Question: How to update cache when database changes?
Answer
Caching is a critical part of improving the performance and scalability of web applications. It helps in reducing the load on the database by storing frequently accessed data in memory. However, maintaining consistency between the cache and the underlying database is a common challenge. Here's how you can update the cache when the database changes:
Use Cache Invalidation
Cache invalidation involves removing entries from the cache so that subsequent requests for those entries fetch fresh data from the database. There are several strategies for cache invalidation:
1. Write-Through Caching
In this approach, the application writes data to the cache and the database simultaneously. This ensures that the cache always contains the most up-to-date data.
def update_data(key, value):
# Update the database
database.update(key, value)
# Update the cache
cache.set(key, value)
2. Cache-Aside (Lazy Loading)
With cache-aside, the application only updates the database. When data is requested, it first checks the cache. If the data is not found, it fetches it from the database and then stores it in the cache for future use. In this scenario, when an update occurs, you simply invalidate or delete the cache entry.
def update_data(key, value):
# Update the database
database.update(key, value)
# Invalidate the cache entry
cache.delete(key)
def get_data(key):
# Try to get data from the cache
value = cache.get(key)
if value is None:
# Load data from the database
value = database.get(key)
# Store the data in the cache for future access
cache.set(key, value)
return value
Use Event-driven Cache Invalidation
For more complex scenarios, consider using an event-driven approach. This involves setting up a notification system that triggers cache invalidation or updates based on database change events.
Implementing with Redis Pub/Sub:
# On the publisher side (where database updates occur)
def publish_database_update_event(key):
redis.publish('database_updates', key)
# On the subscriber side (cache invalidation logic)
def on_database_update_event(key):
cache.delete(key)
# Setting up the subscriber
def setup_subscriber():
pubsub = redis.pubsub()
pubsub.subscribe(**{'database_updates': on_database_update_event})
pubsub.run_in_thread(sleep_time=0.001)
Conclusion
Updating the cache when the database changes requires careful planning and implementation of invalidation strategies. The choice of strategy depends on your application's specific needs for consistency, complexity, and performance. Write-through caching works well for applications requiring strong consistency, whereas cache-aside may be preferred for read-heavy applications with less stringent consistency requirements. For dynamic or complex environments, an event-driven approach can provide a more flexible solution.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common Database Performance Questions (and Answers)
- What is the difference between database latency and throughput?
- What is database read latency and how can it be reduced?
- How can you calculate p99 latency?
- How can one check database latency?
- What causes latency in database replication and how can it be minimized?
- How can you reduce database write latency?
- How can you calculate the P90 latency?
- How can you calculate the p95 latency in database performance monitoring?
- How can you calculate the p50 latency?
- What is database latency?
- What are the causes and solutions for latency in database transactions?
- What is the difference between p50 and p95 latency in database performance metrics?
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
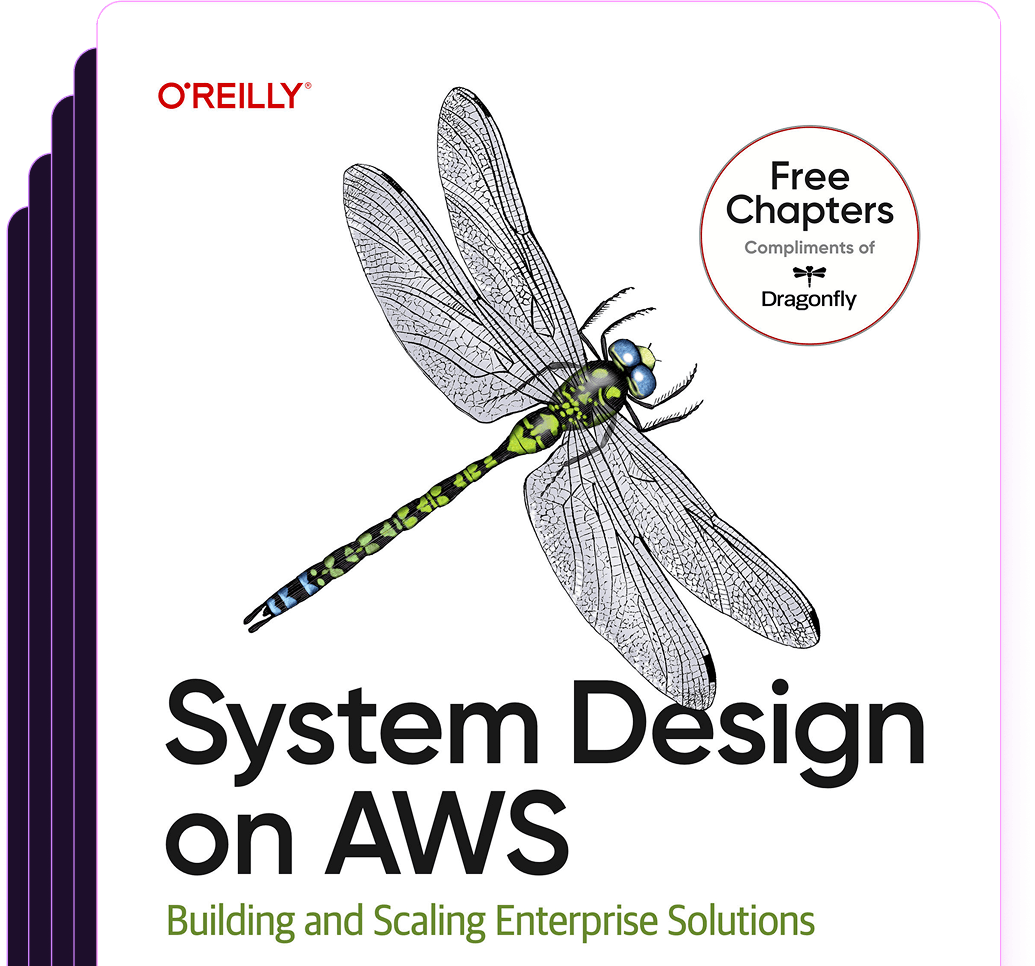
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost