Question: How can I improve MongoDB writes per second?
Answer
Improving MongoDB's write throughput – the number of write operations performed per second – involves several strategies focusing on hardware, database schema design, and MongoDB configuration. Here are some methods to consider:
1. Use WiredTiger Storage Engine
Ensure you're using the WiredTiger storage engine, which is the default for MongoDB versions 3.2 and above. It offers better concurrency and compression, directly impacting write performance.
// To check your current storage engine, run the following command in the mongo shell:
db.serverStatus().storageEngine
2. Sharding
Distribute your data across multiple machines using sharding. This approach splits data into smaller, more manageable parts, allowing for horizontal scaling and distributing write load.
3. RAID Configuration
Use RAID 10 (mirroring and striping) for your disk setup. It offers a balance of speed and redundancy, improving write performance while ensuring data safety.
4. Write Concerns
Adjust your write concern levels based on your application's consistency requirements. Less strict write concerns ({w: 0}
or {w: 1}
) can increase write speed but at the cost of data durability and consistency.
db.collection.insertOne(
{ item: "card", qty: 15 },
{ writeConcern: { w: 1 } }
)
5. Indexing
Ensure that your indexes support your write patterns. Inefficient indexing can slow down writes due to the overhead of updating indexes. However, be cautious not to over-index as each additional index requires updates on write operations.
6. Hardware Improvements
- RAM: More RAM allows MongoDB to keep larger working sets in memory, reducing disk I/O.
- SSD: Solid State Drives significantly improve read/write speeds compared to traditional HDDs.
7. Connection Pooling
Maintain a pool of database connections to avoid the overhead of establishing connections for every write operation. Most MongoDB drivers handle connection pooling automatically.
8. Bulk Operations
Use bulk inserts or updates when possible. Grouping multiple write operations in a single command reduces overhead and improves throughput.
db.collection.bulkWrite([
{ insertOne: { document: { a: 1 } } },
{ updateOne: { filter: {a: 2}, update: {$set: {a: 2}}, upsert:true } },
{ deleteOne: { filter: {c: 1} } }
])
9. Avoid Jumbo Chunks in Sharded Clusters
In sharded setups, ensure chunks are split and balanced properly. Jumbo chunks can prevent efficient distribution of data and operations across shards.
Implementing these strategies can significantly improve your MongoDB write performance. However, always monitor and test changes in a staging environment before applying them to production to understand their impact.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common MongoDB Performance Questions (and Answers)
- How to improve MongoDB query performance?
- How to check MongoDB replication status?
- How do you connect to a MongoDB cluster?
- How do you clear the cache in MongoDB?
- How many connections can MongoDB handle?
- How does MongoDB sharding work?
- How to check MongoDB cluster status?
- How to change a MongoDB cluster password?
- How to create a MongoDB cluster?
- How to restart a MongoDB cluster?
- How do I reset my MongoDB cluster password?
- How does the $in operator affect performance in MongoDB?
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
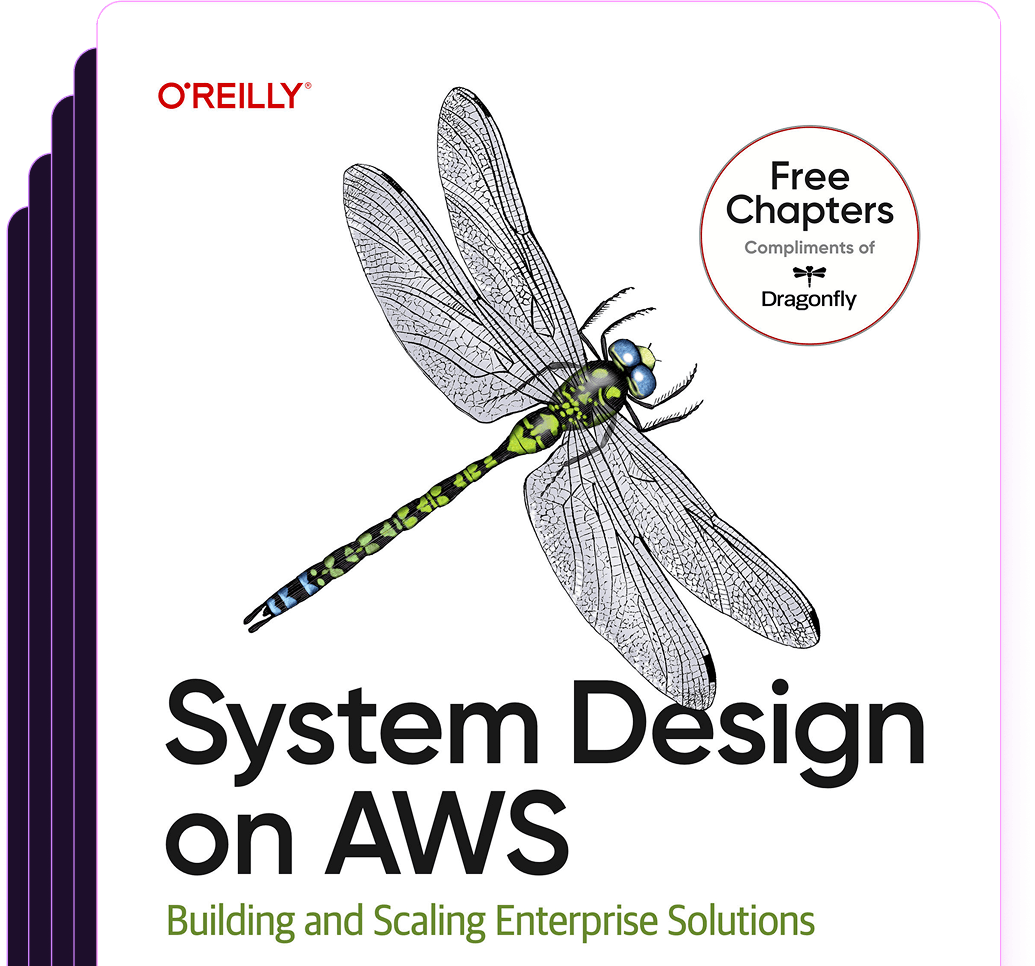
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost