Question: Is MongoDB a key-value database?
Answer
MongoDB is often categorized as a NoSQL database, which means it doesn't use the traditional table-based relational database structure. Instead, it employs a more flexible data storage model, allowing for varied data structures. While it is true that MongoDB can be used similarly to a key-value store, describing it solely as a key-value database simplifies its capabilities too much.
At its core, MongoDB is a document-oriented database. It stores data in flexible, JSON-like documents where fields can vary from document to document. This model allows data to be stored in a way that's more congruent with object-oriented programming, making it easier for developers to work with data.
Key-value stores are a type of database that associate a unique key with a specific value. While MongoDB does use keys (the '_id' field in each document can be considered a key) to access documents, the 'value' in MongoDB is a rich, structured document capable of holding arrays and sub-documents. This goes beyond what is traditionally expected from a key-value store, where values are usually simple strings or blobs.
To illustrate MongoDB's capabilities with an example:
// Connecting to a MongoDB database
const MongoClient = require('mongodb').MongoClient;
const url = "mongodb://localhost:27017/";
const dbName = 'mydatabase';
MongoClient.connect(url, function(err, db) {
if (err) throw err;
const dbo = db.db(dbName);
// Inserting a document into a collection
const myObj = { name: "John Doe", age: 30, address: "123 Main St" };
dbo.collection("customers").insertOne(myObj, function(err, res) {
if (err) throw err;
console.log("1 document inserted");
db.close();
});
});
In this example, while we do have a 'key' (implicitly the '_id' MongoDB provides), the 'value' (the document itself) consists of multiple fields. This illustrates how MongoDB's use case extends well beyond that of a simple key-value store to support complex, nested documents.
In conclusion, while MongoDB can technically perform the functions of a key-value store, its capabilities allow for much more complex and nuanced data modeling, making it better described as a document-oriented database.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common Key-Value Databases Questions (and Answers)
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
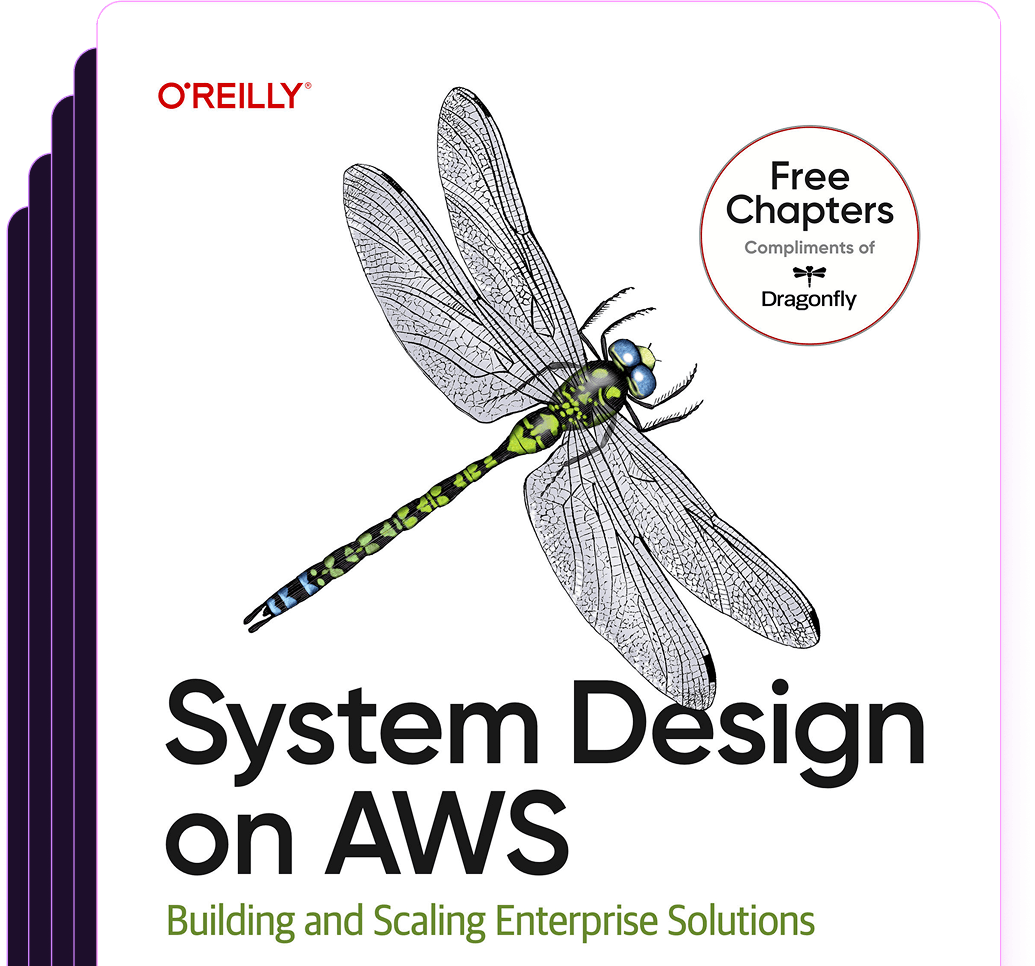
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost