Checking if a Key Exists in Redis using PHP (Detailed Guide w/ Code Examples)
Use Case(s)
In Redis, checking if a key exists is quite commonly done while retrieving values from Redis cache. If your application is written in PHP, you might need to check whether a key exists before trying to retrieve its value to avoid possible errors or exceptions.
Code Examples
Let's assume you're already connected to your Redis instance. Here's an example demonstrating how to check if a key exists.
$redis = new Redis();
$redis->connect('127.0.0.1', 6379);
$key = 'testKey';
if($redis->exists($key)) {
echo "Key exists";
} else {
echo "Key does not exist";
}
In this code, we're first creating a new instance of the Redis class and connecting to the local Redis server. We then use the exists
method to check if the key 'testKey'
exists. If it does, we print 'Key exists', else we print 'Key does not exist'.
Best Practices
- Always handle potential connection errors when working with Redis. Your application should be able to respond appropriately if the Redis server is down or unreachable.
- Avoid frequent calls to
exists
. Instead consider using Redis transactions (MULTI/EXEC commands) or Lua scripting for complex atomic operations.
Common Mistakes
- Don't forget that the
exists
function returns the number of keys existing among those specified as arguments. So always checking it in boolean context might not give the expected results if you are checking multiple keys at once. - Requesting a non-existent key from Redis will not provide an error; it will return
null
. Therefore, always check if a key exists before trying to get its value.
FAQs
Q: Can I use wildcards with the exists
function?
A: No, the exists
function doesn't support patterns or wildcards. If you need to check for keys following a certain pattern, consider using the keys
command first, and then check for existence for each key.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
- PHP Redis: Get All Keys Matching Pattern
- PHP Redis: Get All Keys Starting With
- PHP Redis: Get Current Memory Usage
- PHP Redis: Getting Key Type
- PHP Redis - Get Hash Values at a Key
- PHP Redis: Getting All Databases
- Redis Get All Hash Keys in PHP
- Getting Memory Stats in PHP Redis
- Getting Redis Configuration Settings in PHP
- Getting Redis Key by Value in PHP
- Getting Number of Subscribers in Redis with PHP
- Retrieving Redis Keys Without TTL in PHP
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
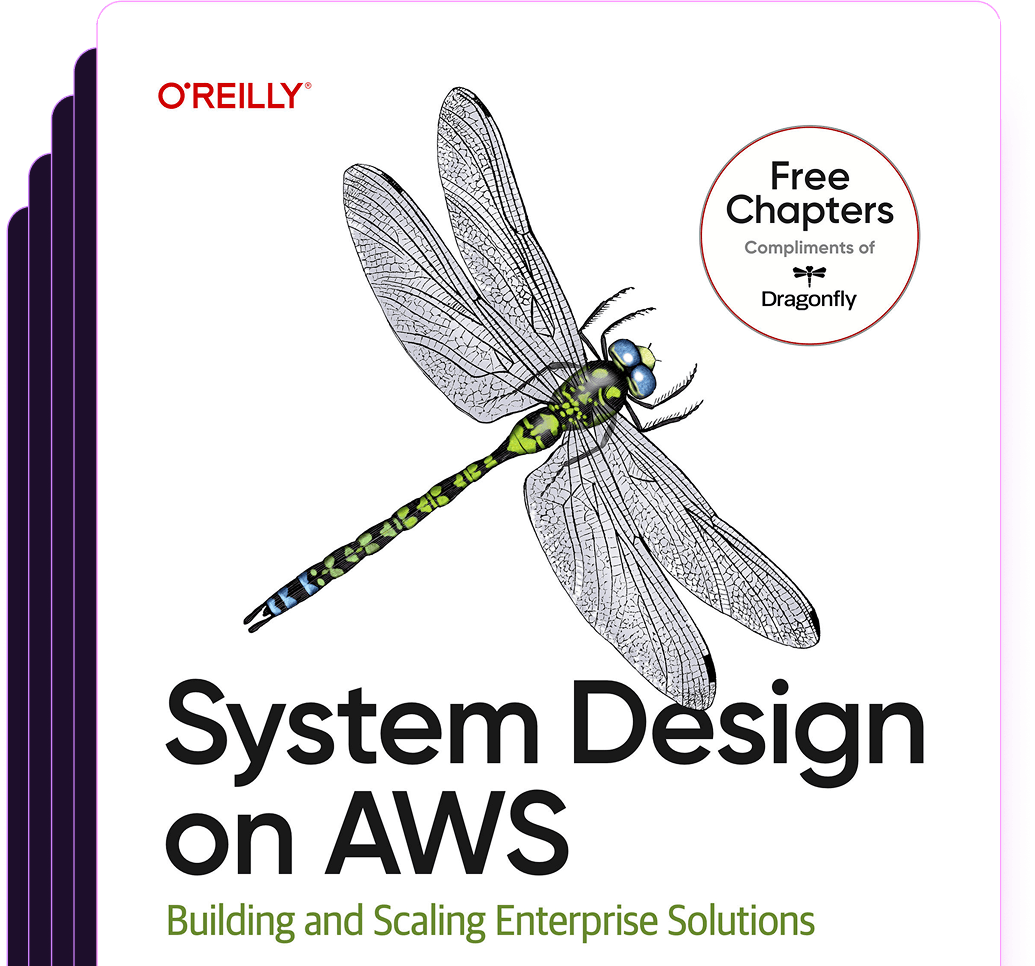
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost