Getting All Keys using PHP Redis (Detailed Guide w/ Code Examples)
Use Case(s)
PHP Redis keys
method is commonly used to retrieve all keys matching a certain pattern in a Redis database. This can be useful in several scenarios such as debugging, maintenance tasks, or when you need to manipulate set of data identified by similar key patterns.
Code Examples
Example 1: Get all keys
In this example, we will get all the keys in our Redis instance. The asterisk (*) is a wildcard that matches all keys.
$redis = new Redis();
$redis->connect('localhost', 6379);
$allKeys = $redis->keys('*');
print_r($allKeys);
Example 2: Get keys with a specific pattern
In this case, we're looking for keys that start with 'user':
$redis = new Redis();
$redis->connect('localhost', 6379);
$userKeys = $redis->keys('user*');
print_r($userKeys);
The 'user*' pattern will match any keys that start with 'user'.
Best Practices
- Be careful when using the
keys
command in a production environment, especially with large databases and broad patterns, as it may degrade performance. - If possible, use the
SCAN
command instead ofKEYS
. SCAN provides a cursor-based iterator which is more efficient and doesn't block the server.
Common Mistakes
- Using KEYS in production environments: The
KEYS
command can negatively impact performance on large databases, so it's not recommended for production environments. Instead, consider usingSCAN
or maintain an index of your keys in a separate data structure. - Ignoring return types: The
KEYS
command returns an array of strings representing the matching keys orFALSE
if there are no keys. Always ensure to handle these return types correctly in your application.
FAQs
Q: Can I use patterns with the Redis keys
method?
A: Yes, you can use patterns when retrieving keys. For example, 'user*' will match any keys that start with 'user'.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
- PHP Redis: Get All Keys Matching Pattern
- PHP Redis: Get All Keys Starting With
- PHP Redis - Get Hash Values at a Key
- Getting Memory Stats in PHP Redis
- Checking if a Key Exists in Redis using PHP
- Getting Number of Subscribers in Redis with PHP
- Getting a String Key using PHP and Redis
- PHP Redis: Get Current Database
- Retrieving Multiple Keys in PHP Using Redis
- PHP Redis: Get Master Status
- Getting Default TTL with PHP Redis
- Getting Current Version of Redis in PHP
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
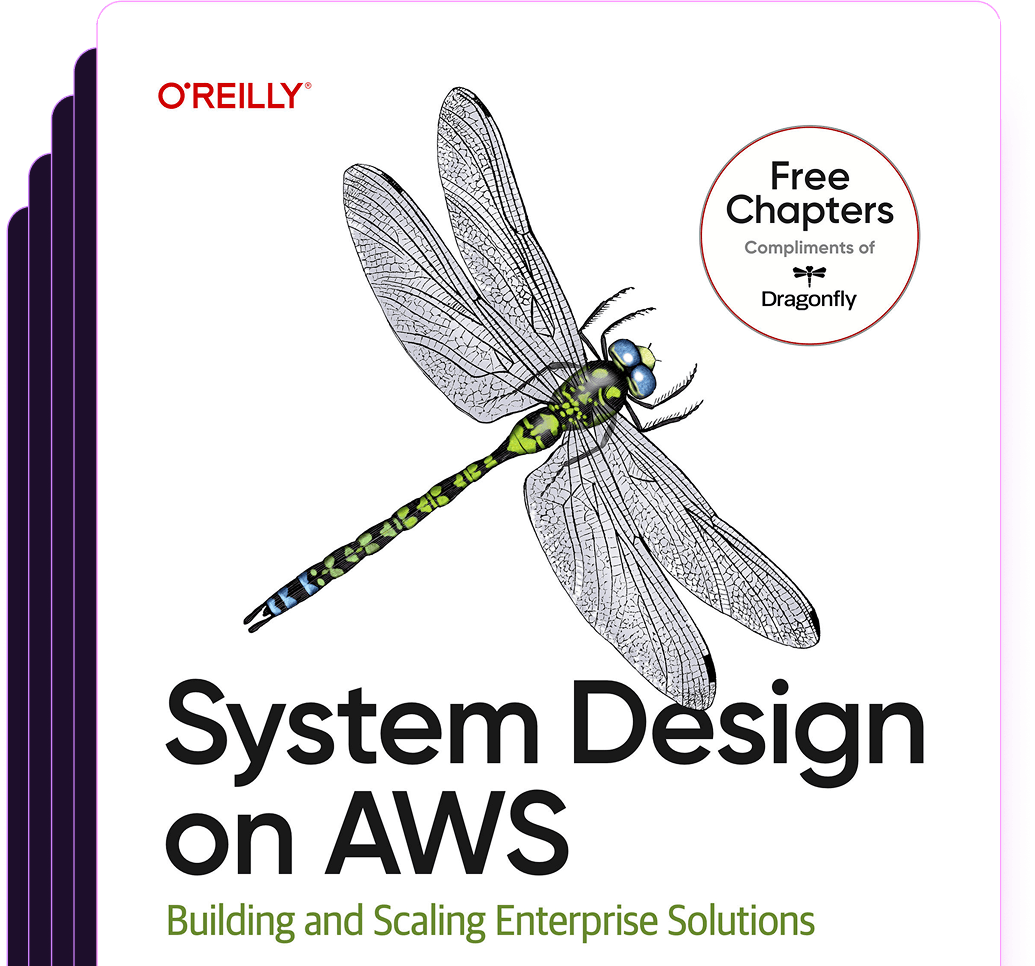
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost