Question: What is the difference between a key-value store and a hashmap?
Answer
Key-value stores and hashmaps are both data structures used to store and manage data, but they serve different purposes and operate in varied contexts. Here's a detailed comparison:
Definition and Use Cases
- Hashmap (or Hashtable): A hashmap is an in-memory data structure that stores data in key-value pairs. The keys are unique, and each key maps to precisely one value. Hashmaps provide fast data retrieval, insertion, and deletion operations. They are widely used in algorithms and programming for tasks like caching, lookups, and maintaining dictionaries.
- Key-Value Store: A key-value store is a type of database designed for storing, retrieving, and managing associative arrays, and more complex data it's typically implemented using hashing under the hood. Key-value stores can be disk-based or in-memory and are optimized for high-volume and high-speed read and write operations, making them suitable for web-scale applications, session storage, and caching.
Performance and Scalability
- Hashmap:
- Fast and efficient for in-memory operations.
- Not inherently designed for persistence or distributed environments.
- Key-Value Store:
- Designed to scale horizontally across clusters, providing high availability and fault tolerance.
- Can handle larger datasets since data can be stored on disk and spread across multiple nodes.
Persistence
- Hashmap: Typically lives in the volatile memory, meaning data is lost when the application terminates or the machine reboots unless explicitly serialized to disk.
- Key-Value Store: Often built with durability in mind, offering features like data replication, persistence to disk, and sometimes even multi-region replication for disaster recovery.
Examples and Usage
- Hashmap Examples: In Java,
HashMap
; in Python,dict
; in C++,unordered_map
.
# Example of using a Python dictionary (hashmap)
my_dict = {'key1': 'value1', 'key2': 'value2'}
print(my_dict['key1']) # Output: value1
- Key-Value Store Examples: Redis, Amazon DynamoDB, etcd, Berkeley DB.
# Example of using Redis as a Key-Value store
import redis
r = redis.Redis(host='localhost', port=6379, db=0)
r.set('key1', 'value1')
print(r.get('key1')) # Output: b'value1'
Conclusion
While both hashmaps and key-value stores manage data in key-value pairs, the choice between them depends on the application's requirements such as the need for persistence, scalability across distributed systems, and the handling of large volumes of data.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common Key-Value Databases Questions (and Answers)
- What are the disadvantages of key-value databases?
- What are the advantages of a key-value database?
- Is MongoDB a key-value database?
- How fast are key-value databases?
- What are the differences between key-value stores and relational databases?
- What is the difference between key-value and document databases?
- What are the characteristics and features of key-value store databases?
- What are the differences between key-value databases and Cassandra?
- When should a key-value database not be used?
- How do you design a database using key-value tables?
- Are key-value databases similar to tables in RDBMS?
- Is Redis a key-value store?
White Paper
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
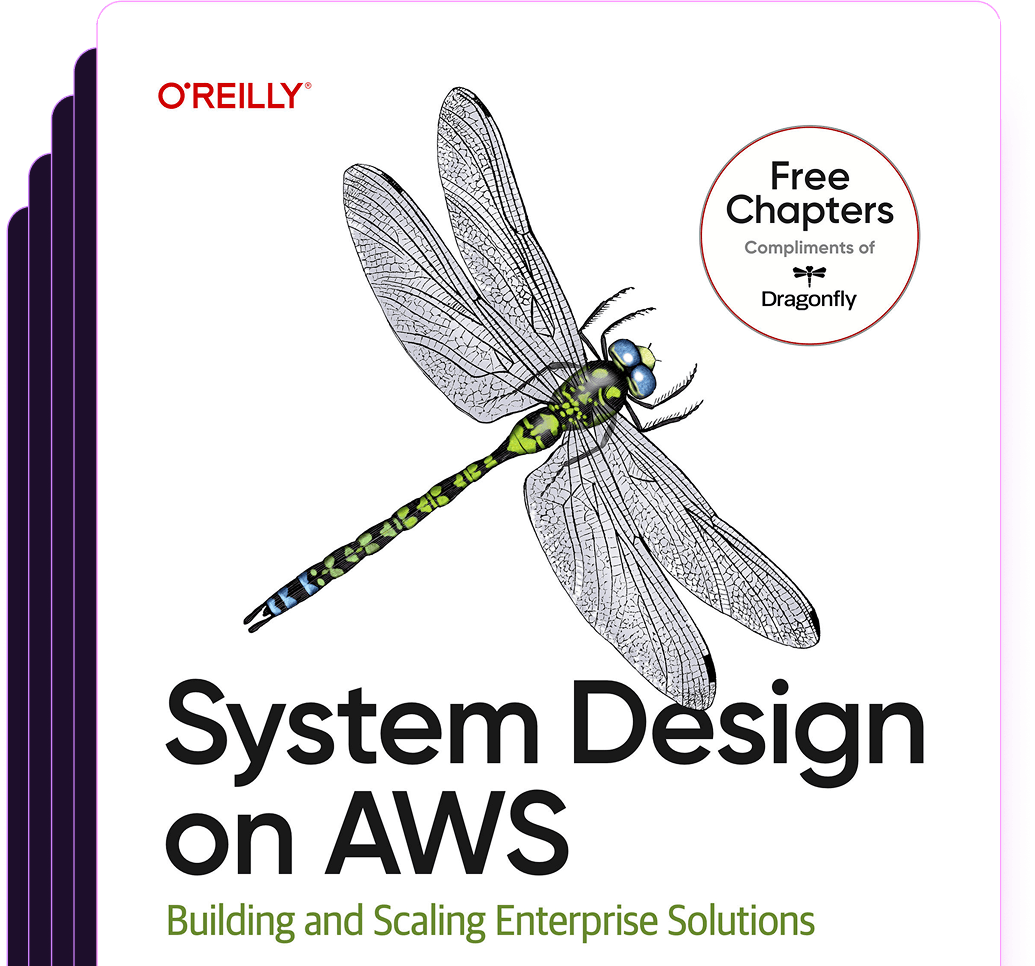
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost