Redis XREADGROUP in Node.js (Detailed Guide w/ Code Examples)
Use Case(s)
The XREADGROUP
command in Redis is used for consuming data from a Stream using the concept of consumer groups. This is particularly useful when you have multiple consumers and want to split the load among them, or if you want to ensure reliable message processing; if one consumer fails, another can pick up the workload.
Code Examples
Firstly, we need a Redis client in Node.js. You can install it via npm:
npm install redis
Next, create a simple Node.js application that uses the xreadgroup
command. Below example assumes that there's already a stream named 'mystream' and a consumer group 'mygroup'.
const redis = require('redis');
const client = redis.createClient();
client.xreadgroup(
'GROUP', 'mygroup', 'consumer1',
'STREAMS', 'mystream', '>',
function(err, reply) {
if (err) throw err;
console.log(reply);
});
client.quit();
In this code snippet, we're reading from mystream
as part of mygroup
with a consumer named consumer1
. The '>' character means that we want to read only messages that were not yet delivered to other consumers of the group.
Best Practices
- Always handle potential errors that may arise during a call to
xreadgroup
. - Consider using blocking reads with a timeout, which is more efficient than polling.
- Be mindful of the memory usage when using Redis Streams, especially if your use case involves storing large quantities of data.
Common Mistakes
- Not creating a consumer group before trying to read from it. You need to use the
XGROUP CREATE
command before usingXREADGROUP
. - Using same consumer name in different instances. Each instance should have a unique consumer name.
FAQs
1. What does the '>' mean in xreadgroup
command?
The '>' character means that we want to read only messages that were not yet delivered to other consumers of the group.
2. How can I ensure data isn't lost if a consumer fails while processing a message?
Redis provides functionality for this exact scenario. If a consumer fails to acknowledge a message, another consumer can claim and process it.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
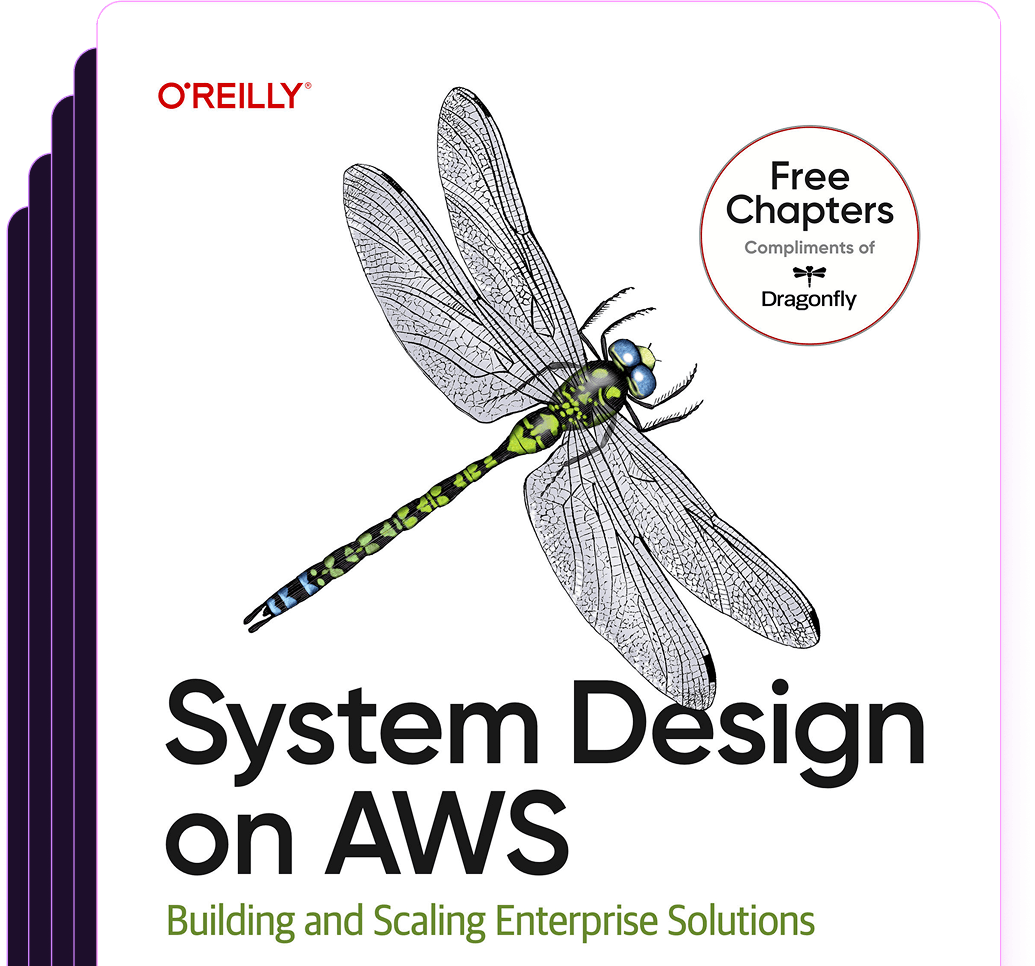
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost