Question: How to Refresh Redis Cache in Spring Boot?
Answer
Refreshing the Redis cache in a Spring Boot application can be achieved using the CacheManager
interface offered by Spring Framework. This interface is instrumental in managing and manipulating caches, including refreshing cache entries.
Follow these steps to refresh the Redis cache in your Spring Boot application:
- Set up the cache manager in your Spring Boot application: Create a method annotated with
@Bean
in your configuration class that produces aRedisCacheManager
instance.
@Configuration
@EnableCaching // Enable caching in Spring application
public class CacheConfiguration {
// Auto-wiring the RedisConnectionFactory
@Autowired
private RedisConnectionFactory redisConnectionFactory;
// Bean definition for CacheManager
@Bean
public CacheManager cacheManager() {
// Define cache configuration
RedisCacheConfiguration cacheConfiguration =
RedisCacheConfiguration.defaultCacheConfig()
.entryTtl(Duration.ofMinutes(10)); // This sets the time to live for cache entries
// Return a new RedisCacheManager built with default configurations and transaction awareness
return RedisCacheManager.builder(redisConnectionFactory)
.cacheDefaults(cacheConfiguration)
.transactionAware()
.build();
}
}
- Refresh the cache programmatically using
CacheManager
: In your service or component class, inject theCacheManager
bean and use it to clear cache entries (thus "refreshing" them).
@Service // Marks this class as a candidate for Spring's component scanning to detect and add beans
public class YourService {
// Auto-wiring the CacheManager within your service
@Autowired
private CacheManager cacheManager;
// Method responsible for refreshing the cache
public void refreshCache(String cacheName) {
Cache cache = cacheManager.getCache(cacheName);
if (cache != null) {
cache.clear(); // Clears all entries from the cache, effectively refreshing it
}
}
// Other methods and code for your service go here
}
- Call the
refreshCache
method to refresh the cache: From your controller or another class, you can now call therefreshCache
method to refresh your cache.
@RestController // Marks this class as a controller where every method returns a domain object instead of a view
@RequestMapping("/api") // Maps incoming requests to the correct method
public class YourController {
// Auto-wiring your custom service within your controller
@Autowired
private YourService yourService;
@PutMapping("/refresh-cache/{cacheName}") // Mapping for PUT requests on "/api/refresh-cache/{cacheName}"
public ResponseEntity<String> refreshCache(@PathVariable String cacheName) {
yourService.refreshCache(cacheName);
return ResponseEntity.ok("Cache " + cacheName + " has been refreshed.");
}
// Other methods and code for your controller go here
}
You can now refresh a specific Redis cache in your Spring Boot application by sending a PUT request to /api/refresh-cache/{cacheName}
.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common Redis Questions (and Answers)
White Paper
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
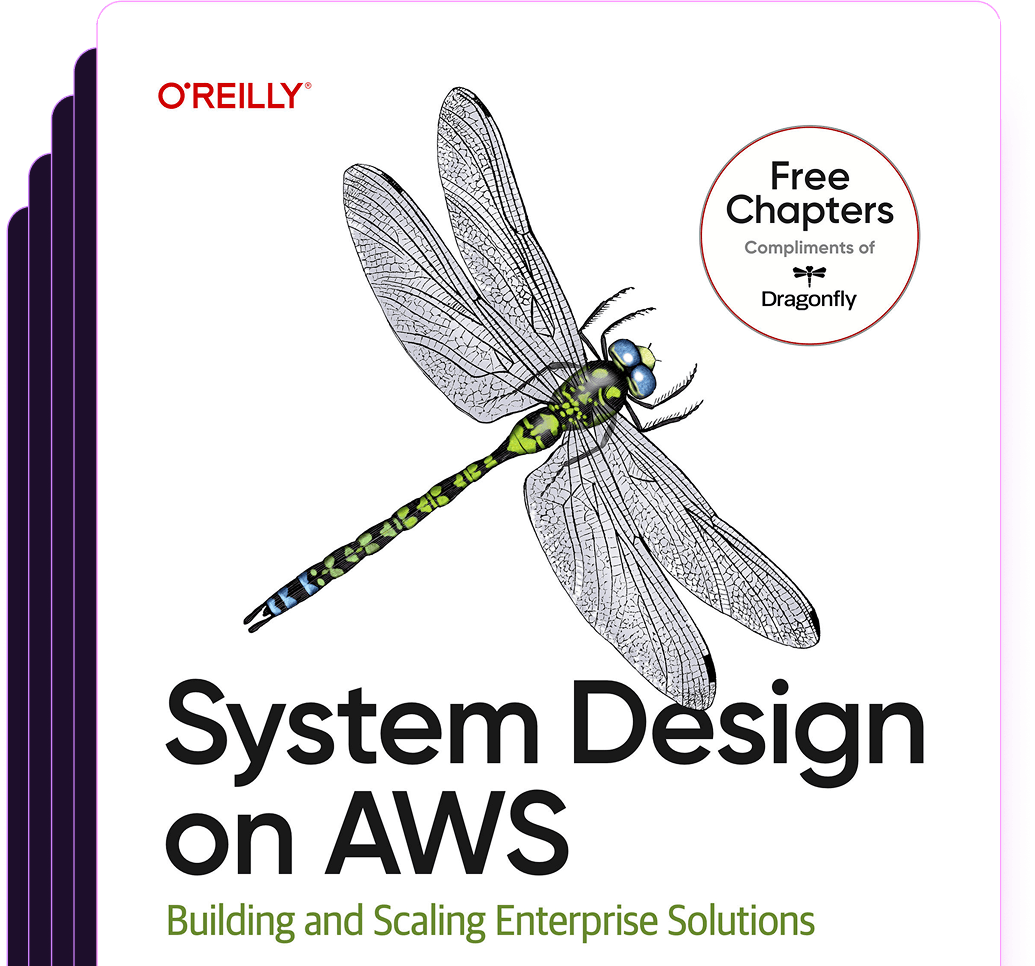
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost