Error: redis.exceptions.responseerror moved
Solution
What's Causing This Error
The error message you encountered, "redis.exceptions.ResponseError: MOVED," indicates that the Redis server has informed the client that the requested key has been moved to a different Redis node. This error commonly occurs in Redis cluster setups where data is distributed across multiple nodes.
When a Redis cluster is configured to use slot-based sharding, keys are mapped to specific slots, and each slot is assigned to a particular Redis node. If a client attempts to access a key that belongs to a different slot and is located on a different node, Redis will respond with a MOVED error and provide the client with the correct node where the key resides.
## Solution - Here's How To Resolve It
To handle this error, you need to update your client code to handle key redirection properly. When you receive a MOVED error, you should extract the new node information from the error response and redirect your Redis client to that node for subsequent operations on that key.
Here's an example of how you can handle the MOVED error in Python using the redis-py library:
```python
import redis
Create a Redis client
redis_client = redis.Redis(host='redis_host', port=6379)
Perform a Redis operation that triggers the MOVED error
try:
result = redis_client.get('your_key')
except redis.exceptions.ResponseError as e:
if e.args[0].startswith('MOVED'):
# Extract the new node information from the error response
moved_info = e.args[0].split(' ')
new_host, new_port = moved_info[2].split(':')
# Update the Redis client connection to the new node
redis_client = redis.Redis(host=new_host, port=int(new_port))
# Retry the operation on the new node
result = redis_client.get('your_key')
else:
# Handle other types of Redis errors
raise
```
By handling the MOVED error and updating the Redis client's connection to the correct node, you can ensure that your client code can properly access the Redis keys in a cluster setup.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common Redis Errors (with Solutions)
- could not connect to redis at 127.0.0.1:6379: connection refused
- redis error server closed the connection
- redis.exceptions.responseerror: value is not an integer or out of range
- redis.exceptions.responseerror noauth authentication required
- redis-server failed to start advanced key-value store
- spring boot redis unable to connect to localhost 6379
- unable to configure redis to keyspace notifications
- redis.clients.jedis.exceptions.jedismoveddataexception
- could not get resource from pool redis
- failed to restart redis service unit redis service not found
- job for redis-server.service failed because a timeout was exceeded
- failed to start redis-server.service unit redis-server.service is masked
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
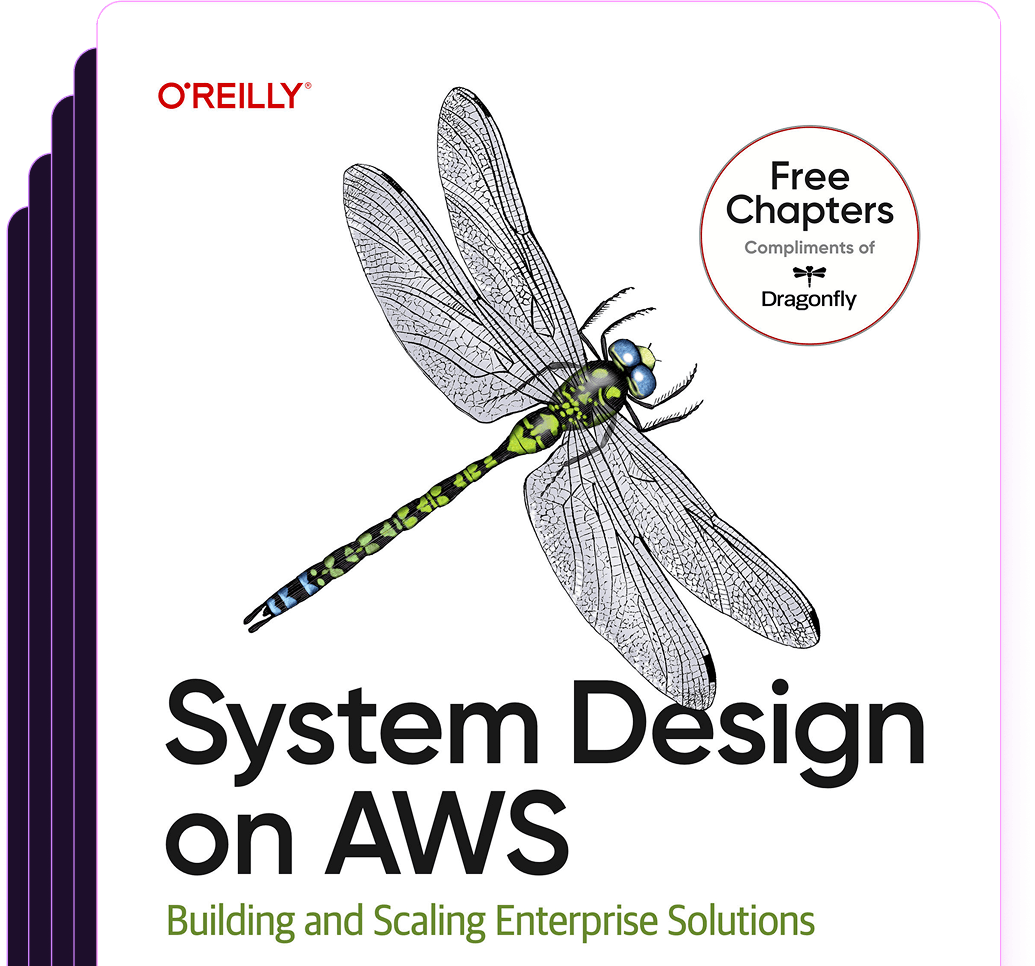
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost