Question: How to connect to cloud database
Answer
Connecting to a cloud database involves several steps that vary depending on the cloud service provider (CSP) and the type of database you're using. Below are general instructions and examples for connecting to a cloud database, specifically focusing on Amazon RDS and Google Cloud SQL.
Amazon RDS
1. Set Up Your Database Instance:
- Log in to the AWS Management Console.
- Navigate to RDS and create a new database instance.
- Configure the instance settings, such as instance class, storage, and database credentials.
- Ensure that the instance is publicly accessible or within your VPC.
2. Configure Security Groups:
- Modify the security group associated with your RDS instance to allow inbound traffic on the database port (default is 3306 for MySQL).
- Add your IP address or application server's IP address to the inbound rules.
3. Connect Using Client Tools:
- Use a tool like MySQL Workbench, pgAdmin, or any other client that supports your database type.
CODE_BLOCK_PLACEHOLDER_0
Google Cloud SQL
1. Set Up Your Database Instance:
- Log in to Google Cloud Console.
- Navigate to SQL and create a new instance.
- Choose the database engine (MySQL, PostgreSQL, SQL Server) and configure instance settings.
2. Authorize Networks:
- In the Connections tab, add the IP addresses that are allowed to connect to this instance (e.g., your home IP, application server IP).
3. Connect Using Client Tools:
- Use tools like MySQL Workbench, pgAdmin, or the Cloud SQL Auth Proxy for secure connections.
from google.cloud.sql.connector import Connector
import sqlalchemy
# Initialize cloud SQL connector
connector = Connector()
def getconn():
conn = connector.connect(
"your-project:your-region:your-instance",
"pymysql",
user="your-username",
password="your-password",
db="your-database"
)
return conn
# Create SQLAlchemy engine
engine = sqlalchemy.create_engine(
"mysql+pymysql://",
creator=getconn,
)
# Execute query
with engine.connect() as connection:
result = connection.execute("SELECT VERSION()")
for row in result:
print(row)
Conclusion
The exact steps might differ based on the database type and CSP, but these examples should give you a good starting point. Always refer to the specific documentation of the service provider for detailed instructions.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common Cloud Databases Questions (and Answers)
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
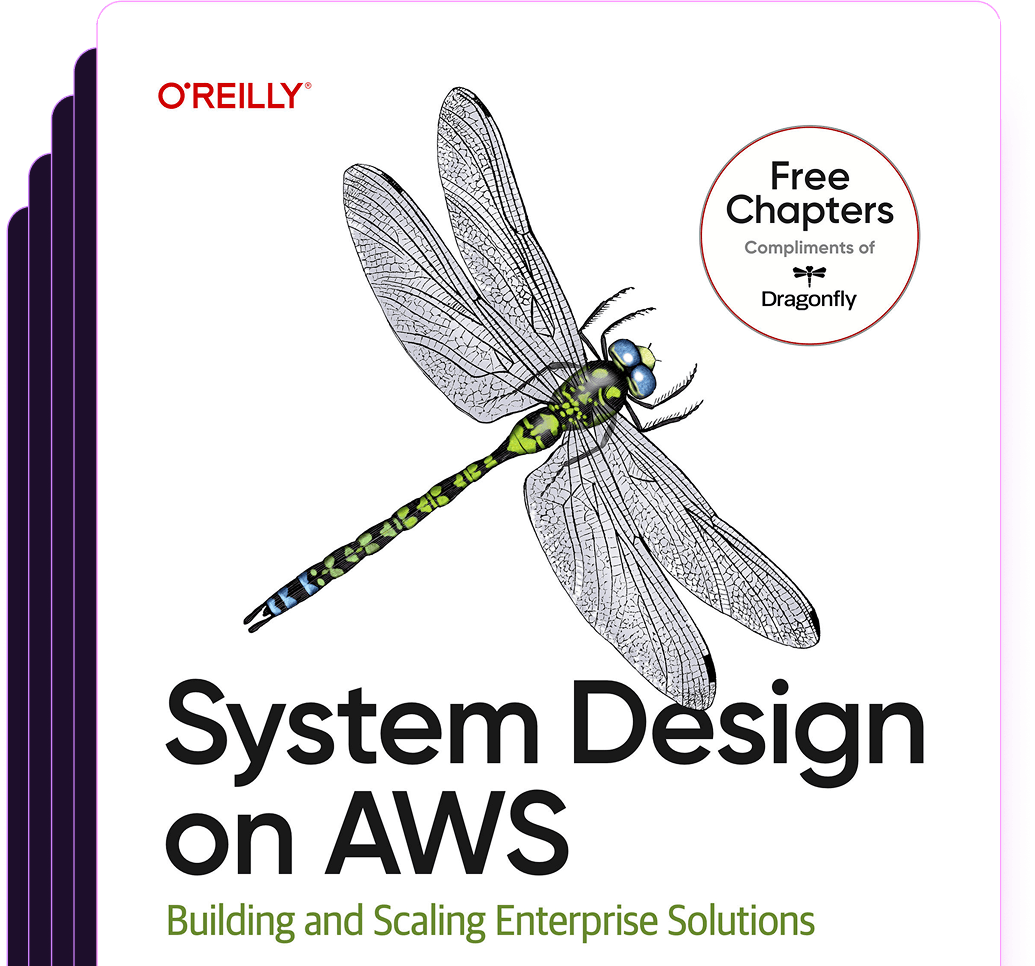
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost