Question: What is the difference between clearing data and clearing cache in a database?
Answer
In databases, the concepts of "clearing data" and "clearing cache" serve different purposes and have distinct impacts on performance and persistence. Understanding these differences is essential for effective database management.
Clearing Data
Clearing data refers to removing records (rows) from one or more tables within the database. This operation directly affects the stored data itself, leading to permanent data loss unless backups exist. It's typically performed to free up space, remove outdated information, or prepare the database for fresh data.
Example:
In SQL, you might clear data using the DELETE
statement:
DELETE FROM users WHERE last_login < '2021-01-01';
This command deletes records of users who haven't logged in since before January 1, 2021.
What You Lose When Clearing Data:
- Permanent Record Deletion: The actual data entries are permanently removed from the database.
- Data Insights: Historical data, analytics, and user behavior insights can be lost.
- Storage Space: Frees up storage space by removing unnecessary or old data.
Clearing Cache
Database caching mechanisms store temporary data, such as query results, to improve performance by reducing the need to access the slower disk storage for frequently requested data. Clearing the cache does not affect the actual data in the database but might slow down subsequent queries until the cache is repopulated.
Databases like PostgreSQL and MySQL have their own caching mechanisms (e.g., the query cache in MySQL), and external caching solutions like Redis are also widely used.
Example:
For MySQL, you can clear the query cache with:
RESET QUERY CACHE;
Note: The effectiveness and availability of this feature depend on the MySQL version and configuration, as query cache has been deprecated in recent versions.
What You Lose When Clearing Cache:
- Temporary Performance: A temporary drop in performance can occur as the cache needs to be rebuilt.
- Speed: Faster access to frequently queried data is temporarily lost.
- Efficiency: Initial queries after clearing the cache may take longer as they rebuild the cache.
Key Differences
- Impact on Data: Clearing data removes actual records from tables, resulting in permanent data loss. Clearing cache only affects temporary stored data and doesn't change the persistent database records.
- Performance Considerations: Removing data can permanently reduce the dataset size, potentially improving long-term performance. Clearing cache might lead to temporary performance degradation while the cache is being rebuilt.
- Use Cases: Clearing data is done for data management and storage optimization. Clearing cache is primarily about maintaining or troubleshooting performance issues.
Both operations are crucial for database administrators, each serving its purpose in different scenarios related to maintaining and optimizing database performance and integrity.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common Database Performance Questions (and Answers)
- What is the difference between database latency and throughput?
- What is database read latency and how can it be reduced?
- How can you calculate p99 latency?
- How can one check database latency?
- What causes latency in database replication and how can it be minimized?
- How can you reduce database write latency?
- How can you calculate the P90 latency?
- How can you calculate the p95 latency in database performance monitoring?
- How can you calculate the p50 latency?
- What is database latency?
- What are the causes and solutions for latency in database transactions?
- What is the difference between p50 and p95 latency in database performance metrics?
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
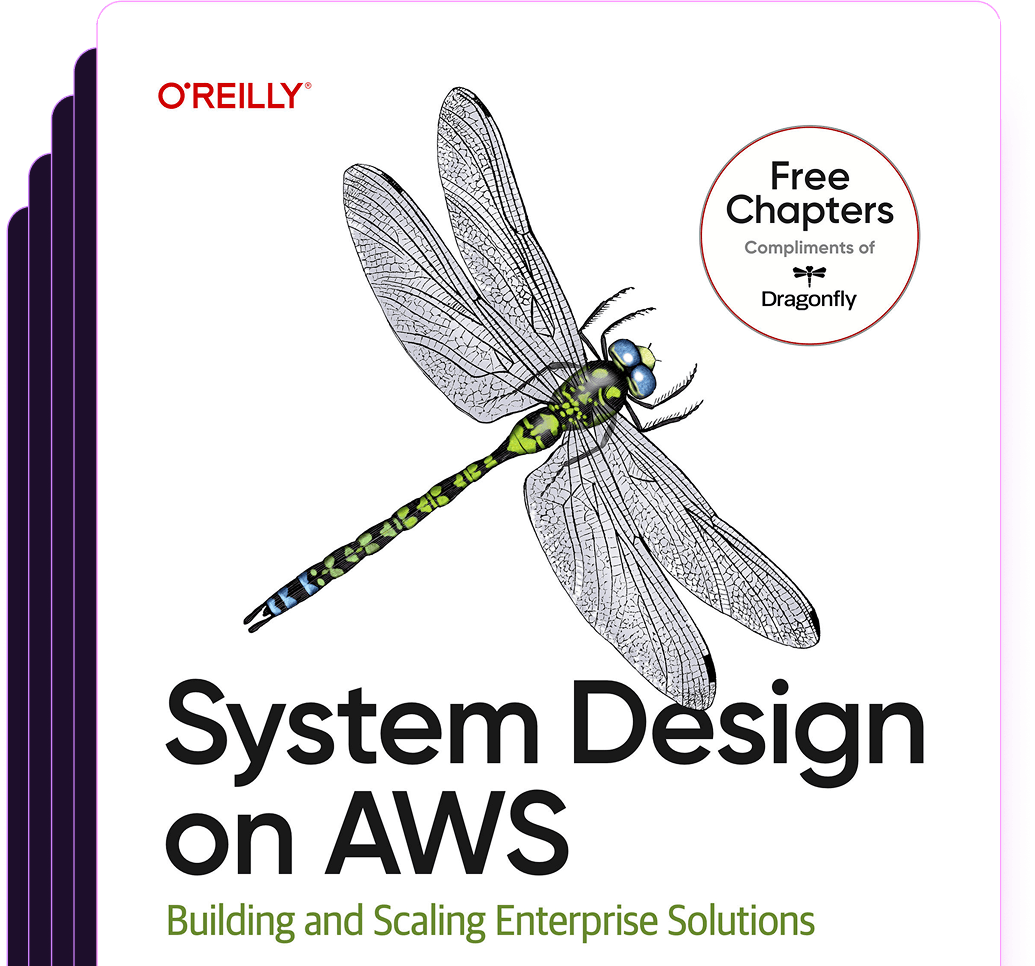
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost