Retrieving a Redis Key Using PHP (Detailed Guide w/ Code Examples)
Use Case(s)
One common use case for the php redis get key
operation is when you need to retrieve the value of a specific key from a Redis database in a PHP application. This might be necessary in situations like caching, where data that was temporarily stored in Redis needs to be fetched for quicker access.
Code Examples
Here's an example of how to use php redis get key
:
<?php
$redis = new Redis();
$redis->connect('127.0.0.1', 6379);
$key = 'myKey';
$value = $redis->get($key);
echo $value;
?>
In this code, we first create a new instance of the Redis class, then connect to a Redis server running on localhost at port 6379. Then we specify a key (myKey
), and use the get()
method to retrieve its value. The value is then printed out.
Best Practices
Always check if the key exists before trying to get its value to avoid null values. You can do this using the exists()
function. Here's how:
<?php
$redis = new Redis();
$redis->connect('127.0.0.1', 6379);
$key = 'myKey';
if ($redis->exists($key)) {
$value = $redis->get($key);
echo $value;
} else {
echo "Key does not exist";
}
?>
This way, you handle potential errors gracefully by checking if the key exists before attempting to retrieve its value.
Common Mistakes
One common mistake is not handling the situation where the specified key does not exist in the Redis store. In this case, the get()
function will return false
. It's a good practice to always check if the key exists before retrieving it.
FAQs
Q: What happens if the key does not exist in the Redis database?
A: The get()
function will return false
if the key does not exist.
Q: Can I connect to a Redis database on a different host or port?
A: Yes, you can. You just need to use the correct IP address and port number when calling the connect()
method.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
- PHP Redis: Get All Keys Matching Pattern
- PHP Redis: Get All Keys Starting With
- PHP Redis - Get Hash Values at a Key
- Getting Memory Stats in PHP Redis
- Checking if a Key Exists in Redis using PHP
- Getting Number of Subscribers in Redis with PHP
- Getting a String Key using PHP and Redis
- PHP Redis: Get Current Database
- Retrieving Multiple Keys in PHP Using Redis
- PHP Redis: Get Master Status
- Getting Default TTL with PHP Redis
- Getting Current Version of Redis in PHP
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
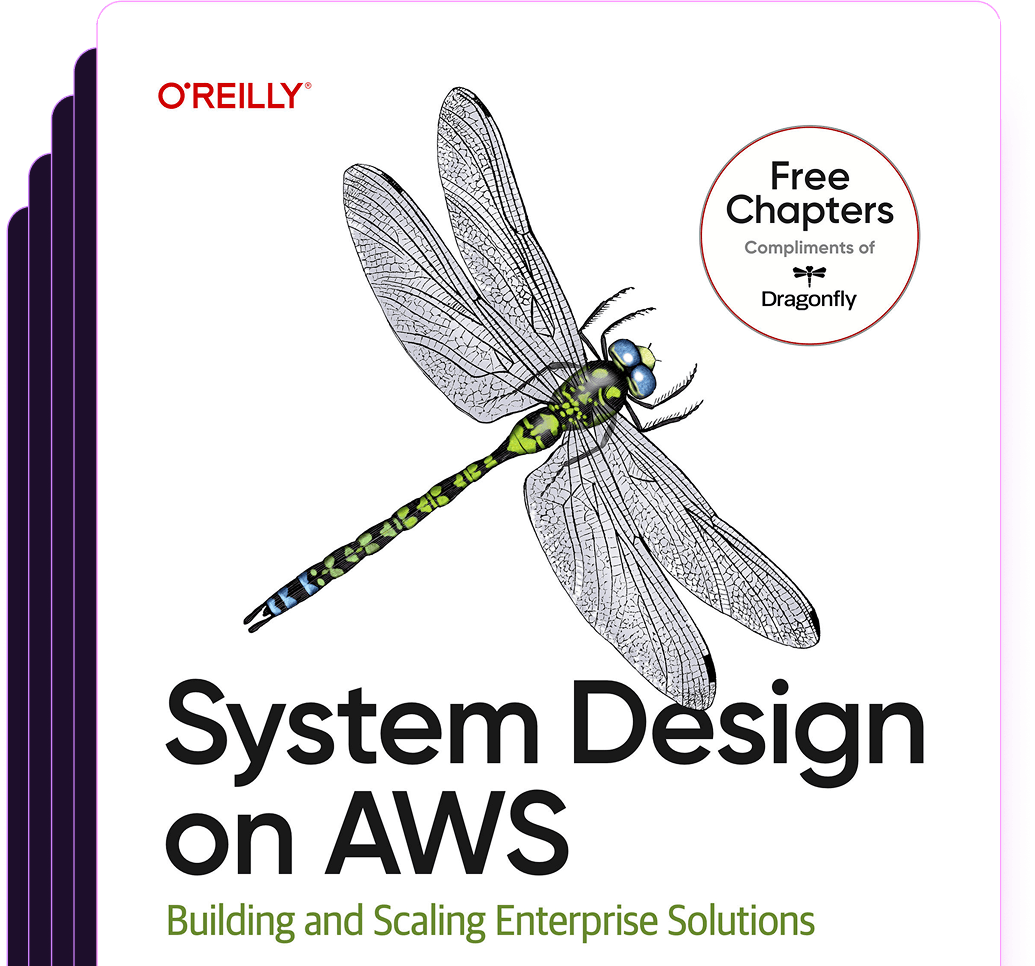
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost