PHP Redis: Get All Keys and Values (Detailed Guide w/ Code Examples)
Use Case(s)
In PHP, using Redis to store data can be extremely beneficial for caching, session storage, and more. Often, you may need to retrieve all keys and their associated values from a Redis database for various reasons such as debugging, data analysis, or system monitoring.
Code Examples
- Get all keys: CODE_BLOCK_PLACEHOLDER_0
In this example, we first establish a connection to the Redis server. The keys('*')
function retrieves all keys from the Redis server.
- Get all keys and values: CODE_BLOCK_PLACEHOLDER_1
After getting all keys, we loop through each key and use the get()
function to retrieve its corresponding value.
Best Practices
- Avoid using the
keys('*')
command in a production environment because it can degrade performance. Use it sparingly or during maintenance periods. - Use more specific patterns with the
keys(pattern)
function where possible to avoid unnecessary hits to the database.
Common Mistakes
- Not handling the case where the database is empty, which can lead to errors. Always check if keys exist before trying to get values.
FAQs
1. How can I filter keys in Redis using PHP? In PHP, you can filter keys in Redis by passing a pattern to the keys(pattern)
function.
2. What happens when I try to get a value of a key that doesn't exist? If you try to get a value of a key that does not exist, Redis will return NULL
.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
- PHP Redis: Get All Keys Matching Pattern
- PHP Redis: Get All Keys Starting With
- PHP Redis: Get Current Memory Usage
- PHP Redis: Getting Key Type
- PHP Redis - Get Hash Values at a Key
- PHP Redis: Getting All Databases
- Redis Get All Hash Keys in PHP
- Getting Memory Stats in PHP Redis
- Checking if a Key Exists in Redis using PHP
- Getting Redis Configuration Settings in PHP
- Getting Redis Key by Value in PHP
- Getting Number of Subscribers in Redis with PHP
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
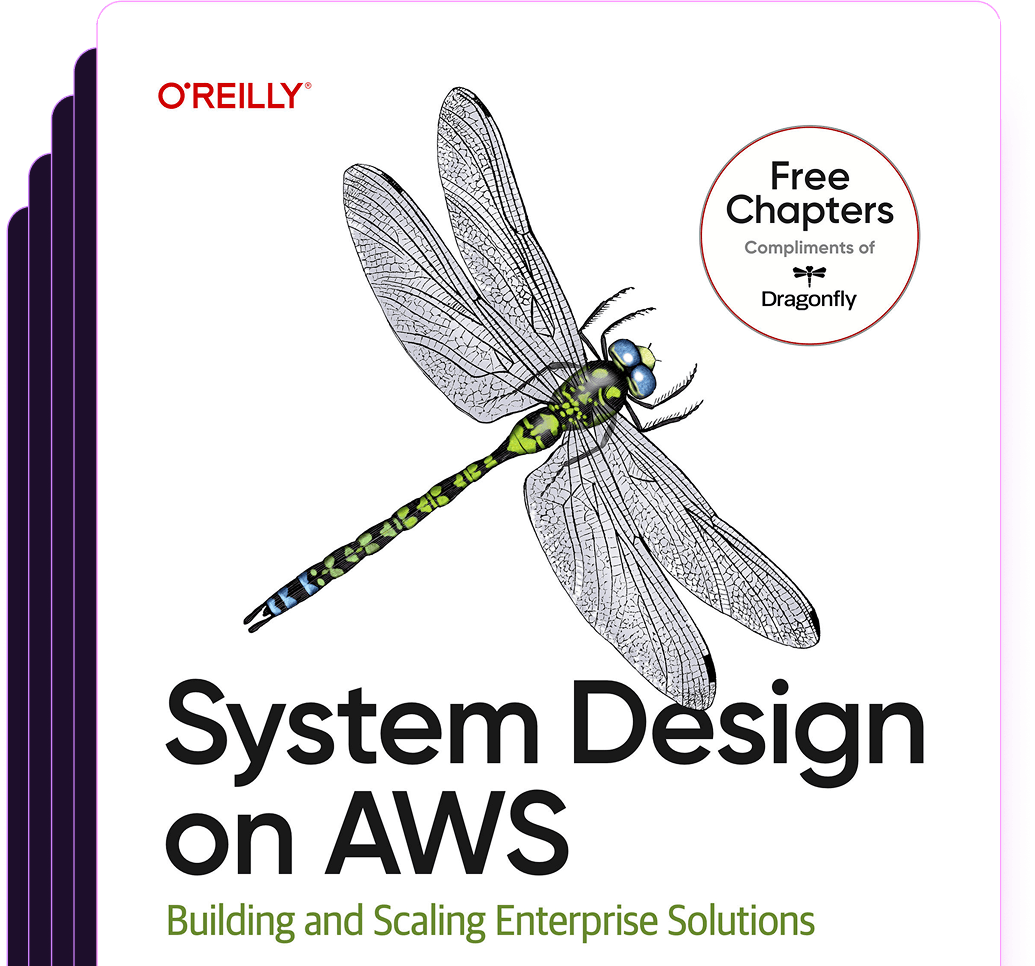
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost