Getting JSON Value from Redis using PHP (Detailed Guide w/ Code Examples)
Use Case(s)
In a typical web application, Redis can be used for caching data to improve performance. This becomes especially useful when you store JSON data in Redis and retrieve it using PHP. This is common in scenarios where the data stored is structured and complex, such as user profiles, configuration settings etc.
Code Examples
- Storing and retrieving JSON data:
<?php
$redis = new Redis();
$redis->connect('127.0.0.1', 6379);
$data = ['name' => 'John Doe', 'age' => 30];
$redis->set('user:1', json_encode($data));
$jsonValue = $redis->get('user:1');
$data = json_decode($jsonValue, true);
echo $data['name']; // Output: John Doe
?>
This code first connects to the Redis server, then encodes an associative array into a JSON string using json_encode()
and stores it in Redis. It then retrieves this data using the get
method, decodes it back into an array using json_decode()
, and finally prints the name.
Best Practices
- Always check for the existence of a key before getting its value to avoid null values and potential errors in your application. You can use the
exists
command for this purpose. - Use appropriate error handling mechanisms while dealing with Redis operations to ensure your application's robustness.
Common Mistakes
- Not properly encoding and decoding JSON data: Make sure to use
json_encode()
when storing data andjson_decode()
when retrieving it. - Forgetting that Redis stores data as strings: Always remember to convert your data to the appropriate format before using it.
FAQs
Q: What happens if I try to get a key that doesn't exist in Redis? A: If you try to get a key that doesn't exist, Redis will return null
.
Q: How can I handle JSON errors when using json_decode()
in PHP? A: You can use the json_last_error_msg()
function to get the error message from the last json_encode()
or json_decode()
call.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
- PHP Redis: Get All Keys Matching Pattern
- PHP Redis: Get All Keys Starting With
- PHP Redis - Get Hash Values at a Key
- Getting Memory Stats in PHP Redis
- Checking if a Key Exists in Redis using PHP
- Getting Number of Subscribers in Redis with PHP
- Getting a String Key using PHP and Redis
- PHP Redis: Get Current Database
- Retrieving Multiple Keys in PHP Using Redis
- PHP Redis: Get Master Status
- Getting Default TTL with PHP Redis
- Getting Current Version of Redis in PHP
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
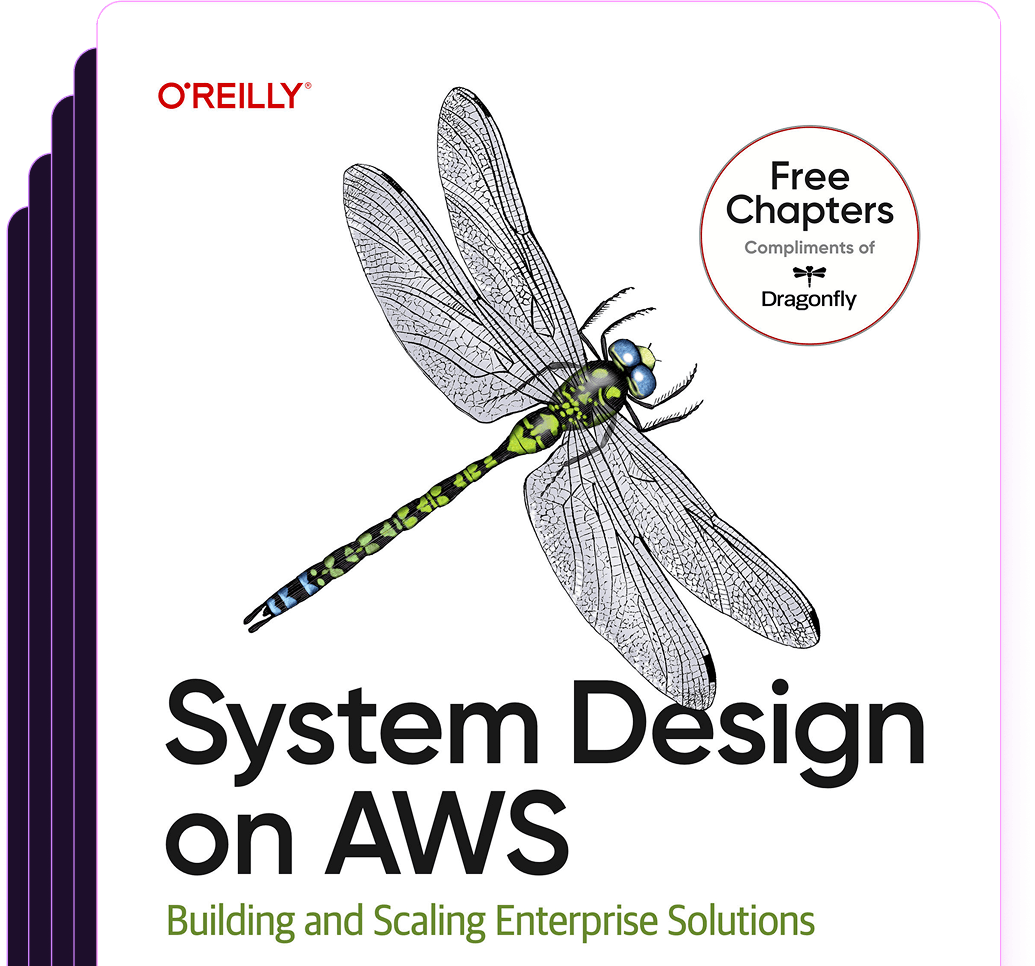
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost