Question: How do you use triggers for partitioning in PostgreSQL?
Answer
Partitioning in PostgreSQL is a technique used to divide large tables into smaller, more manageable pieces, called partitions, based on certain keys such as dates or IDs. While PostgreSQL introduced declarative partitioning since version 10, which simplifies the partitioning process, there are scenarios where using triggers for partition maintenance can be useful, especially in older versions or for complex partitioning logic that declarative partitioning might not directly support.
Using Triggers for Partitioning
Triggers in PostgreSQL are database callbacks that are automatically executed or fired when certain events occur. In the context of partitioning, triggers can be used to automatically insert rows into the correct partition based on some logic written inside the trigger function.
Here's a step-by-step guide on how to set up partitioning using triggers:
- Define Master Table: This is the table that will represent all partitions logically.
- Create Partition Tables: These are the actual partitions. They should have the same structure as the master table.
- Write a Trigger Function: This function contains the logic to direct rows to the appropriate partition.
- Attach Trigger to Master Table: The trigger fires upon inserts into the master table and calls the trigger function to route the row to the right partition.
Example
Let's assume we want to partition a table named logs
by month.
```sql
-- Step 1: Create the master table
CREATE TABLE logs (
log_id serial PRIMARY KEY,
log_text text NOT NULL,
log_date date NOT NULL
);
-- Step 2: Create partition tables (for January and February, as examples)
CREATE TABLE logs_january PARTITION OF logs FOR VALUES FROM ('2022-01-01') TO ('2022-02-01');
CREATE TABLE logs_february PARTITION OF logs FOR VALUES FROM ('2022-02-01') TO ('2022-03-01');
-- For older PostgreSQL or manual partitioning:
-- CREATE TABLE logs_january (
-- CHECK (log_date >= DATE '2022-01-01' AND log_date < DATE '2022-02-01')
-- ) INHERITS (logs);
-- Repeat for February...
-- Step 3: Create the trigger function
CREATE OR REPLACE FUNCTION logs_insert_trigger()
RETURNS TRIGGER AS $$
BEGIN
IF (NEW.log_date >= DATE '2022-01-01' AND NEW.log_date < DATE '2022-02-01') THEN
INSERT INTO logs_january VALUES (NEW.*);
ELSIF (NEW.log_date >= DATE '2022-02-01' AND NEW.log_date < DATE '2022-03-01') THEN
INSERT INTO logs_february VALUES (NEW.*);
-- Add more ELSEIF clauses for other months/partitions
ELSE
RAISE EXCEPTION 'Date out of range. No partition found for %', NEW.log_date;
END IF;
RETURN NULL; -- Since this is an AFTER INSERT trigger, it doesn't need to return anything
END;
$$ LANGUAGE plpgsql;
-- Step 4: Attach the trigger to the master table
CREATE TRIGGER insert_logs_trigger
BEFORE INSERT ON logs
FOR EACH ROW EXECUTE FUNCTION logs_insert_trigger();
```
This example demonstrates basic partition management using triggers. It's just the tip of the iceberg, and real-world scenarios might require more sophisticated logic, depending on the application's needs.
Points to Consider
- Performance: Using triggers might add overhead to your insert operations. Test to ensure performance meets your requirements.
- Maintenance: Complex partitioning logic in triggers can become hard to manage. Ensure your partitioning strategy remains maintainable.
- Declarative Partitioning: If possible, prefer declarative partitioning for its simplicity and better integration within PostgreSQL.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common PostgreSQL Questions (and Answers)
- How do you manage Postgres replication lag?
- How can I limit the number of rows updated in a PostgreSQL query?
- How does sharding work in PostgreSQL?
- How do you limit the number of rows deleted in PostgreSQL?
- How do you use the PARTITION OVER clause in PostgreSQL?
- What are PostgreSQL replication slots and how do they work?
- How can you partition an existing table in PostgreSQL?
- How do you partition a table by multiple columns in PostgreSQL?
- How do you check the replication status in PostgreSQL?
- What are the scaling limits of PostgreSQL?
- How do you scale Azure PostgreSQL?
- How do you use the limit clause in PostgreSQL to get the top N rows of a query result?
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
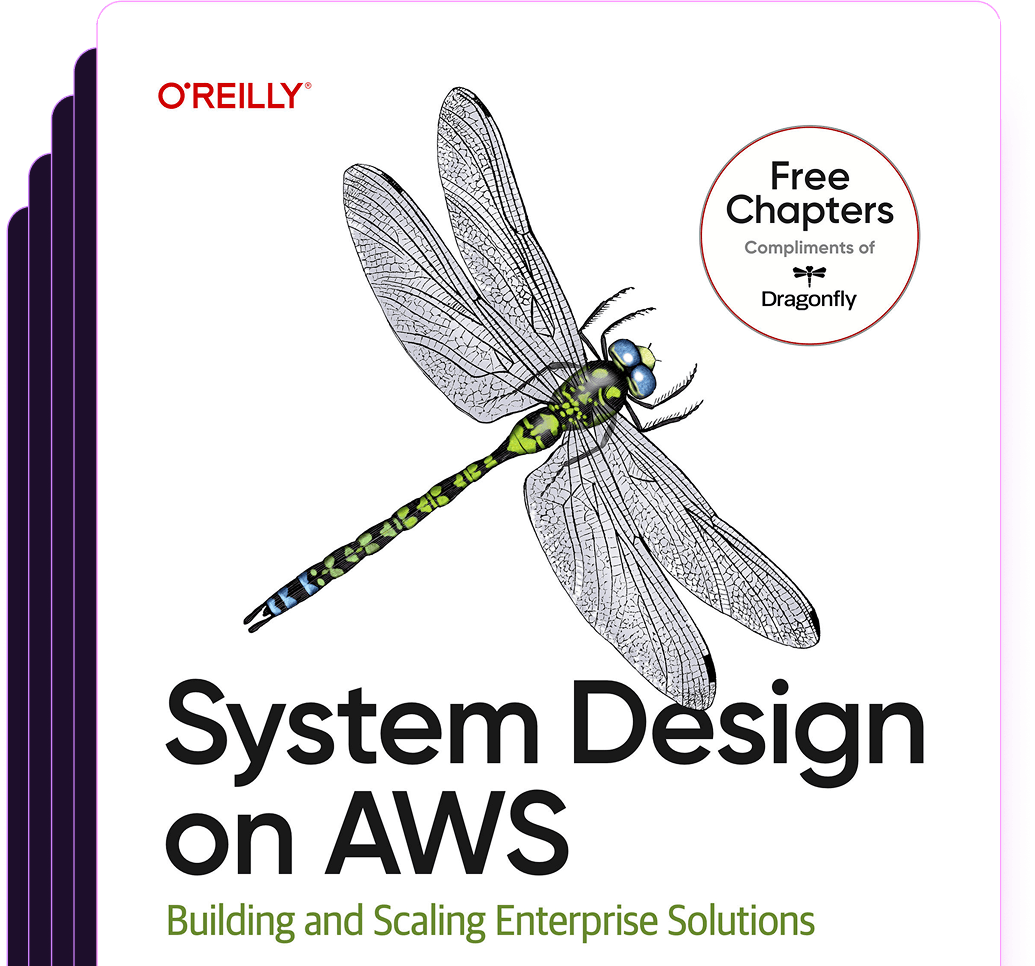
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost