BullMQ - Ultimate Beginners Guide + Getting Started Tutorial
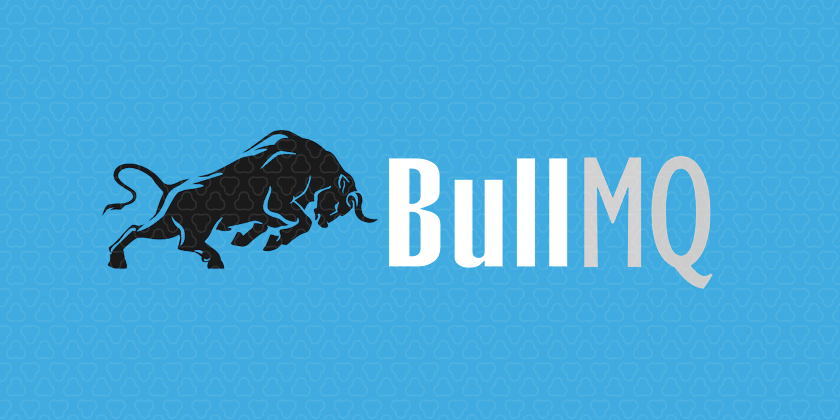
BullMQ Basics
What's BullMQ?
BullMQ is an advanced Node.js queue solution for handling distributed jobs and messages in Redis. It was built on top of the Bull library, enhancing the performance and functionality while maintaining a focus on reliability.
The history of BullMQ dates back to 2014 when it was released as an open-source project named "Bull". The original Bull project was widely adopted due to its high-speed processing capabilities and low memory footprint. However, developers felt the need for even more powerful features, and thus, BullMQ was born in 2019.
Since then, BullMQ has gained quite a reputation within the developer community. Its proven reliability combined with new feature additions makes it a popular choice for managing workloads in distributed systems.
How BullMQ Works
At a basic level, BullMQ creates job queues in Dragonfly or Redis (powerful in-memory data stores). These job queues are essential lists of tasks waiting to be processed. Workers, which are essentially separate processes or threads, pick up these jobs and execute them.
Jobs are added to a queue, and each job involves data and options. Once a job has been processed, it can either be completed successfully or fail due to errors. In case of failure, the job can be retried based on your specified settings.
Core Features & Advantages of BullMQ
Scalability
Scalability is one of the key features of BullMQ. Since it leverages Dragonfly/Redis, you can easily scale your applications by increasing the number of workers that process jobs. You have complete control over how many workers you want to use based on the workload.
Priority Queuing
Not all jobs are created equal. Some tasks may require immediate attention. BullMQ offers priority queuing, meaning that you can assign higher priority levels to certain jobs making them jump ahead in the queue.
// Example of creating a job with priority const job = await queue.add('myJobName', {foo: 'bar'}, {priority: 1});
Job Events and Listeners
BullMQ provides the ability to listen for specific events on job queues. These include events like completed
, failed
, stalled
etc. Using these listeners, you can monitor and react to changes in the processing lifecycle.
// Example of listening to a completed event queue.on('completed', (job, result) => { console.log(`Job ${job.id} completed with result ${result}`); });
Rate Limiter
Another notable feature is its built-in rate limiter, which prevents your job processing from overloading your system or a third-party API. By setting the rate limit, you ensure that only a certain number of jobs are processed within a specified duration.
// Example of adding a queue with rate limit const queue = new Queue('myQueue', { limiter: { max: 1000, duration: 5000, }, });
Repeatable Jobs
Certain tasks need to be performed over regular intervals. With BullMQ's repeatable jobs feature, you can easily schedule such tasks without the worry of manual re-entries.
// Example of creating a repeatable job queue.add('myRepeatJob', {foo: 'bar'}, {repeat: {cron: '*/5 * * * *'}});
Top BullMQ Use Cases
BullMQ can be used in a variety of applications. Here are some common use cases:
-
Background Tasks: From image processing to sending emails, background tasks are well-suited for BullMQ.
-
Scheduled Jobs: If you have jobs that need to run at specific times, like nightly database backups or scheduled notifications, BullMQ has you covered.
-
Rate-limited API Requests: If you're interacting with third-party APIs that have rate limits, the built-in rate limiter of BullMQ can be a lifesaver.
-
Processing Large Data: When dealing with large datasets, processing data in smaller chunks as jobs can greatly improve performance and efficiency.
BullMQ's features make it an ideal choice for managing workloads in distributed systems and microservices architecture. With its simplicity and ease-of-use, it's no wonder that more developers are adopting BullMQ for their job queue needs.
Key Concepts and Terminologies in BullMQ
Jobs, Queues, and Workers - What They Are
In the context of BullMQ, a job represents a single unit of work. Each function or task that needs to be executed asynchronously can be defined as a job, and every job has a unique ID.
A queue is essentially a container for these jobs. It's a list where jobs are added, organized, and awaited to be processed. One queue can contain multiple jobs, making it a way to manage the execution order of tasks. You can also create multiple queues for different types of jobs.
const Queue = require('bullmq').Queue; const myQueue = new Queue('PaintingQueue'); myQueue.add('Paint Mona Lisa', {color: 'blue'});
Workers, on the other hand, are responsible for consuming or performing the jobs from the queue. One worker can handle multiple jobs from a queue, but each job will be processed by only one worker at a time.
const Worker = require('bullmq').Worker; new Worker('PaintingQueue', async (job) => { paintMonaLisa(job.data.color); });
Understanding Events and Listeners
Events and listeners in BullMQ play significant roles in maintaining a healthy and efficient workflow. An event is an action or occurrence recognized by the software that may be handled by the program. These could be "job completed," "job failed," or even "queue drained."
myQueue.on('completed', (job, result) => { console.log(`Job with id ${job.id} has been completed`); });
A listener, in general, is a procedure or function in a computer program that waits for an event to occur. In other words, these methods react to specific events and are used to handle their side effects.
Importance of Job Priority and Rate Limiting
In any queue system, the order of execution matters. That's where job priority comes into play. Job priority lets you control the sequence in which jobs are processed. A job with a higher priority will be executed before a low-priority job. In BullMQ, you can assign a priority level when adding a job to the queue.
myQueue.add('Paint Mona Lisa', {color: 'blue'}, {priority: 1});
Rate limiting is another crucial feature offered by BullMQ, ensuring you don't overload your resources by processing too many jobs at once. This allows you to limit the number of jobs processed within a defined time period.
const rateLimitedQueue = new Queue('painting', { limiter: { max: 100, duration: 5000 } });
In this example, BullMQ ensures no more than 100 jobs are processed every 5 seconds.
What a Job Lifecycle Looks Like
Understanding a job lifecycle is key to efficiently managing your tasks. The typical job lifecycle in BullMQ follows these steps:
- Adding a Job: The journey begins when a job is added to a queue.
- Waiting: Jobs wait in the queue until a worker is available to process them.
- Active: When a worker picks up a job, it moves into the active state.
- Completed/Failed: Once the job is processed, depending on the outcome, it moves to either the completed queue or the failed queue.
- Delayed: If some jobs are set to be processed in the future, they reside in the delayed state until their designated time arrives.
- Repeat: For recurring jobs, after completion, they go back to the delayed state for the next run.
BullMQ provides events for all these states, enabling your application to react accordingly. Each of these steps is crucial for maintaining a smooth and efficient processing flow.
myQueue.on('completed', (job, result) => { console.log(`Job with id ${job.id} has been completed`); });
Now that we've understood these key concepts, let's get started with the tutorial and do an example BullMQ implementation.
Getting Started with BullMQ
Installation and Setup of BullMQ
Prerequisites
Before proceeding with the setup of BullMQ, you need a few things ready on your system:
- Node.JS - Ensure you have NodeJS installed (at least version 10.x.x). You can check this by running
node -v
in your terminal. - Dragonfly or Redis - As BullMQ depends on Dragonfly or Redis, ensure either of them are installed and running. You can verify this by running
redis-cli ping
. If it returnsPONG
, you're good to go. (Theredis-cli ping
command will work for both data stores since Dragonfly has a Redis compatible API)
Installing BullMQ and Required Packages
Installation of BullMQ is pretty straightforward if you have Node.js up and running. Simply use the following command:
npm install bullmq --save
This command installs BullMQ and adds it to your project's dependencies.
Setting Up a Basic Queue and Job Processor
Once we've installed BullMQ, let's set up a basic queue and job processor.
Here's a simple example to kick off:
const { Queue } = require('bullmq'); // Initialize a new queue const myQueue = new Queue('my-queue'); // Add jobs to the queue myQueue.add('myJob', { foo: 'bar' });
This snippet creates a new queue named 'my-queue' and adds a job named 'myJob' with data {foo: 'bar'}
to it.
Writing Your First Job Processor with BullMQ
Let's proceed with writing a job processor for our previously created job.
const { Worker } = require('bullmq'); // Initialize a new worker const myWorker = new Worker('my-queue', async job => { // Process the job data console.log(job.data); });
This code initiates a worker that starts processing jobs from 'my-queue'. When it processes our 'myJob', it will log {foo: 'bar'}
to the console.
Explanation of Each Step
- We import
Worker
from BullMQ. - We initialize a new worker with two parameters: the queue name to watch ('my-queue') and a function to handle job processing. The function is asynchronous, allowing us to do operations that may take some time, like database calls or HTTP requests.
- Inside the processing function, we simply log the job's data. In a real-world scenario, your processing would likely be more complex.
Handling Job Events
BullMQ allows you to listen to events such as when a job has been completed or failed. This is extremely useful for tracking job progress or handling errors.
Here's how you can set up event listeners:
myWorker.on('completed', (job) => { console.log(`Job with ID ${job.id} has been completed.`); }); myWorker.on('failed', (job, err) => { console.error(`Job with ID ${job.id} has failed with error: ${err.message}`); });
This will log a message every time a job completes or fails.
Error Handling and Logging
Proper error handling and logging are crucial for debugging and maintaining your applications.
With BullMQ, errors in a job processing function will cause the job to fail:
const badWorker = new Worker('my-queue', async job => { throw new Error('Something went wrong'); });
This job will immediately fail, triggering the 'failed' event.
Remember to add proper logging and error handling mechanisms in your production apps. Logging packages like Winston or Bunyan can help manage logs better, and error tracking services like Sentry can notify you when errors occur.
Common Challenges in Using BullMQ (w/ Solutions)
- Memory Leaks: In some cases, you might find your application consuming more memory than expected. It's often due to job data not being removed after completion, causing the Redis database to fill up.
Always ensure you're calling the job.remove()
function after the job has completed. If a job isn't required to be kept, it should be removed to free up space.
queue.on('completed', async (job) => { await job.remove(); });
- Stalled Jobs: Stalling occurs when a job has been started but has not completed or failed within its timeout period. This issue is difficult to predict as it can be caused by various factors like process crashes or lack of error handling.
Monitor your application to identify and rectify stalled jobs as soon as possible. Using queue.on('stalled', (job) => {})
, you can handle stalled jobs effectively.
- Misunderstanding Priority Queuing: Priority queues in BullMQ aren't strictly followed in a linear fashion, which can lead to confusion if misunderstood.
Remember that priority in BullMQ is more like a weighting system that affects the likelihood of jobs being selected next. It doesn't guarantee strict order. Remember that priority in BullMQ is more like a weighting system that affects the likelihood of jobs being selected next. It doesn't guarantee strict order.
- Difficulty with Event Handling: Event handling with BullMQ can be complex, especially distinguishing between global and local events.
Understand the difference between local events (which apply to a specific job) and global events (which apply across all jobs). Use the appropriate listeners for each scenario.
- Concurrency and Scaling Issues: If not handled correctly, concurrency can lead to problems scaling your queue across multiple workers or processes.
Be sure to correctly set your concurrency limits when creating your queue, and consider using separate queues or even separate Redis instances to allow jobs to process concurrently.
const myQueue = new Bull('myQueue', { limiter: { max: 1000, duration: 5000 } });
- Lack of Idempotency: BullMQ does not enforce idempotency on jobs -- repeating jobs with the same name doesn't automatically prevent duplication.
You can enforce idempotency by using job IDs effectively. This way, you can skip adding the job if it already exists in the queue with the same ID.
try { await queue.add('myJob', data, { jobId: 'uniqueId' }); } catch (error) { if (error.message !== 'Job already exists') { throw error; } // Job already in queue, no need to add again.
Keep these common pitfalls in mind when working with BullMQ. They'll help ensure that your job queue works efficiently and effectively, while also preventing issues that could compromise your application's performance.
Conclusion
BullMQ offers an effective solution for managing tasks and jobs in Node.js applications. With its broad range of capabilities - from handling multiple queues to processing delayed jobs - BullMQ truly stands out as a powerful library. So dive in, explore its features, and let BullMQ streamline your task management processes.
BullMQ - FAQ
Start building today
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement.