Question: What is a persistent object cache and how can one implement it?
Answer
A persistent object cache refers to a caching technique where objects are stored in a persistent storage, such as a disk or database. This allows for quicker retrieval of the data, as the system bypasses the need to recompute or fetch the data from an original complex, time-consuming source.
Persistent object caches are especially beneficial when working with expensive data operations, like database queries or API calls, which you might prefer to perform once and then cache for subsequent use.
A common way to implement a persistent object cache is using Redis or Memcached along with a language-specific client. Below is a simple example of implementing a persistent object cache using Python and Redis:
Install the redis-py client:
pip install redis
And then, a Python script to store and retrieve a cache object would look something like this:
import redis
import json
# Connect to local redis instance
r = redis.Redis(host='localhost', port=6379, db=0)
object_key = 'user:1234'
data = {
"name": "John Doe",
"email": "john.doe@example.com"
}
# Save object as JSON string
r.set(object_key, json.dumps(data))
print('Data saved in cache')
# Retrieve and load object
cache_data = r.get(object_key)
if cache_data:
print('Data retrieved from cache')
data = json.loads(cache_data)
else:
print('No data in cache')
In this example, we're storing a Python dictionary as a JSON string in Redis. The key is user:1234
and the value is the JSON string. When retrieving the data from cache, we check if data exists for the key. If the data exists, we load it back into a Python dictionary.
Remember that while caching can dramatically improve performance, it also adds complexity to your application. You need to ensure consistency between your cache and the original data sources.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common In Memory Questions (and Answers)
- What is a Distributed Cache and How Can It Be Implemented?
- How do you design a distributed cache system?
- How can I set up and use Redis as a distributed cache?
- Why should you use a persistent object cache?
- What are the differences between an in-memory cache and a distributed cache?
- What is AWS's In-Memory Data Store Service and how can it be used effectively?
- What is a distributed cache in AWS and how can it be implemented?
- How can you implement Azure distributed cache in your application?
- What is the best distributed cache system?
- Is Redis a distributed cache?
- What is the difference between a replicated cache and a distributed cache?
- How can you implement a distributed cache using Docker?
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
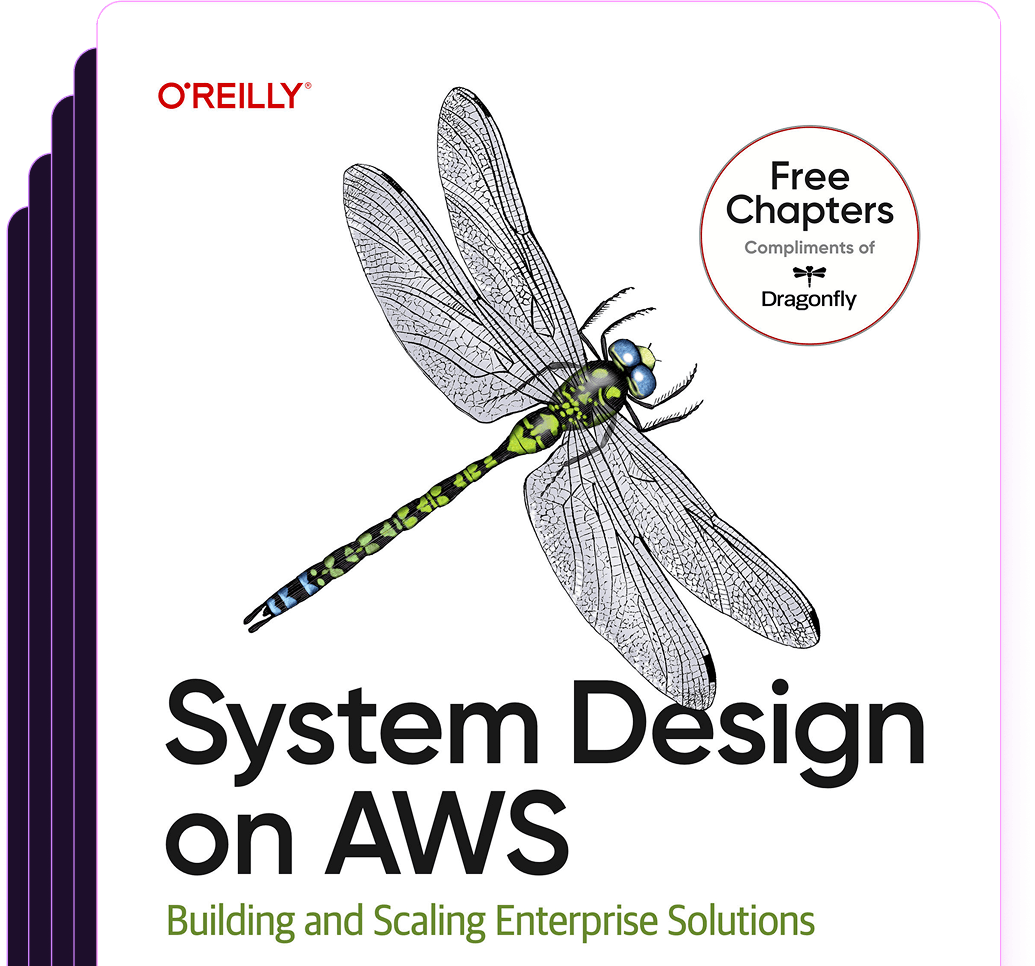
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost