Question: How can I connect to a MongoDB cluster with a connection string?
Answer
Connecting to a MongoDB cluster involves using a MongoDB connection string, which specifies the necessary information for connecting to the database. The general format of a MongoDB connection string is as follows:
```
mongodb+srv://<username>:<password>@<cluster-address>/test?retryWrites=true&w=majority
```
Steps to Connect to MongoDB Cluster
- Get Your Connection String: Log in to your MongoDB Atlas dashboard (or your MongoDB hosting provider) where you've set up your cluster. Navigate to the 'Connect' section of your cluster and choose 'Connect your application'. Here, you will be provided with the standard connection string.
- Replace Placeholder Values: You'll need to replace
<username>
,<password>
, and<cluster-address>
with your actual database username, password, and cluster address. Sometimes, you might also need to replace/test
with your specific database name if you're not connecting to a database named 'test'. - Use the Connection String in Your Application: In most applications, you'll use a MongoDB client library to connect to your database. Here's an example using the popular Node.js MongoDB driver:
const { MongoClient } = require('mongodb');
// Replace the following with your Atlas connection string
const uri = 'mongodb+srv://<username>:<password>@<cluster-address>/myFirstDatabase?retryWrites=true&w=majority';
const client = new MongoClient(uri, { useNewUrlParser: true, useUnifiedTopology: true });
async function run() {
try {
await client.connect();
console.log('Connected successfully to server');
// Proceed with operations (e.g., CRUD operations)
} finally {
await client.close();
}
}
run().catch(console.dir);
Replace mongodb+srv://<username>:<password>@<cluster-address>/myFirstDatabase?retryWrites=true&w=majority
with your actual connection string.
Important Considerations
- Ensure that your IP address is whitelisted in your MongoDB cluster's network access settings to allow connections from your application.
- Always store your connection strings securely, especially since they contain sensitive information such as your database username and password. Environment variables or secret management tools are commonly used for this purpose.
- Use the correct driver version compatible with your MongoDB server version for optimal performance and security.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common MongoDB Performance Questions (and Answers)
- How to improve MongoDB query performance?
- How to check MongoDB replication status?
- How do you connect to a MongoDB cluster?
- How do you clear the cache in MongoDB?
- How many connections can MongoDB handle?
- How does MongoDB sharding work?
- How to check MongoDB cluster status?
- How to change a MongoDB cluster password?
- How to create a MongoDB cluster?
- How to restart a MongoDB cluster?
- How do I reset my MongoDB cluster password?
- How does the $in operator affect performance in MongoDB?
White Paper
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
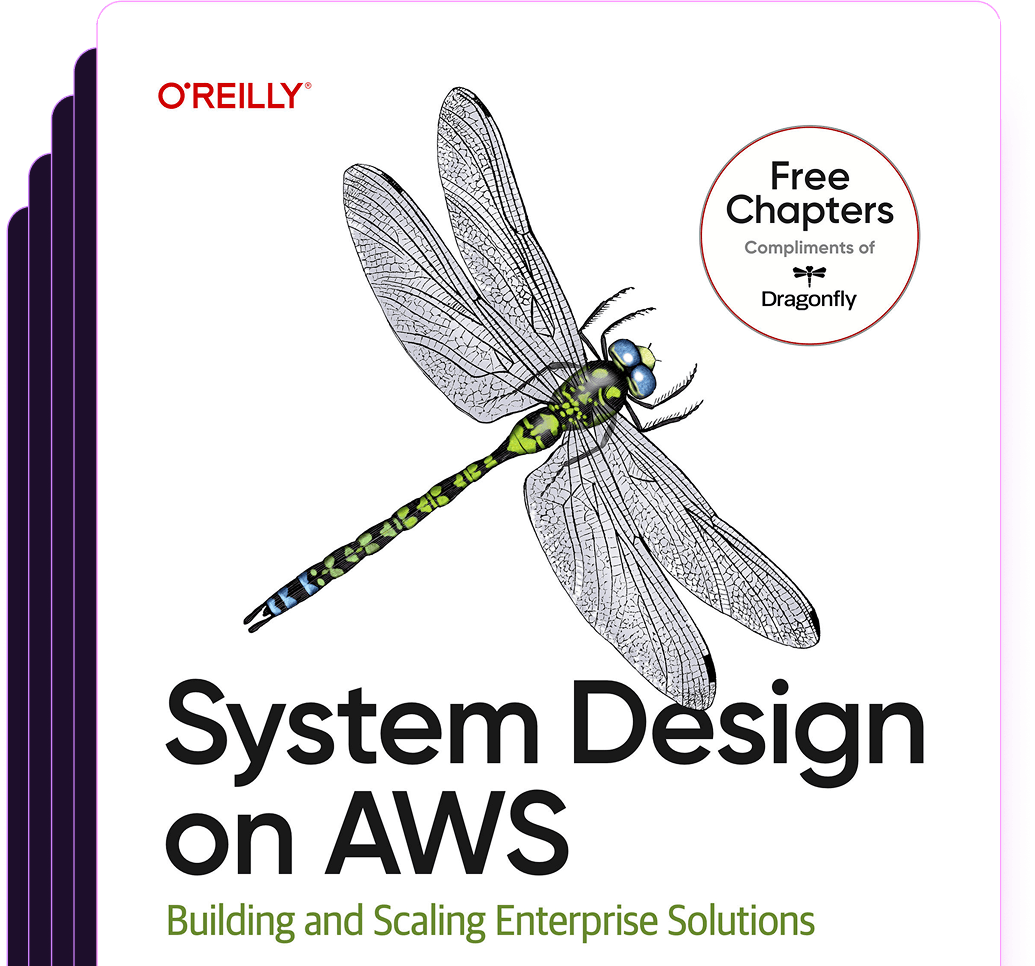
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost