Redis XREAD in Golang (Detailed Guide w/ Code Examples)
Use Case(s)
The XREAD
command in Redis is utilized for reading data from one or more streams, where data is stored as key-value pairs. This is commonly used in cases where you need to make your application respond to stream changes, similar to pub/sub pattern but with the ability to receive past messages.
Code Examples
Here's an example of using XREAD
in Go with the "go-redis" package:
package main
import (
"fmt"
"github.com/go-redis/redis/v8"
"golang.org/x/net/context"
)
func main() {
rdb := redis.NewClient(&redis.Options{
Addr: "localhost:6379",
Password: "",
DB: 0,
})
ctx := context.TODO()
// Reading stream 'mystream' starting from '$', which means the latest message
streams, err := rdb.XRead(ctx, &redis.XReadArgs{
Streams: []string{"mystream", "$"},
}).Result()
if err != nil {
panic(err)
}
for _, stream := range streams {
fmt.Println("Stream:", stream.Stream)
for _, message := range stream.Messages {
fmt.Println("ID:", message.ID)
for k, v := range message.Values {
fmt.Println(k, v)
}
}
}
}
In this example, we're creating a connection to a local Redis instance and reading from the 'mystream' stream. The $
character indicates that we want to read from the latest message. If there are any messages, they're printed to the console.
Best Practices
- In a production environment, handle
XREAD
errors appropriately instead of usingpanic(err)
. - If the application needs to continue from where it left off after a restart, store the last read ID somewhere persistent and provide it instead of
$
.
Common Mistakes
- Supplying an invalid stream name or ID to
XReadArgs
will causeXREAD
to fail. Always ensure the stream name and ID are correct. - Using
$
will give you only new messages. If you want to process old messages as well, use0
or the specific message ID.
FAQs
Q: Can I read from multiple streams with XREAD?
A: Yes, Redis's XREAD
command supports reading from multiple streams at once. You can specify multiple streams in the Streams
slice of XReadArgs
.
Q: What does the '$' character mean when reading from a stream in Redis?
A: When reading from a stream in Redis, the $
character represents the latest message in the stream. If you want to read from the start of the stream, you can use 0
instead.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
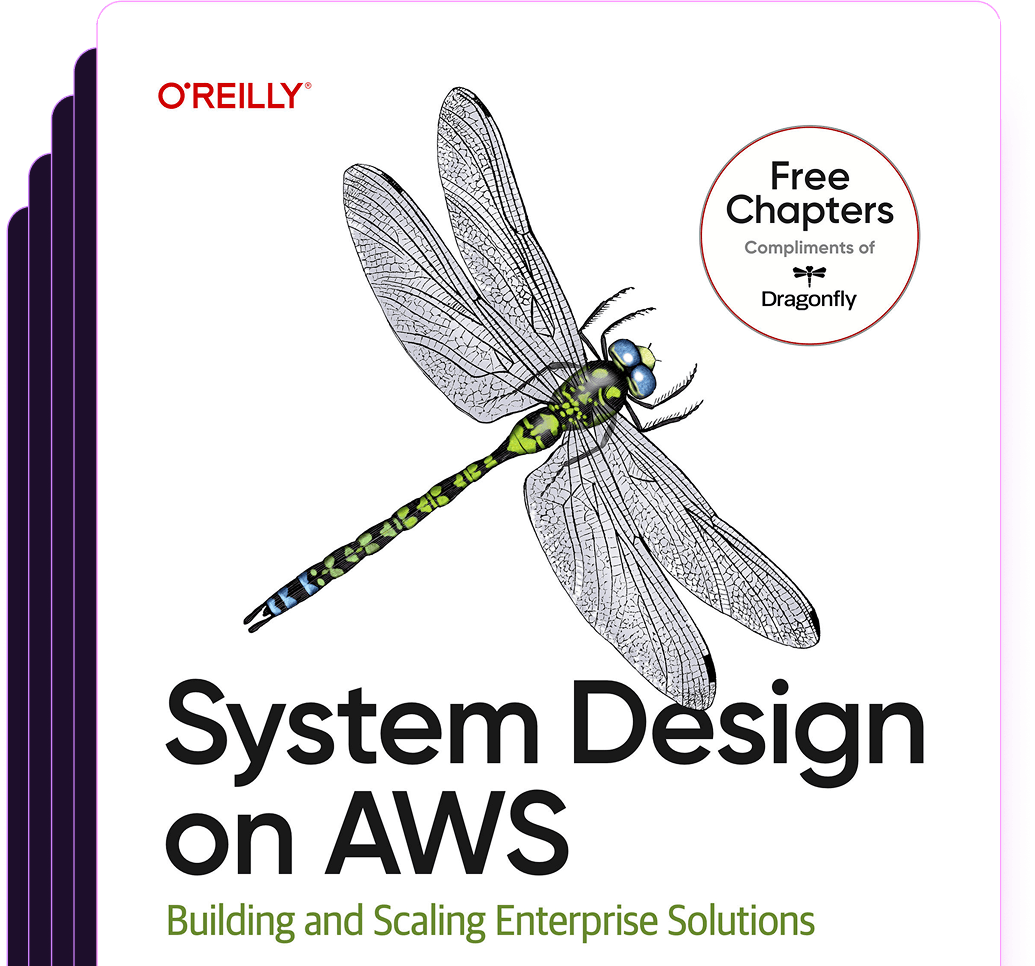
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost