Question: How does the performance of MongoDBs replaceOne method compare to other update methods?
Answer
In MongoDB, the replaceOne
method is used to replace a single document that matches a specified filter with a new document. Understanding the performance impact of using replaceOne
compared to other update operations like updateOne
or updateMany
is essential for optimizing database interactions.
Performance Considerations
- Atomicity:
replaceOne
operates atomically on a single document level, meaning it can be very efficient if you need to replace an entire document as it avoids multiple field-level update commands. - Index Usage: The performance of
replaceOne
greatly depends on how efficiently MongoDB can find the document to replace. Using indexed fields in your filter criteria can significantly speed up the operation. - Write Concern: The write concern level affects the performance of all write operations, including
replaceOne
. A higher write concern level (e.g., requiring acknowledgment from multiple replicas) will lead to slower operations but increased data safety. - Document Size: Replacing a document involves writing the new document to disk. Larger documents require more disk I/O, which can impact performance. Similarly, if the replacement document significantly differs in size from the original, it might lead to additional overhead due to page reallocations in the storage layer.
Code Example
// Assuming a collection named 'products'
const db = client.db('yourDatabaseName');
const collection = db.collection('products');
// Replace a single document matched by `_id`
collection.replaceOne({ _id: someId }, { name: 'New Product Name', category: 'New Category', price: 99.99 })
.then(result => console.log(`Successfully replaced ${result.modifiedCount} document.`))
.catch(err => console.error(`Failed to replace document: ${err}`));
Comparison with updateOne
and updateMany
- Use Case: Use
replaceOne
when you need to replace the entirety of a document. If you only need to modify specific fields within a document,updateOne
orupdateMany
might be more appropriate and potentially more efficient, especially if the changes are minor. - Performance: For small changes,
updateOne
could perform better since it modifies existing fields rather than replacing the whole document. However, for complete document overhauls,replaceOne
can be more straightforward and possibly more efficient.
In summary, the performance of replaceOne
depends on several factors, including document size, index usage, and write concern. It's best used when needing to replace an entire document. Always ensure your query filters use indexed fields to maximize efficiency, and consider the nature of your update operations when choosing between replaceOne
, updateOne
, or updateMany
.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common MongoDB Performance Questions (and Answers)
- How to improve MongoDB query performance?
- How to check MongoDB replication status?
- How do you connect to a MongoDB cluster?
- How do you clear the cache in MongoDB?
- How many connections can MongoDB handle?
- How does MongoDB sharding work?
- How to check MongoDB cluster status?
- How to change a MongoDB cluster password?
- How to create a MongoDB cluster?
- How to restart a MongoDB cluster?
- How do I reset my MongoDB cluster password?
- How does the $in operator affect performance in MongoDB?
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
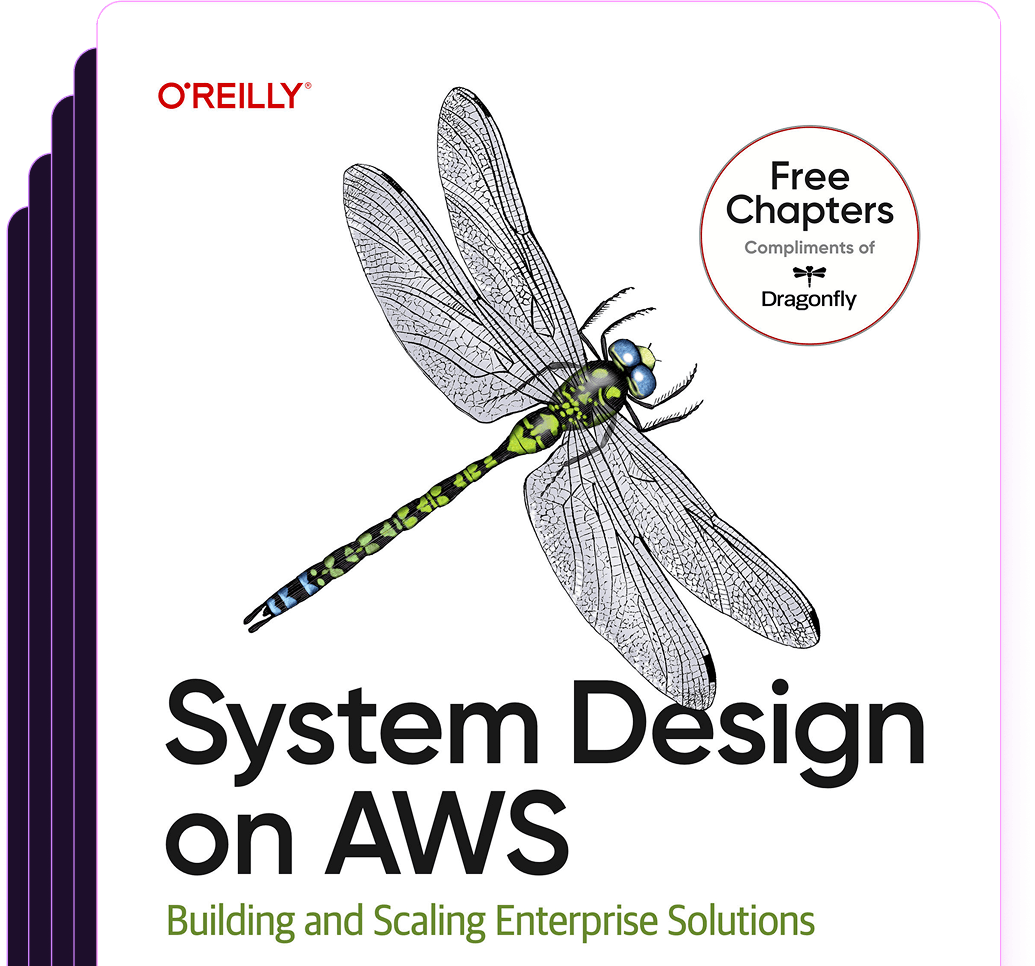
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost