Question: How can I improve MongoDB insert performance?
Answer
Improving MongoDB insert performance involves several strategies that target different aspects of the data insertion process. Here are some key approaches:
1. Use Bulk Operations
When inserting multiple documents, use bulk operations instead of inserting each document individually. This reduces network round trips and can significantly improve performance.
db.collection.insertMany([
{ item: 'item1', qty: 10 },
{ item: 'item2', qty: 20 },
// Add more documents as needed
]);
2. Write Concerns
Lowering the writeConcern
level can increase insertion speed by reducing the guarantees MongoDB provides on the data being written. For example, using { w: 1 }
ensures that the write operation is acknowledged by only the primary server.
db.collection.insert(
{ item: 'item3', qty: 30 },
{ writeConcern: { w: 1 } }
);
However, be cautious as this might compromise data durability.
3. Indexes
While indexes are critical for query performance, each index on a collection also means additional work during inserts, updates, and deletes. Ensure you only maintain necessary indexes and consider dropping them temporarily during large bulk insertion operations if appropriate.
4. Hardware Considerations
- RAM: Ensure MongoDB has enough RAM to keep its working set (the frequently accessed data and indexes) in memory. Insufficient RAM leading to disk swapping can severely degrade performance.
- Disk Type: Use SSDs over HDDs for faster read/write capabilities, crucial for high insertion rates.
- Network Latency: Minimize network latency between your application and MongoDB servers, especially for distributed environments. Consider using MongoDB's intra-cluster compression settings to reduce network bandwidth.
5. Document Size
Smaller documents get processed faster. If possible, limit the size of the documents you're inserting. This is particularly relevant when dealing with large arrays or deeply nested objects.
6. Use WiredTiger Storage Engine
Ensure you're using the WiredTiger storage engine, which is the default from MongoDB version 3.2 onwards. It offers better performance and concurrency capabilities compared to older engines like MMAPv1.
7. Sharding
For extremely large datasets or high-throughput scenarios, consider sharding your MongoDB collection across multiple servers. This distributes the write load but introduces complexity in managing the database.
In conclusion, improving MongoDB insert performance is about finding the right balance between operational requirements, hardware capabilities, and MongoDB's configuration options. Carefully consider the implications of each optimization technique based on your specific use case.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common MongoDB Performance Questions (and Answers)
- How many connections can MongoDB handle?
- How to check MongoDB cluster status?
- How to restart a MongoDB cluster?
- How do I reset my MongoDB cluster password?
- What is a MongoDB cluster proxy and how does it work?
- How does using DBRef affect performance in MongoDB?
- How do you configure a MongoDB replica set with username and password authentication?
- How do you compare times in MongoDB?
- How do MongoDB and Neo4j compare in terms of performance?
- How does WriteConcern affect performance in MongoDB?
- How does MongoDB compare to Oracle in terms of performance?
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
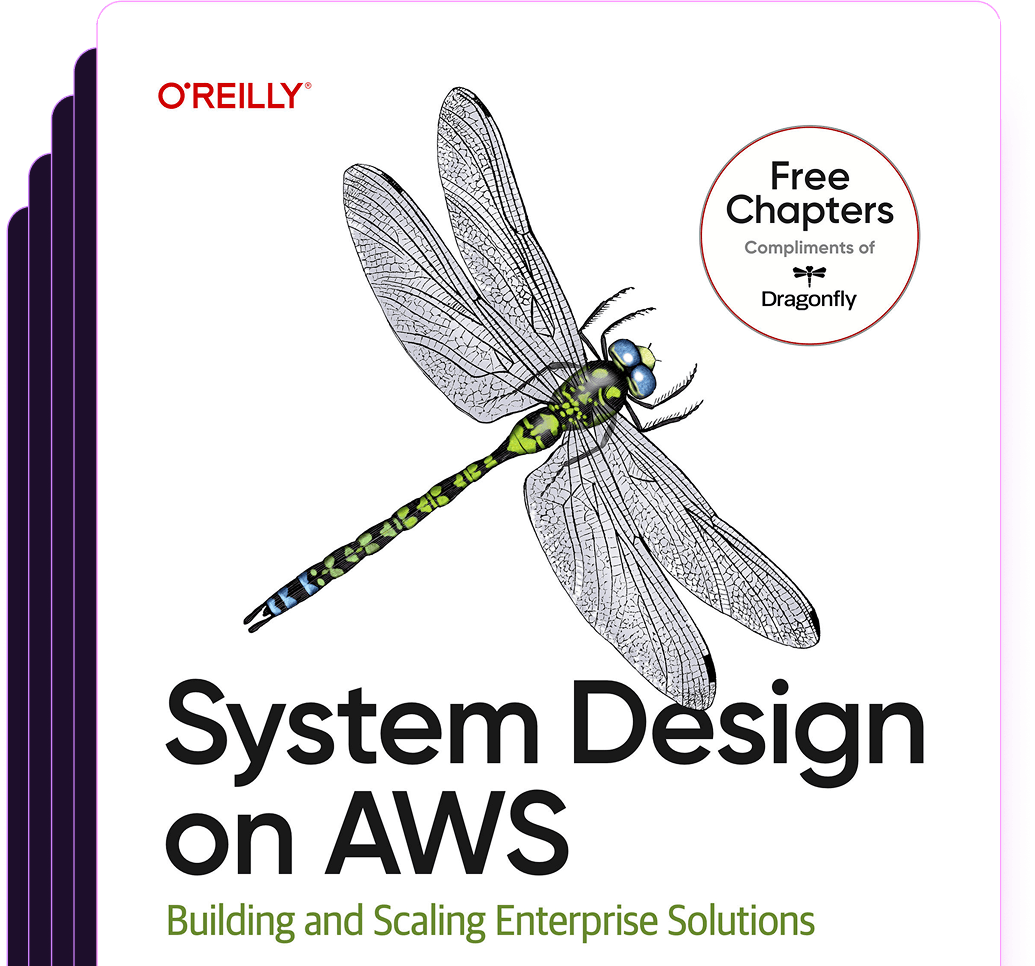
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost