Redis HRANDFIELD in PHP (Detailed Guide w/ Code Examples)
Use Case(s)
HRANDFIELD
is a Redis command used to get one or more random fields from a hash. It is useful for scenarios where you want to retrieve random elements without knowing the keys beforehand. This can be beneficial in applications such as showing random user profiles, selecting random product recommendations, etc.
Code Examples
Example 1: Fetching a Single Random Field
$redis = new Redis();
$redis->connect('127.0.0.1', 6379);
$field = $redis->hRandField('myhash');
echo "Random field: $field";
This code connects to Redis and retrieves a single random field from the hash named 'myhash'.
Example 2: Fetching Multiple Random Fields
$redis = new Redis();
$redis->connect('127.0.0.1', 6379);
$fields = $redis->hRandField('myhash', 3);
print_r($fields);
This example will fetch three random fields from 'myhash'. The second argument specifies the count of fields to retrieve.
Example 3: Fetching Multiple Random Fields with Values
$redis = new Redis();
$redis->connect('127.0.0.1', 6379);
$fieldsWithValues = $redis->hRandField('myhash', 3, true);
print_r($fieldsWithValues);
In this case, we're fetching three random fields along with their values from 'myhash'. Setting the third argument to true
returns an associative array of fields and their corresponding values.
Best Practices
- Use appropriate error handling when establishing a connection to the Redis server.
- Ensure that the Redis extension is properly installed and available in your PHP environment.
- Be careful with the number of fields you request; asking for more fields than are present in the hash could lead to performance issues.
Common Mistakes
- Forgetting to check if the hash key exists before attempting to retrieve random fields can lead to null or unexpected results.
- Not accounting for the possibility of receiving duplicate fields when requesting multiple random fields without values.
FAQs
Q: What happens if hRandField
is called on a non-existent key? A: If the hash doesn't exist, hRandField
will return FALSE
.
Q: Can hRandField
return the same field more than once when asking for multiple fields? A: Yes, if you are not retrieving the values (setting the third parameter to false
), you may get duplicates. To avoid this, either fetch fields with values or handle deduplication in your PHP code.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
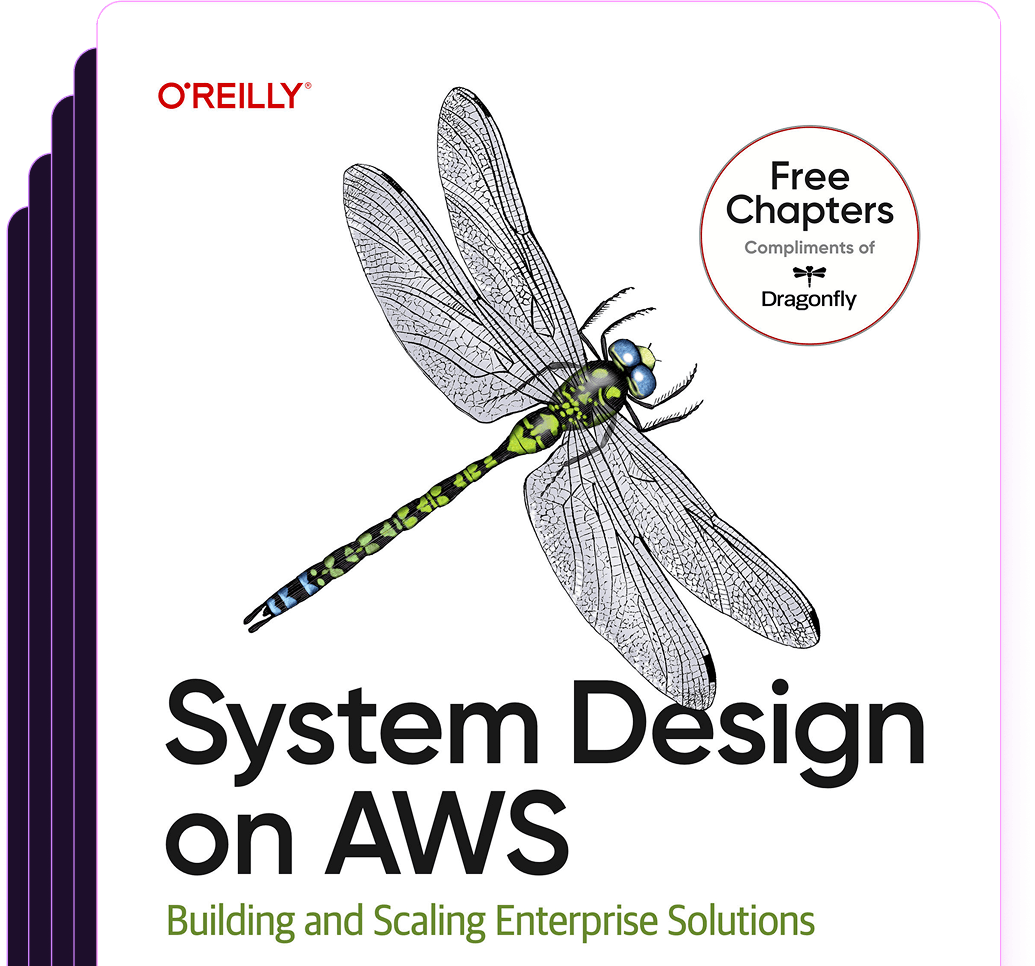
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost