Redis HINCRBY in Java (Detailed Guide w/ Code Examples)
Use Case(s)
The HINCRBY
command in Redis is used when you need to increment the integer value of a hash field by the given number. This operation is atomic, meaning it's safe to use in a concurrent environment. Some common uses for HINCRBY
include:
- Incrementing counters stored in a hash.
- Keeping track of user activities within a period (e.g., video plays, button clicks).
Code Examples
Let's turn to Jedis, a popular Java client for Redis.
- Incrementing a hash field
import redis.clients.jedis.Jedis;
public class Main {
public static void main(String[] args) {
// Connecting to Redis server on localhost
Jedis jedis = new Jedis("localhost");
// Increment the field 'views' in hash 'video:1234' by 1
jedis.hincrBy("video:1234", "views", 1);
jedis.close();
}
}
In this example, we connect to a local Redis instance and increase the count of a hypothetical 'views' field in a 'video:1234' hash by 1. If the 'views' field didn't exist before, it will be created.
Best Practices
- Error handling: Always check if the key and field exist before trying to increment them.
HINCRBY
assumes that the field is an integer and will return an error if the field contains non-integer values. - Type consistency: Keep your data types consistent. If a field is intended as a numeric counter, don't store string or other data type in it.
Common Mistakes
- Assuming the field is an integer:
HINCRBY
only works on integer fields. If the current field value is not an integer, Redis will return an error. - Ignoring concurrency: Even though
HINCRBY
is atomic and safe to use concurrently, other operations around it might not be. Consider using transactions if needed.
FAQs
Q: What happens if the field does not exist?
A: If the hash field does not exist, Redis will assume it's 0 and then perform the increment operation.
Q: What if the increment makes the value larger than the maximum integer size?
A: Redis allows for 64 bit integers. If an increment leads to a value beyond this, it will return an error because it can't store the result.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
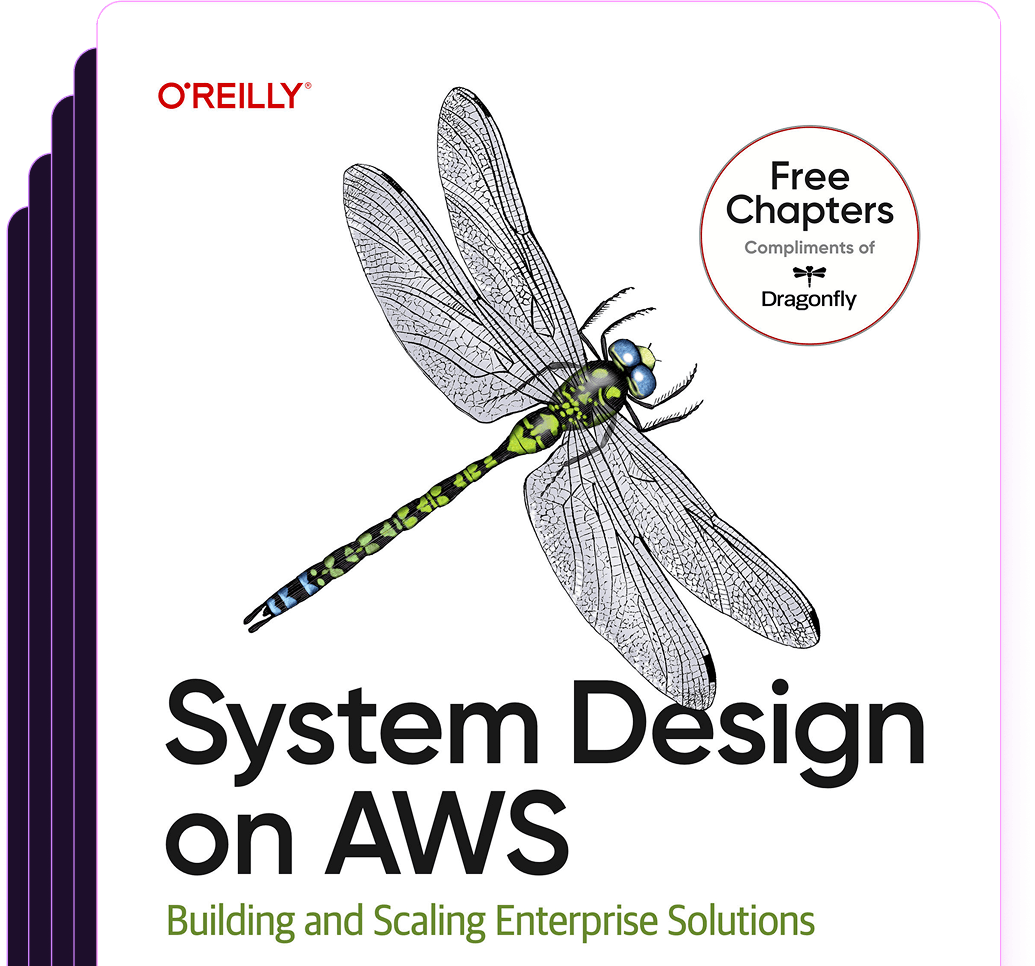
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost