Question: How does the findOneAndUpdate operation affect performance in MongoDB?
Answer
One of the key operations in MongoDB for updating documents is findOneAndUpdate
. This method searches for a document that matches a given filter, updates it according to the update operations provided, and returns either the original document or the updated document, based on the options specified. Understanding its performance implications is crucial for optimizing MongoDB applications.
Performance Considerations
The performance of findOneAndUpdate
depends on several factors:
- Indexes: Ensure that the query part of the operation uses indexed fields. Without indexes, MongoDB performs a collection scan, significantly slowing down the operation, especially as the dataset grows.
- Document Size: The size of the document being updated can impact performance. Larger documents take more time to update, especially if they need to be moved because the updated version doesn't fit in the original document's space on disk.
- Write Concern: The write concern specifies the level of acknowledgment required from MongoDB when writing data. A higher level of write concern (
w: "majority"
, for example) ensures greater data safety but can impact performance due to the additional overhead of waiting for more acknowledgments. - Update Complexity: The complexity of the update operation itself can affect performance. Operations that involve array modifications, especially with operators like
$push
,$pull
, or$addToSet
, might be more resource-intensive than simple field updates.
Optimizing findOneAndUpdate Performance
To optimize performance when using findOneAndUpdate
, consider the following:
- Use Indexes Efficiently: Make sure your query selector is covered by an index. Use the
.explain("executionStats")
method to analyze query performance and indexing. - Project Only Necessary Fields: If you don't need the returned document, or if you only need certain fields, use the projection option to limit the amount of data MongoDB has to read and return.
- Batch Updates: If possible, batch updates together instead of issuing multiple
findOneAndUpdate
calls for individual documents. However, this may not always apply if you need the found document or have to handle each update differently. - Consider Update Patterns: Evaluate whether all updates require the atomicity and immediate feedback of
findOneAndUpdate
. In some cases, batching updates withupdateMany
or using other update methods might be more efficient.
Example
db.collection.findOneAndUpdate(
{ _id: "uniqueDocId" }, // Filter
{ $set: { fieldToUpdate: "newValue" } }, // Update
{
projection: { fieldToShow1: 1, fieldToShow2: 1 }, // Options: Return specific fields
returnOriginal: false, // Return the updated document
upsert: true // Create a document if no documents match the filter
}
);
In conclusion, while findOneAndUpdate
is a powerful and versatile method, its performance depends on careful consideration of factors like indexes, document size, write concern, and the complexity of the update operation. By applying best practices and optimizations, you can ensure that your MongoDB applications run efficiently.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common MongoDB Performance Questions (and Answers)
- How to improve MongoDB query performance?
- How to check MongoDB replication status?
- How do you connect to a MongoDB cluster?
- How do you clear the cache in MongoDB?
- How many connections can MongoDB handle?
- How does MongoDB sharding work?
- How to check MongoDB cluster status?
- How to change a MongoDB cluster password?
- How to create a MongoDB cluster?
- How to restart a MongoDB cluster?
- How do I reset my MongoDB cluster password?
- How does the $in operator affect performance in MongoDB?
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
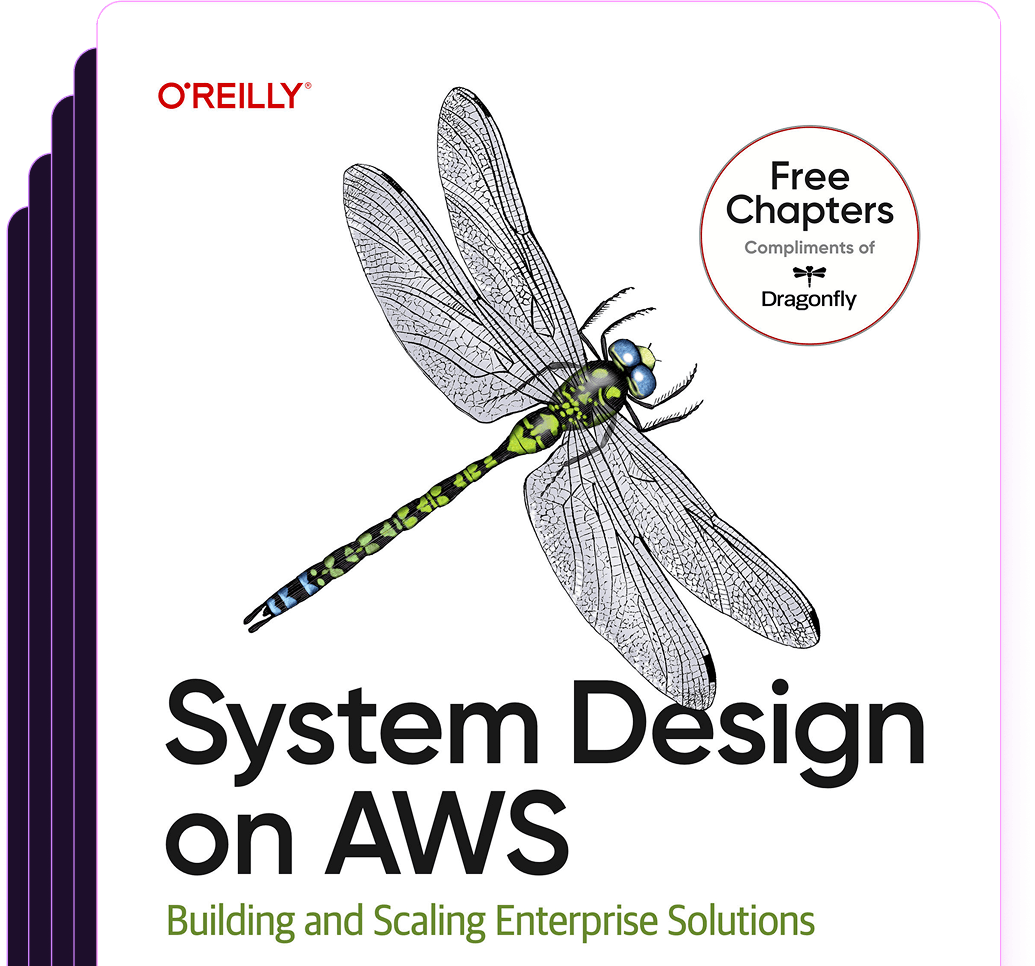
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost