Question: What is the difference between object cache and database cache?
Answer
Object caches and database caches are both crucial components in optimizing the performance of web applications by reducing the load on the database, but they serve different purposes and operate at different layers of the application stack.
Object Cache
An object cache stores fully or partially constructed objects from your application code. It's primarily used to store the results of complex computations, API call responses, or the contents of files that require significant processing time to generate. This type of caching is done in memory, using technologies like Redis or Memcached, to quickly retrieve these objects without needing to reconstruct them from scratch.
Example Usage:
import redis
r = redis.Redis(host='localhost', port=6379, db=0)
# Storing an object (e.g., user profile)
user_profile = {'name': 'John Doe', 'age': 30}
r.set('user:1000', json.dumps(user_profile))
# Retrieving an object
user_data = r.get('user:1000')
if user_data:
user_profile = json.loads(user_data)
Database Cache
A database cache, on the other hand, specifically refers to caching the results of queries made to a database. It can significantly reduce the time to fetch data by avoiding repeated executions of expensive SQL queries. Database caching can be managed directly by the database management system (DBMS) itself or implemented externally using a caching layer.
Example Usage:
Many modern database systems have built-in cache mechanisms that automatically store the results of queries. For explicit caching with a tool like Redis, you could do something similar to the above example but for query results:
```python
query = "SELECT * FROM users WHERE id = 1000"
cache_key = f"query:{hash(query)}"
Check if the result is in cache
result = r.get(cache_key)
if result:
data = json.loads(result)
else:
# Execute the query in the database
data = execute_query_in_database(query)
# Store the result in cache for future requests
r.set(cache_key, json.dumps(data))
```
Differences
- Scope: Object caching is broader and can include any serializable data generated within an application, while database caching is specifically focused on the results of database queries.
- Implementation: Object caches are typically implemented within the application code or an intermediary layer using tools like Redis or Memcached. Database caching might be managed internally by the DBMS or through an external cache.
- Use Cases: Use object caching when the cost of constructing an object is high, regardless of its source. Use database caching to optimize frequent, expensive queries to a database.
In summary, choosing between object caching and database caching depends on what aspect of your application needs optimization. Often, the most effective approach involves a combination of both strategies to minimize database load and improve the overall performance of your application.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common Database Performance Questions (and Answers)
- What is the difference between database latency and throughput?
- What is database read latency and how can it be reduced?
- How can you calculate p99 latency?
- How can one check database latency?
- What causes latency in database replication and how can it be minimized?
- How can you reduce database write latency?
- How can you calculate the P90 latency?
- How can you calculate the p95 latency in database performance monitoring?
- How can you calculate the p50 latency?
- What is database latency?
- What are the causes and solutions for latency in database transactions?
- What is the difference between p50 and p95 latency in database performance metrics?
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
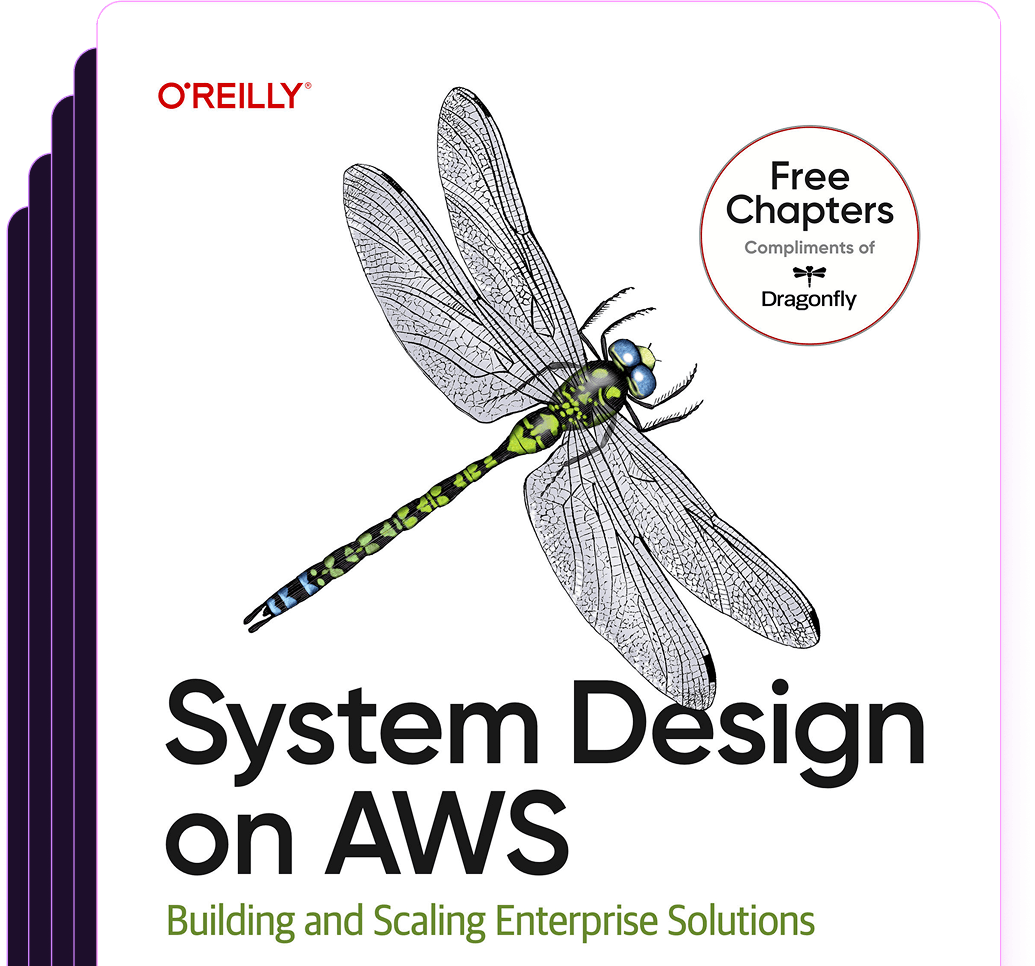
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost