Redis HSET in Ruby (Detailed Guide w/ Code Examples)
Use Case(s)
The HSET
command in Redis is used to set the field in the hash stored at key to value. If the key does not exist, a new key holding a hash is created. In Ruby, this is commonly used for storing complex objects or multiple related data points.
Code Examples
Here's an example of using HSET in Ruby with the redis
gem:
require 'redis'
# Create a new Redis connection
redis = Redis.new
# Set the hash field
redis.hset('user:1001', 'name', 'Alice')
redis.hset('user:1001', 'email', 'alice@example.com')
# The user:1001 hash now has two fields - name and email.
In this example, we're creating a new Redis connection, then using the hset
method to set two fields ('name' and 'email') for the hash associated with the key 'user:1001'.
It's also possible to set multiple fields at once:
redis.hmset('user:1002', 'name', 'Bob', 'email', 'bob@example.com')
In this example, we're using the hmset
method to set both 'name' and 'email' fields of the 'user:1002' hash simultaneously.
Best Practices
- It's a good idea to handle exceptions that might occur during Redis operations. This could be done by wrapping your code in a begin-rescue block.
- When naming keys, follow a convention that includes the object type and ID for easy identification (e.g., 'user:1001').
Common Mistakes
- Not checking if the operation was successful. In Ruby, the
hset
function returns true if the field is a new field in the hash and value has been set. If the field already exists in the hash and the value did not get updated (because it's the same as the old value), it returns false.
FAQs
- What happens if the key does not exist? If the given key doesn't exist, Redis will create a new key holding a hash with the provided field and value.
- Can HSET be used on non-hash keys? No, if the key exists and does not hold a hash value, an error is returned.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
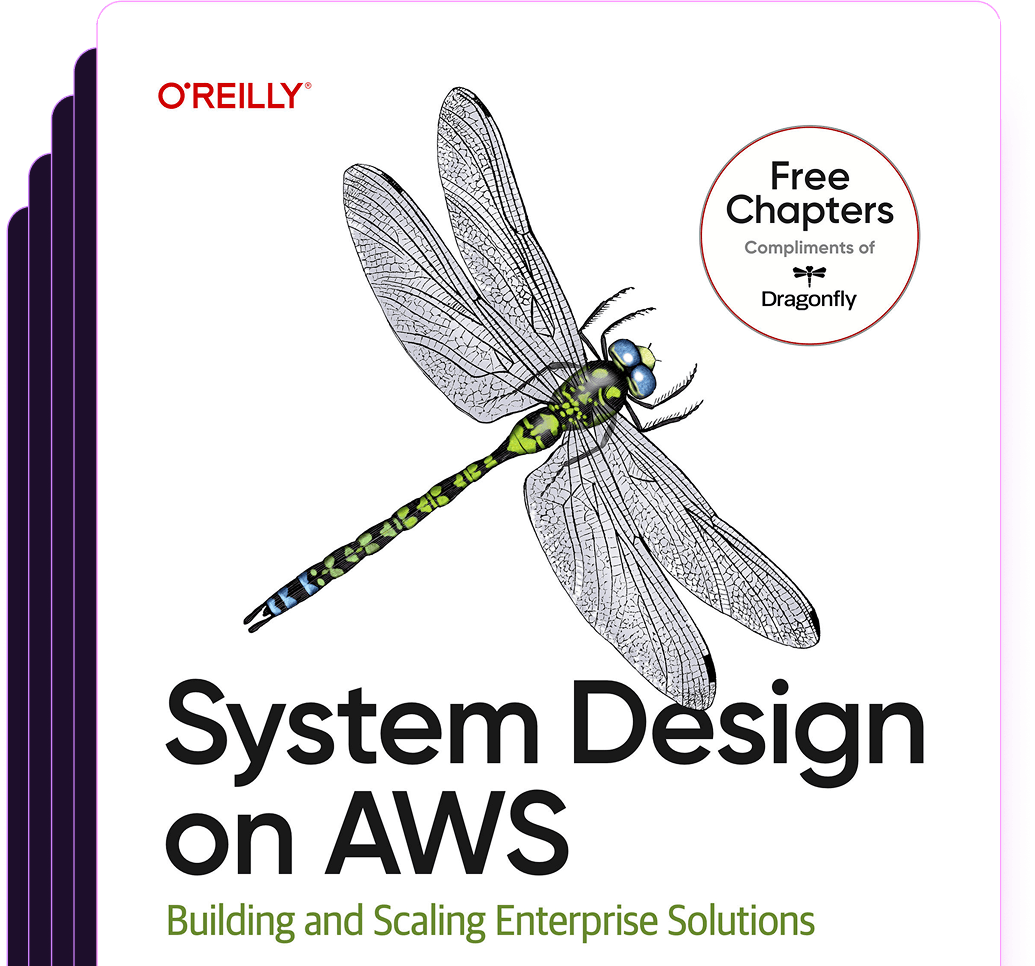
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost