redis.StrictRedis(host='localhost', port=6379, db=0)
# Function to update configuration settings in cache
def update_config(config_key, new_settings):
config_value = json.dumps(new_settings)
# Set the new configuration in cache
client.set(config_key, config_value)
print(f"Config for {config_key} updated.")
# Example usage
new_settings = {
'feature_flag': True,
'max_connections': 100,
'timeout': 300
}
update_config('app:config', new_settings)
Explanation: This example updates a JSON-encoded configuration setting in the cache using the set
command. The configuration is serialized to a JSON string before being stored.
Best Practices
- Set expiration times: Use TTL (time-to-live) to ensure outdated data is eventually removed from the cache.
- Atomic operations: Use pipelines or transactions where multiple related commands need to be executed atomically to avoid inconsistent states.
- Monitor cache size: Regularly monitor your cache size and eviction policy to prevent memory overflow issues.
Common Mistakes
- Ignoring exception handling: Always handle exceptions that may arise from network issues or server unavailability to make your application robust.
- Overusing the cache: Storing too much data in the cache can lead to performance degradation. Ensure only frequently accessed and important data is cached.
- Not synchronizing cache and database: Ensure mechanisms are in place to keep the cache and database synchronized to prevent stale data issues.
FAQs
Q: How do I know if my cache update was successful? A: You can use the get
or hgetall
commands to retrieve the value after updating it and verify its correctness.
Q: Can I batch update multiple keys in Redis? A: Yes, you can use pipelines to batch update multiple keys efficiently.
Q: Is there a limit to the size of data I can cache in Redis? A: While Redis can handle large datasets, it's essential to monitor memory usage and configure appropriate eviction policies to manage memory effectively.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
- Redis Concurrent Update in Python
- Redis Update Value in List in Python
- Redis Update Value Without Changing TTL in Python
- Redis JSON Update in Python
- Redis Bulk Update in Python
- Redis Update TTL in Python
- Redis Update Value in Python
- Redis Update TTL on Read in Python
- Redis Atomic Update in Python
- Redis Conditional Update in Python
- Redis Partial Update in Python
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
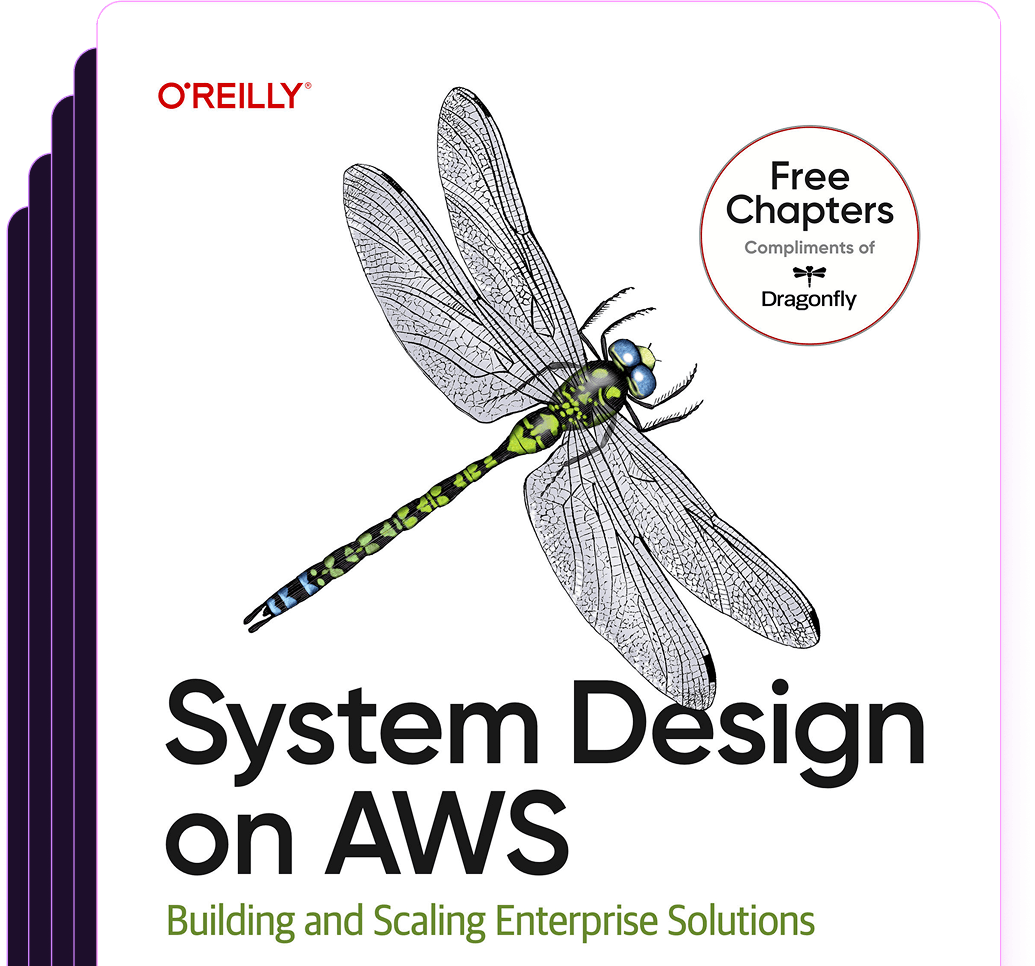
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost