Question: How can I improve MongoDB cursor performance?
Answer
Improving MongoDB cursor performance involves techniques and practices aimed at optimizing query execution, reducing resource consumption, and enhancing the overall efficiency of data retrieval operations. Here are some strategies:
1. Use Indexes Effectively
Indexes can drastically improve query performance by allowing MongoDB to efficiently search for documents. Ensure that your queries are supported by appropriate indexes.
db.collection.createIndex({ field: 1 }); // Creates an index on 'field'
2. Limit Fields in the Projection
When querying, specify only the fields you need. This reduces the amount of data MongoDB has to read and transfer over the network.
db.collection.find({}, { field1: 1, field2: 1 }); // Returns only field1 and field2
3. Use $limit
and $skip
Wisely
For pagination, consider using $limit
to control the number of documents returned. However, $skip
can be inefficient on large collections as it still scans the skipped documents. Consider alternative pagination methods, such as range queries based on indexed fields.
db.collection.find().limit(10); // Limits the result to 10 documents
4. Utilize Cursor Methods for Large Result Sets
For operations that return a large number of documents, use cursor methods like .batchSize()
to control the number of documents returned in each batch, reducing memory usage on both client and server sides.
db.collection.find().batchSize(100);
5. Optimize Sort Operations
Sorting can be resource-intensive, especially for large datasets. Ensure your sort operation uses an index. If sorting by multiple fields, consider compound indexes.
db.collection.createIndex({ field1: 1, field2: -1 }); // Compound index for sorting
6. Monitor and Analyze Query Performance
Use MongoDB's monitoring tools and database commands like explain()
to analyze query performance and optimize further.
db.collection.find().explain('executionStats');
7. Avoid Large In-Memory Sorts
If you must sort a large number of documents, ensure the operation does not exceed the sort memory limit by using an appropriate index.
Conclusion
Improving MongoDB cursor performance is crucial for maintaining fast, efficient applications. By implementing these strategies, you can optimize your MongoDB queries for better speed and resource utilization.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common MongoDB Performance Questions (and Answers)
- How to improve MongoDB query performance?
- How to check MongoDB replication status?
- How do you connect to a MongoDB cluster?
- How do you clear the cache in MongoDB?
- How many connections can MongoDB handle?
- How does MongoDB sharding work?
- How to check MongoDB cluster status?
- How to change a MongoDB cluster password?
- How to create a MongoDB cluster?
- How to restart a MongoDB cluster?
- How do I reset my MongoDB cluster password?
- How does the $in operator affect performance in MongoDB?
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
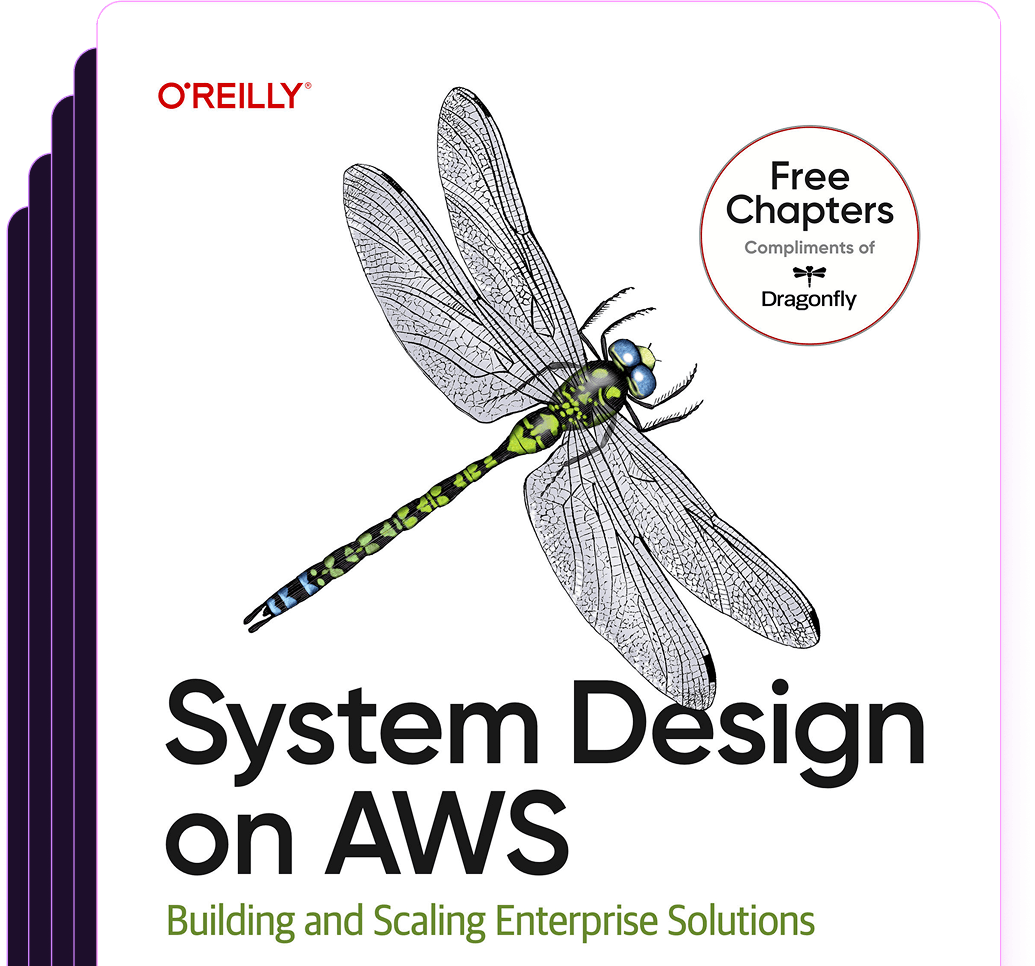
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost