Redis Sorted Set: Expire Key (Detailed Guide w/ Code Examples)
Use Case(s)
Setting an expiration time for keys in Redis is crucial for managing memory efficiently and ensuring that outdated data is automatically removed. This is particularly useful in scenarios such as caching, session management, and implementing TTL (Time-To-Live) features for dynamic content.
Code Examples
To set an expiration for a key in a Redis sorted set, you typically use the EXPIRE
or PEXPIRE
command. Below are examples in Python, Node.js, and Golang:
Python
Using the redis-py
library: CODE_BLOCK_PLACEHOLDER_0
Node.js
Using the ioredis
library: CODE_BLOCK_PLACEHOLDER_1
Golang
Using the go-redis
library: CODE_BLOCK_PLACEHOLDER_2
Best Practices
- Use expiration settings to manage memory usage effectively, especially for cache-like structures.
- Monitor the TTL values to ensure that critical data does not expire unexpectedly.
- Combine
EXPIRE
with persistence mechanisms like RDB or AOF to avoid data loss on restarts.
Common Mistakes
- Setting an expiration on the wrong key or forgetting to set it altogether.
- Assuming that expired keys will be immediately removed; they are lazily deleted when accessed or by the Redis internal process.
FAQs
Q: Can I update the expiration time of a key? A: Yes, calling EXPIRE
again on the same key updates its expiration time.
Q: What happens when a key expires? A: The key will be automatically deleted from the database once it reaches its expiration time.
Q: Is there a way to persistently store expired keys? A: By default, expired keys are removed and not stored. However, you can use events to log or take action when keys expire.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
- Redis Sorted Set: Get Highest Score
- Redis Sorted Set: Create
- Redis Sorted Set: Get by Key
- Redis Sorted Set: Limit Size
- Redis Sorted Set: Same Score
- Redis Sorted Set: Sorting by Multiple Fields
- Redis Sorted Set TTL
- Redis Sorted Set: Sort by Date
- Redis Sorted Set: Check Exists
- Redis Sorted Set: Remove by Score
- Redis Sorted Set: Rate Limit
- Redis Sorted Set: Custom Order
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
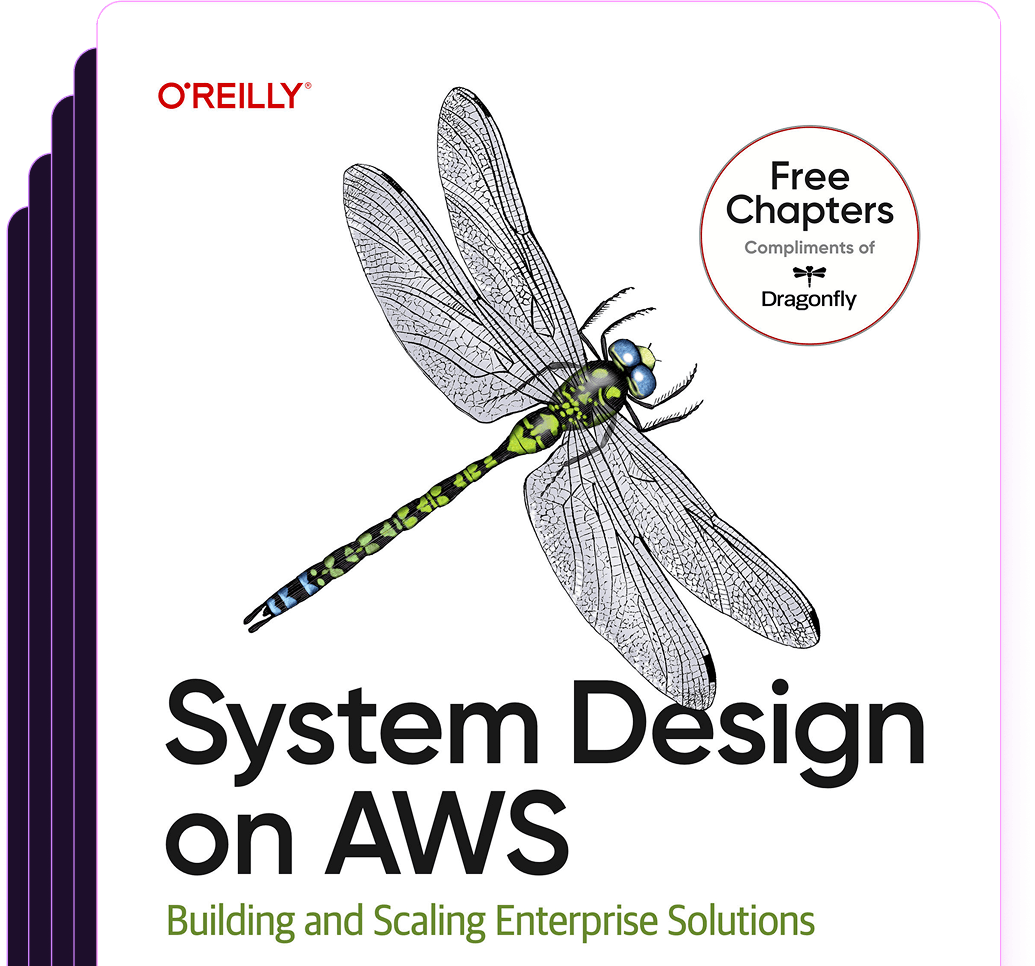
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost