Redis XCLAIM in Golang (Detailed Guide w/ Code Examples)
Use Case(s)
In a stream data structure, the XCLAIM command is used when a consumer group needs to take over pending messages from another consumer. This is needed when the original consumer that fetched the message failed to acknowledge it within a specified timeout or crashed.
Code Examples
Here's an example of how you might use XCLAIM
with the Go go-redis
client. In this case, we're going to claim messages that have not been acknowledged for at least 5000 milliseconds (5 seconds).
package main
import (
"fmt"
"github.com/go-redis/redis/v7"
)
func main() {
client := redis.NewClient(&redis.Options{
Addr: "localhost:6379",
Password: "",
DB: 0,
})
streams := []string{"mystream"}
group := "mygroup"
consumer := "consumer1"
msgs, err := client.XPendingExt(&redis.XPendingExtArgs{
Stream: streams[0],
Group: group,
Start: "-",
End: "+",
Count: 10,
}).Result()
if err != nil {
panic(err)
}
for _, msg := range msgs {
xclaimArgs := &redis.XClaimArgs{
Stream: streams[0],
Group: group,
Consumer: consumer,
MinIdle: 5000,
Messages: []string{msg.ID},
}
msgs, err := client.XClaim(xclaimArgs).Result()
if err != nil {
panic(err)
}
for _, msg := range msgs {
fmt.Println("Claimed message ID: ", msg.ID)
}
}
}
In the above code, we first check for all pending messages of the mystream
stream and mygroup
group. We then claim any messages that have been idle for more than 5000 milliseconds.
Best Practices
- It's a good practice to set a reasonable timeout value for claiming pending messages in case a consumer crashes or is otherwise unable to acknowledge a message.
- When designing your application, keep in mind that you will need some way to handle failed messages. This could be through manual intervention, or you could design self-healing systems that use XCLAIM to automatically redistribute work to active consumers.
Common Mistakes
- A common mistake when using
XCLAIM
is not setting an appropriate min-idle-time. If you set this value too low, consumers might step on each other's toes by claiming messages that are still being processed by other consumers.
FAQs
Q1: What happens if a message is claimed by two different consumers?
A1: The message gets assigned to the consumer that issued the XCLAIM
command most recently. However, it’s considered good practice to avoid such situations as much as possible, because it can lead to duplicate processing of messages.
Q2: Can a consumer claim a message that has already been acknowledged?
A2: No, once a message has been acknowledged with XACK
, it cannot be claimed again. It is removed from the pending entries list.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
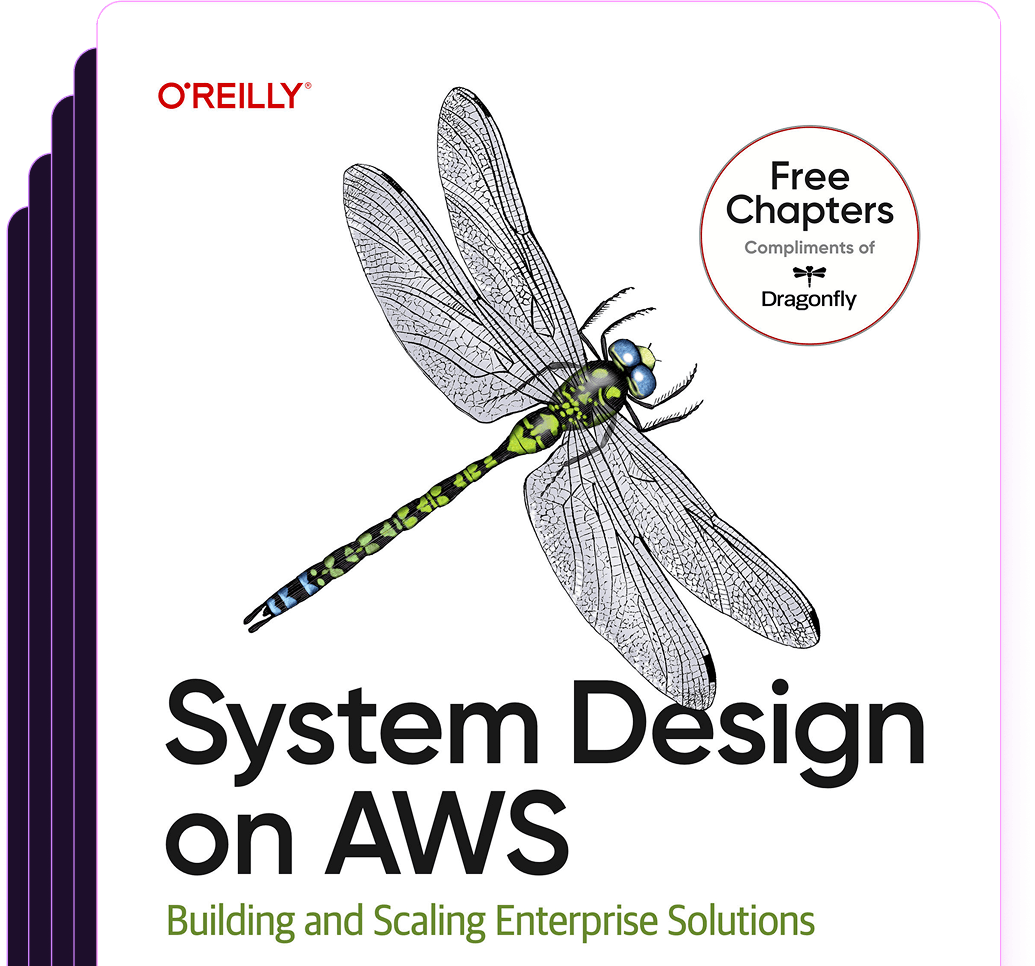
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost