Question: Do you need a game engine to make a game?
Answer
No, you don't strictly need a game engine to make a game. However, using a game engine can greatly simplify the process and allow you to focus more on the gameplay, art, and story.
Without a game engine, you would need to write all of the code yourself from scratch for everything including rendering, physics, audio, and more. This is not only a lot of work, but also requires a broad range of expertise in various complex fields such as 3D graphics and physics simulation.
If you decide to develop a game without an engine, here's a simple example of how you might create a window and render a basic 2D shape using OpenGL in C++:
#include <GL/glut.h>
void display() {
glClear(GL_COLOR_BUFFER_BIT);
glBegin(GL_TRIANGLES);
glVertex2f(-0.5f, -0.5f);
glVertex2f( 0.5f, -0.5f);
glVertex2f( 0.0f, 0.5f);
glEnd();
glFlush();
}
int main(int argc, char** argv) {
glutInit(&argc, argv);
glutCreateWindow("OpenGL Setup Test");
glutDisplayFunc(display);
glutMainLoop();
return 0;
}
On the other hand, if you use a game engine like Unity or Unreal, many of these features are provided out of the box. For example, in Unity, creating a sprite on the screen could be as simple as dragging and dropping an image into your scene in the editor.
The choice between using a game engine or not depends largely on your game's requirements, your team's skill set, and the resources you have available. However, for most game developers—particularly those just starting out—a game engine is an invaluable tool that can save a lot of time and effort.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common Game Dev Questions (and Answers)
- Do Game Engines Cost Money?
- Can I Use an SQL Database for Game Development?
- How are databases used in game development?
- How do you save multiplayer game data, in a database or a file?
- How can you design an efficient database for a game?
- Should I Use Redis or MySQL for Game Development?
- What are the differences between using a database and JSON for games?
- Do Video Games Use Databases?
- Does game development require knowledge of mathematics?
- Should I Use a Game Engine or Not?
- Is a game engine considered a framework?
- How can you design a game leaderboard system?
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
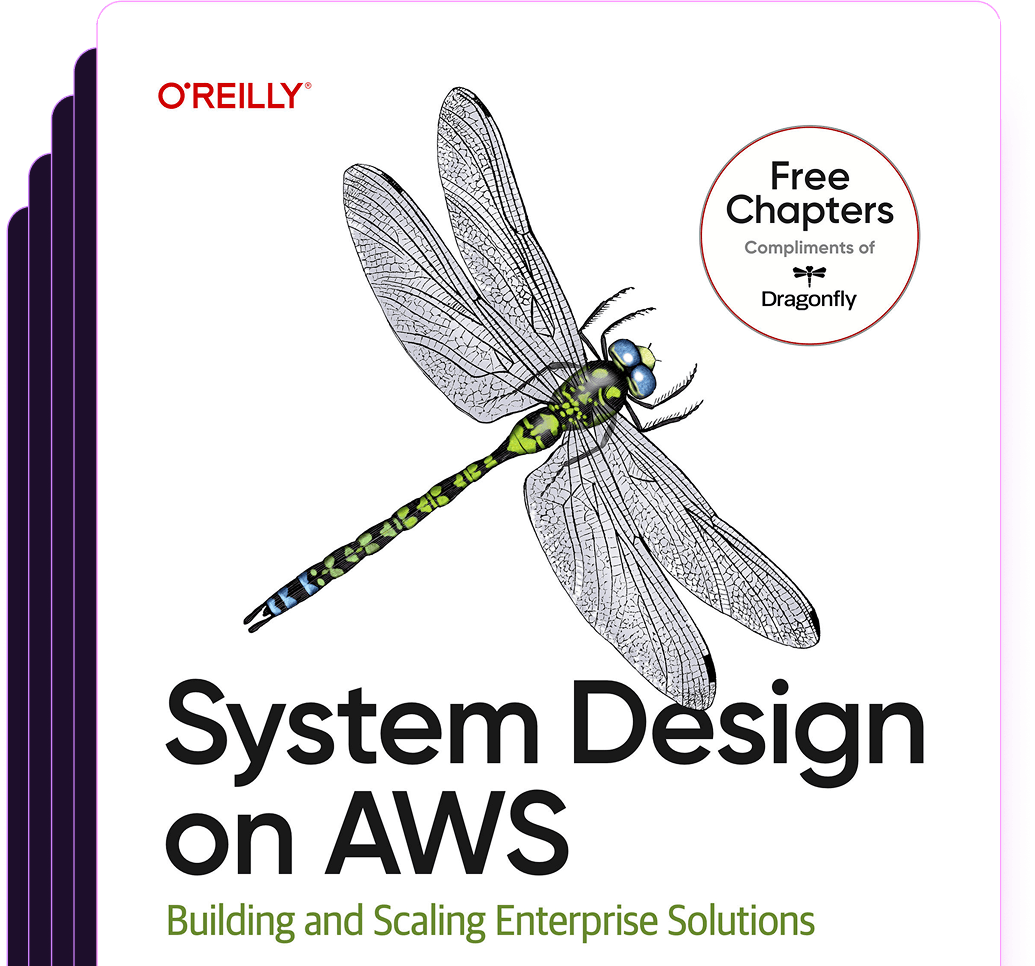
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost