Question: How can you improve performance when sorting in MongoDB?
Answer
Improving performance when sorting in MongoDB involves optimizing your query and indexing strategy to ensure efficient sorting operations. Here are key strategies:
- Use Indexes for Sorting: Creating appropriate indexes on fields you frequently sort by can dramatically increase query performance. When a query uses a sort operation, MongoDB can often use the ordering in an index to avoid an explicit sort step.
```javascript
db.collection.createIndex({ fieldName: 1 }); // Ascending order
db.collection.createIndex({ fieldName: -1 }); // Descending order
```
Ensure that your queries can take advantage of these indexes by matching the query predicates and sort order with the index.
- Compound Indexes: If you're sorting on multiple fields, consider using compound indexes that include all the fields you're sorting on in the correct order.
```javascript
db.collection.createIndex({ firstField: 1, secondField: -1 });
```
- Limit Results: If you are displaying results to users (e.g., in a web application), it often makes sense only to return a subset of the sorted documents. Combining sorting with
.limit()
can significantly reduce the workload on MongoDB by minimizing the amount of data sorted and returned. - Use
$sort
Aggregation Stage Wisely: When using aggregation pipelines, be mindful of where you place the$sort
stage. Early sorting before reducing the dataset size can lead to unnecessary work. Consider filtering or reducing the result set before sorting if possible. - Avoid SORT_STAGE and COLLSCAN: Use the
explain()
method to analyze your queries' execution plans. If you see aSORT_STAGE
without an index (COLLSCAN
), it means MongoDB is performing an in-memory sort, which can be slow and inefficient for large datasets. - Memory Considerations: The MongoDB sort operation has a memory limit of 32MB. For operations that exceed this limit, consider using indexes to sort the data or breaking down the operation into smaller chunks.
- Monitor Performance: Use MongoDB monitoring tools such as MongoDB Atlas's built-in monitoring or the
mongostat
andmongotop
utilities to keep an eye on your database's performance over time, adjusting indexes and queries based on real usage patterns.
By carefully designing your schema, indexing strategy, and queries, you can significantly enhance the performance of sort operations in MongoDB.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common MongoDB Performance Questions (and Answers)
- How to improve MongoDB query performance?
- How to check MongoDB replication status?
- How do you connect to a MongoDB cluster?
- How do you clear the cache in MongoDB?
- How many connections can MongoDB handle?
- How does MongoDB sharding work?
- How to check MongoDB cluster status?
- How to change a MongoDB cluster password?
- How to create a MongoDB cluster?
- How to restart a MongoDB cluster?
- How do I reset my MongoDB cluster password?
- How does the $in operator affect performance in MongoDB?
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
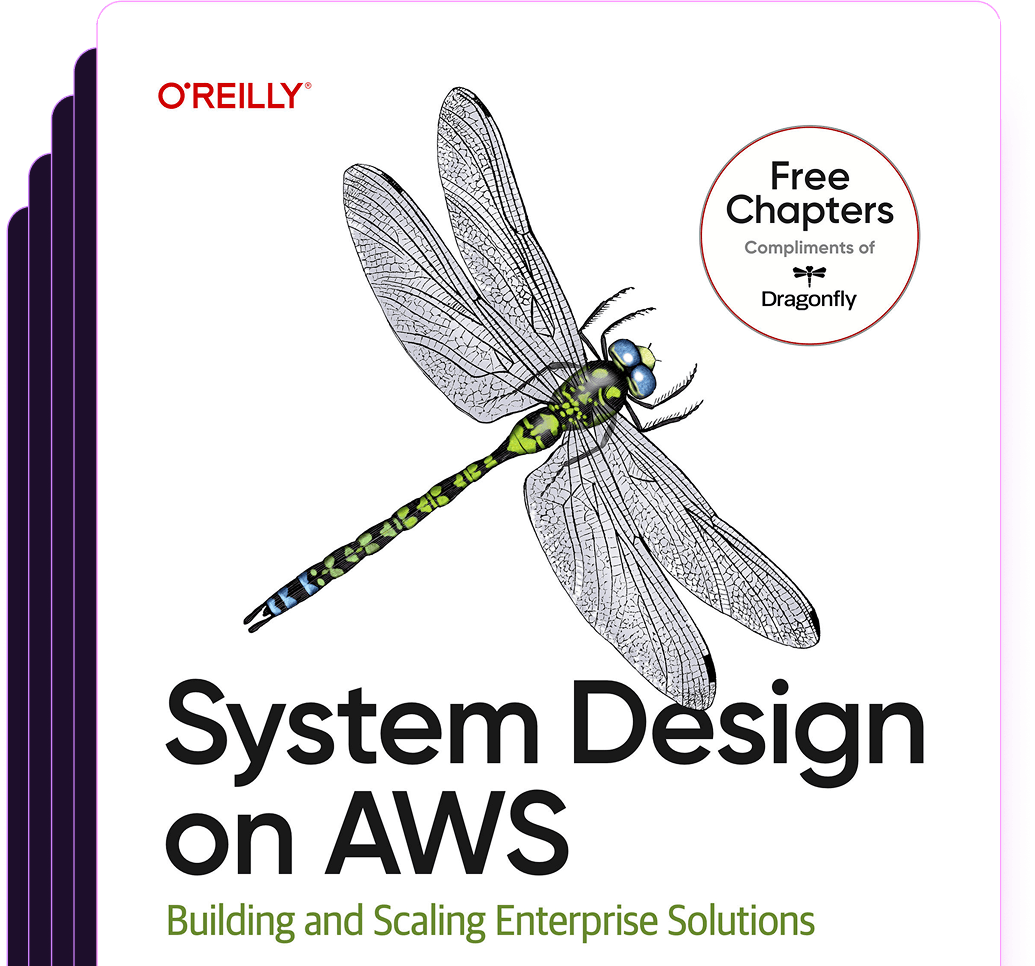
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost