Redis HSET in Java (Detailed Guide w/ Code Examples)
Use Case(s)
Redis HSET is commonly used when you need to store multiple related data points, such as attributes of an object, into a single key in your Redis database. This makes access and manipulation of these related data easier and more efficient, especially in cases where the fields are frequently retrieved together.
Code Examples
We use Jedis, a popular Redis client for Java.
Here's how to use HSET:
// import the Jedis class
import redis.clients.jedis.Jedis;
public class Main {
public static void main(String[] args){
// connect to redis server
Jedis jedis = new Jedis("localhost");
// use HSET command
jedis.hset("user:1", "name", "John Doe");
jedis.hset("user:1", "email", "john.doe@example.com");
}
}
In this example, we're creating a hash with the key user:1
and setting fields (name
and email
) with corresponding values.
Best Practices
- Consider using
hsetnx
if you want to ensure that a field does not get overwritten if it already exists. - Always close the connection to the Redis server when you're done.
Common Mistakes
- Be aware that by default, Jedis connects to localhost. You'll need to provide the host and port if your Redis server is located elsewhere.
- Remember that while Redis is powerful, it's not necessarily the ideal solution for all data storage problems. Use it judiciously and appropriately.
FAQs
Q: Can I set multiple fields at once? A: Yes, use the hmset
method to set multiple fields at once. It accepts a Map as an argument.
Q: What if the key already exists? A: If the key already exists, the HSET command will update the value of the field, not the entire hash.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
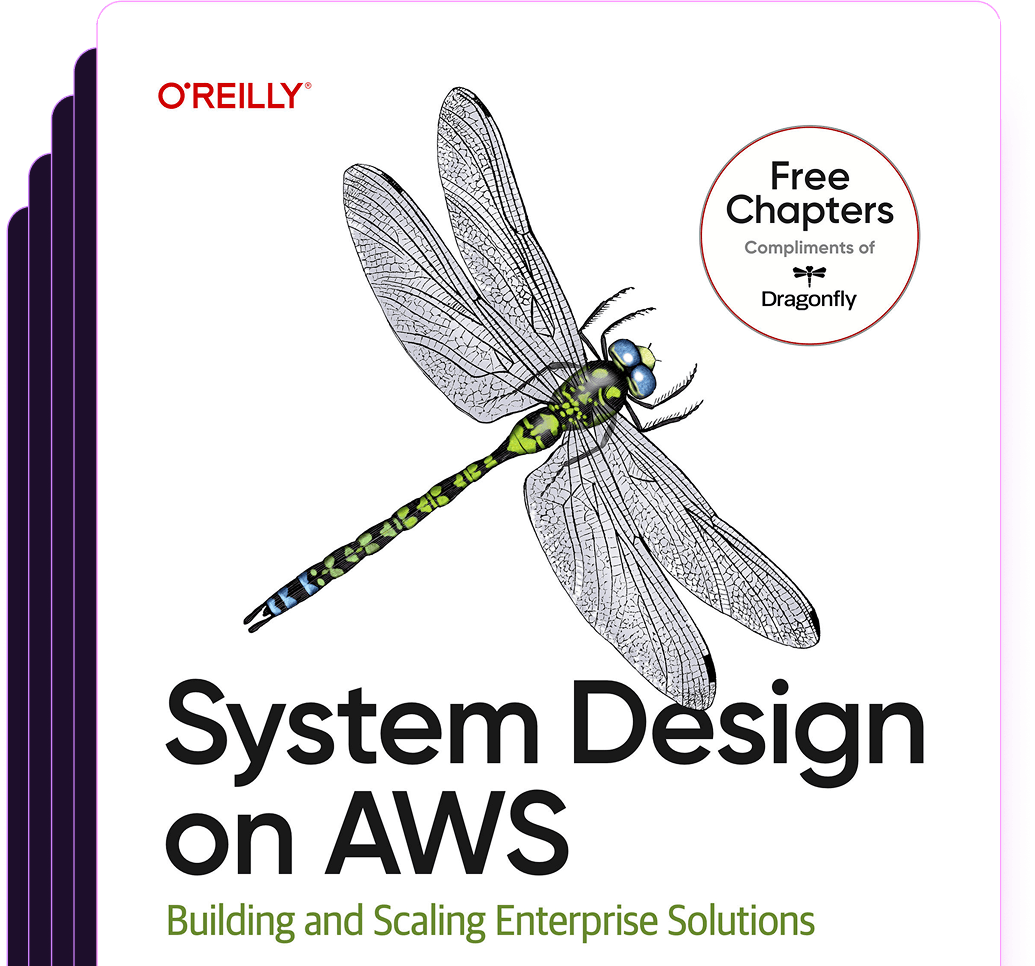
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost