Redis Sorted Set: Sorting by Multiple Fields (Detailed Guide w/ Code Examples)
Use Case(s)
- Ranking entities based on multiple criteria, such as user scores in a game (e.g., speed, accuracy).
- Creating leaderboards where multiple attributes determine position.
- Implementing complex ordering for items based on several fields.
Code Examples
Brief Explanation
Sorting by multiple fields with Redis sorted sets involves creating compound scores or using Lua scripts to manage multiple attributes. Here are examples in Python, Node.js, and Golang.
Python Example
import redis
# Connecting to Redis
r = redis.StrictRedis(host='localhost', port=6379, db=0)
# Add members with compound scores
r.zadd('leaderboard', {'user:1': 1000 + 0.5, 'user:2': 900 + 0.75})
# Get the sorted set
sorted_set = r.zrange('leaderboard', 0, -1, withscores=True)
print(sorted_set)
Node.js Example
const redis = require('redis');
const client = redis.createClient();
client.on('connect', function() {
console.log('Connected to Redis');
});
// Add members with compound scores
client.zadd('leaderboard', 1000 + 0.5, 'user:1', 900 + 0.75, 'user:2');
// Get the sorted set
client.zrange('leaderboard', 0, -1, 'WITHSCORES', (err, response) => {
if (err) throw err;
console.log(response);
});
Golang Example
package main
import (
"fmt"
"github.com/go-redis/redis/v8"
"context"
)
func main() {
ctx := context.Background()
client := redis.NewClient(&redis.Options{
Addr: "localhost:6379",
})
// Add members with compound scores
client.ZAdd(ctx, "leaderboard", &redis.Z{Score: 1000 + 0.5, Member: "user:1"}, &redis.Z{Score: 900 + 0.75, Member: "user:2"})
// Get the sorted set
result, _ := client.ZRangeWithScores(ctx, "leaderboard", 0, -1).Result()
fmt.Println(result)
}
Best Practices
- Ensure scores are generated consistently to avoid unintended overlaps.
- Use Lua scripts for more atomic and complex operations involving multiple fields.
- Regularly clean up and maintain your sorted sets to avoid performance degradation.
Common Mistakes
- Not normalizing fields before combining them into a single score can lead to incorrect orderings.
- Forgetting to handle floating point precision issues when combining multiple fields into one score.
- Neglecting the use of transactions or Lua scripts for multi-step operations, which could lead to race conditions.
FAQs
Q: Can I sort by multiple fields directly in Redis? A: Direct sorting by multiple fields isn't supported natively, but you can combine scores or use Lua scripts to achieve similar results.
Q: How do I ensure uniqueness when combining multiple fields? A: Normalize your fields to a consistent range and use a formula that effectively combines them without overlap.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
- Redis Sorted Set: Get Highest Score
- Redis Sorted Set: Create
- Redis Sorted Set: Get by Key
- Redis Sorted Set: Limit Size
- Redis Sorted Set: Same Score
- Redis Sorted Set TTL
- Redis Sorted Set: Sort by Date
- Redis Sorted Set: Expire Key
- Redis Sorted Set: Check Exists
- Redis Sorted Set: Remove by Score
- Redis Sorted Set: Rate Limit
- Redis Sorted Set: Custom Order
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
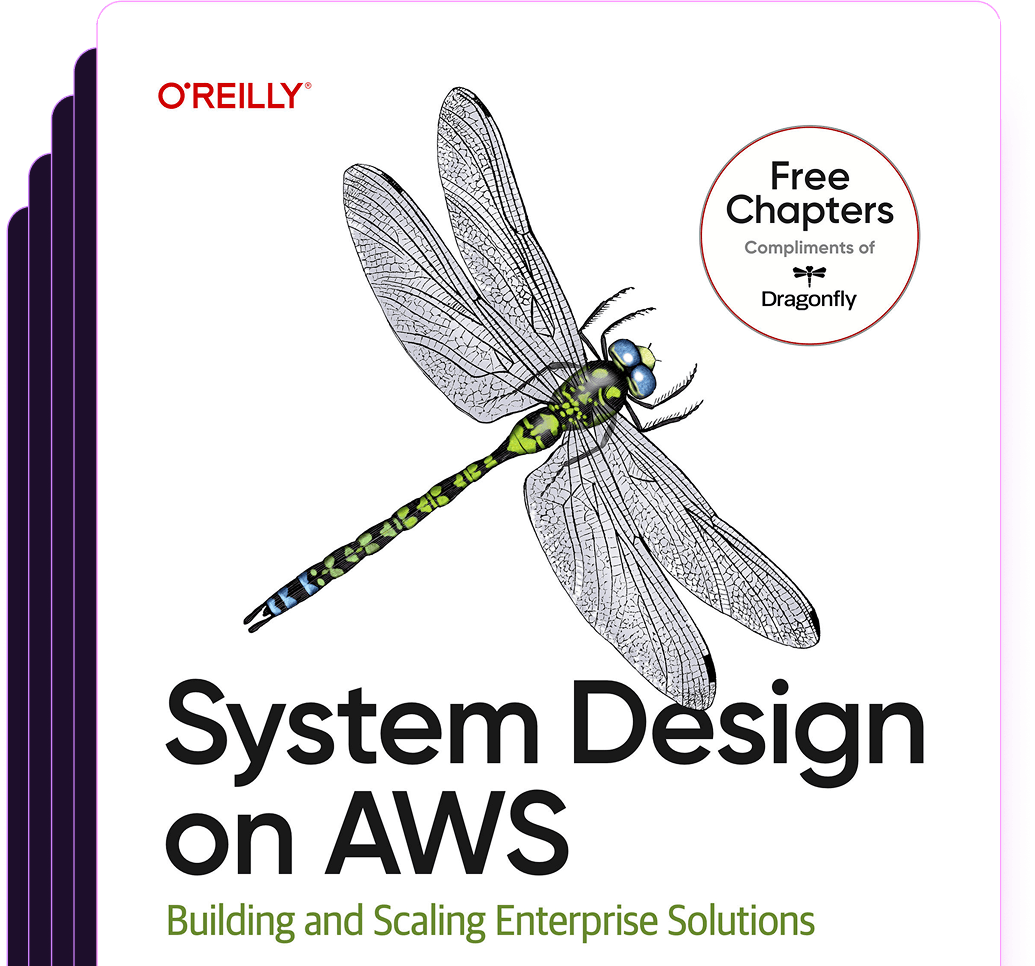
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost