Question: What is the performance difference between a database and JSON?
Answer
It's important to understand that databases and JSON (JavaScript Object Notation) are not directly comparable, as they serve different purposes. Databases are designed for storing, managing, and retrieving structured data efficiently, while JSON is a lightweight data-interchange format that is easy for humans to read and write, and for machines to parse and generate.
However, in terms of performance, here's a comparative analysis:
- Storage Efficiency: Databases, especially relational ones like MySQL or PostgreSQL, organize data in tables, allowing efficient storage for large sets of structured data. On the other hand, JSON stores data as text, which can be less space-efficient, especially when dealing with large amounts of data.
- Read/Write Speeds: Databases are generally faster at reading and writing large volumes of data because they use specialized algorithms and data structures optimized for these tasks. JSON files would need to be read entirely into memory before accessing data, which becomes inefficient and slow with larger data sets.
- Querying Capabilities: Databases support complex queries, including sorting, filtering, joining, and aggregating data. This allows for fast access to subsets of data based on specific criteria. With JSON, you would typically need to load all data into memory and process it using general-purpose programming languages, such as JavaScript, which can be slower and consume more resources.
- Concurrency and Transactions: Databases offer built-in support for concurrent transactions and data consistency mechanisms, which are crucial for multi-user applications. JSON does not inherently support transactions or concurrency.
- Scalability: Databases are generally more scalable, offering features like indexing, partitioning, and replication to handle larger data sizes and higher loads. JSON files might not scale well for large datasets because they need to be read wholly into memory.
However, it should be noted that JSON is often used in conjunction with databases. For example, many NoSQL databases like MongoDB use a form of JSON (BSON) for storing and querying data. Also, JSON is commonly used for transferring data over the network in web APIs due to its lightweight nature and easy integration with JavaScript.
# Example: Querying data from PostgreSQL and having results in JSON format.
import psycopg2
import json
try:
conn = psycopg2.connect(database='testdb', user='postgres', password='secret', host='127.0.0.1', port='5432')
print("Opened database successfully")
except:
print("Cannot connect to the database")
cur = conn.cursor()
cur.execute("SELECT * FROM COMPANY;")
rows = cur.fetchall()
# Convert query results to JSON
json_rows = [dict(row) for row in rows]
print(json.dumps(json_rows, indent=4))
conn.close()
In this Python code snippet, we connect to a PostgreSQL database, execute a SELECT query, fetch all rows and then convert them to JSON format using Python's json module.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common Database Performance Questions (and Answers)
- What is the difference between database latency and throughput?
- What is database read latency and how can it be reduced?
- How can you calculate p99 latency?
- How can one check database latency?
- What causes latency in database replication and how can it be minimized?
- How can you reduce database write latency?
- How can you calculate the P90 latency?
- How can you calculate the p95 latency in database performance monitoring?
- How can you calculate the p50 latency?
- What is database latency?
- What are the causes and solutions for latency in database transactions?
- What is the difference between p50 and p95 latency in database performance metrics?
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
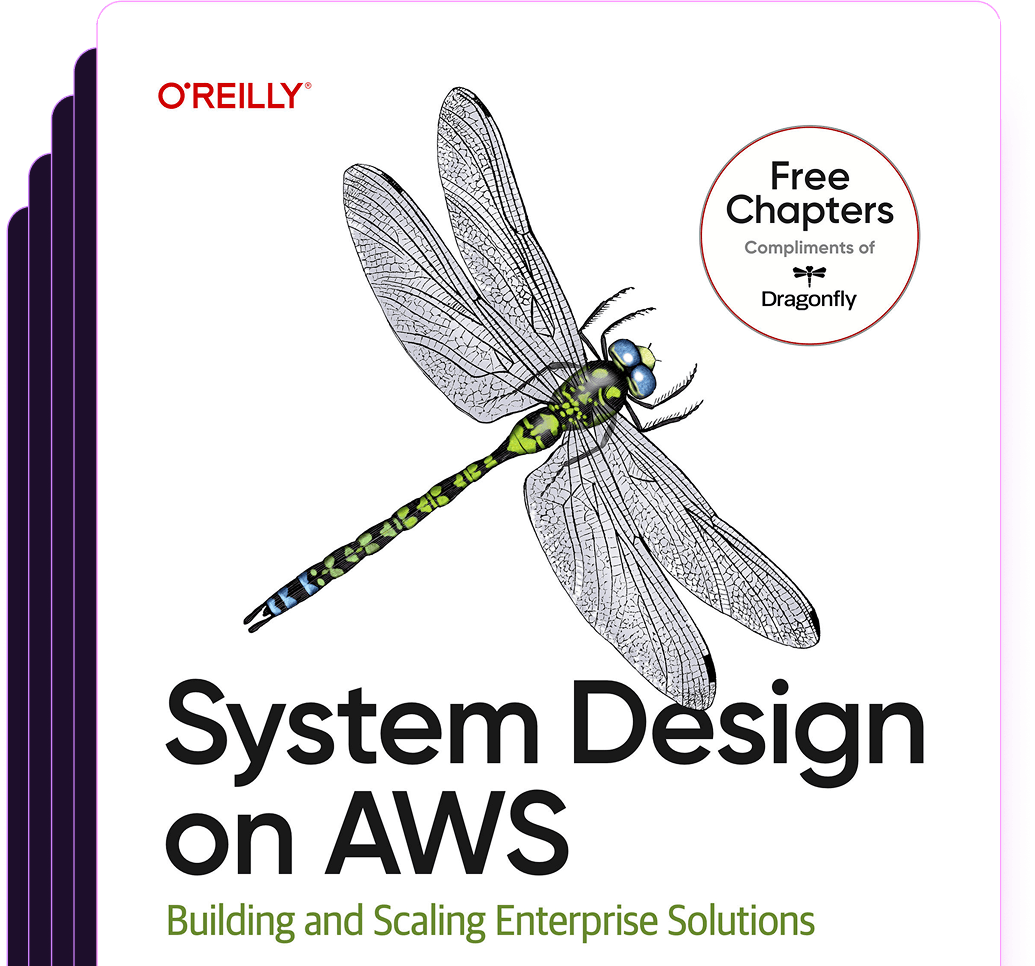
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost