Redis XREAD in Node.js (Detailed Guide w/ Code Examples)
Use Case(s)
The XREAD
command is used in Redis Streams when a client wants to consume data. It allows for both blocking and non-blocking reads from one or multiple streams. This is particularly useful when you need to process stream data in real-time, such as in a chat application, tracking user activity, or handling event-driven architectures.
Code Examples
Consider an application where we are using the Node Redis client library and want to read from a Redis Stream named "mystream".
- Blocking Read
const redis = require('redis');
const client = redis.createClient();
client.xread('BLOCK', 0, 'STREAMS', 'mystream', '$', function(err, stream) {
if (err) throw err;
console.log(stream);
});
In this example, we are using the BLOCK
option with a timeout of 0, indicating that it will wait indefinitely for new data. The dollar sign $
indicates that we want to read any new messages that arrive after initiating this command.
- Non-Blocking Read
const redis = require('redis');
const client = redis.createClient();
client.xread('STREAMS', 'mystream', '0', function(err, stream) {
if (err) throw err;
console.log(stream);
});
In this instance, we're performing a non-blocking read. The '0' indicates that we want to start reading from the beginning of the stream.
Best Practices
- When reading from streams, always track the last ID you've read up to so you can continue from there the next time.
- Use blocking reads (
XREAD BLOCK
) with caution. It's often better to implement polling with a pause instead of using indefinite blocking reads (BLOCK
0) that could potentially tie up a connection indefinitely.
Common Mistakes
- Misunderstanding the behaviors of blocking and non-blocking reads can lead to issues. Non-blocking reads will return immediately, even if there’s no data to read, whereas blocking reads will wait until there's data available or a timeout occurs.
- When using
XREAD
, be aware that it returns an array of results, not a single result. Even if you're reading from just one stream, you need to handle the returned data accordingly.
FAQs
Q: Can we read from multiple streams using XREAD in Node?
Yes, you can add more stream IDs to the 'STREAMS' option like so:
client.xread('STREAMS', 'mystream1', 'mystream2', 'lastID1','lastID2', function(err, stream) {
if (err) throw err;
console.log(stream);
});
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
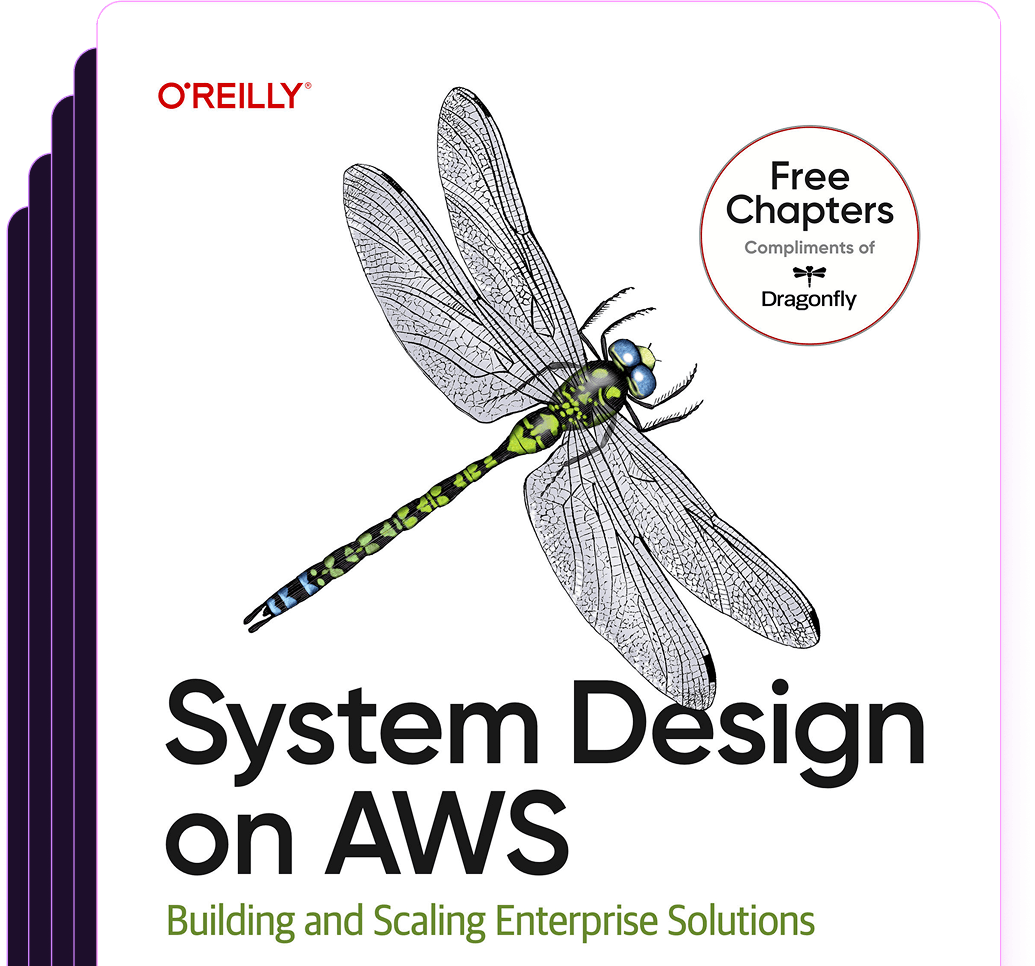
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost