Redis Get All Keys in Ruby (Detailed Guide w/ Code Examples)
Use Case(s)
Getting all keys from a Redis database is useful when you need to inspect the data, perform operations on bulk keys, or debug the system. It's often used during application development and debugging, but should be used with caution in production as it can affect performance.
Code Examples
In Ruby, you can use the keys
method from the redis
gem to get all keys. Here's an example:
require 'redis'
redis = Redis.new
keys = redis.keys('*')
puts keys
This code connects to the Redis server, fetches all keys using the wildcard character (*
), and prints them. The keys('*')
command matches all keys.
In case you want to fetch keys with a specific pattern, you can do so by providing that pattern to the keys
method. For example, to get all keys that start with 'user', you can write:
require 'redis'
redis = Redis.new
keys = redis.keys('user*')
puts keys
Best Practices
Although the KEYS
command can be very useful for development, it’s not recommended for production environments. This command can degrade performance, because it needs to look at every key in the database. For large databases, consider using the SCAN
command instead, which allows you to iteratively retrieve keys without blocking the database.
Common Mistakes
One common mistake is using KEYS
in production environments, which can block the Redis instance while it performs the operation. This might lead to performance issues if the database is large.
Another mistake is not handling potential exceptions when connecting to the Redis server. Always make sure you handle these cases in your code.
FAQs
1. Can I use the KEYS
command in production environments?
While technically possible, it's not recommended due to potential performance degradation. Use the SCAN
command instead.
2. How can I fetch keys according to a specific pattern?
You can provide a pattern to the keys
method. For example, redis.keys('user*')
retrieves all keys starting with 'user'.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
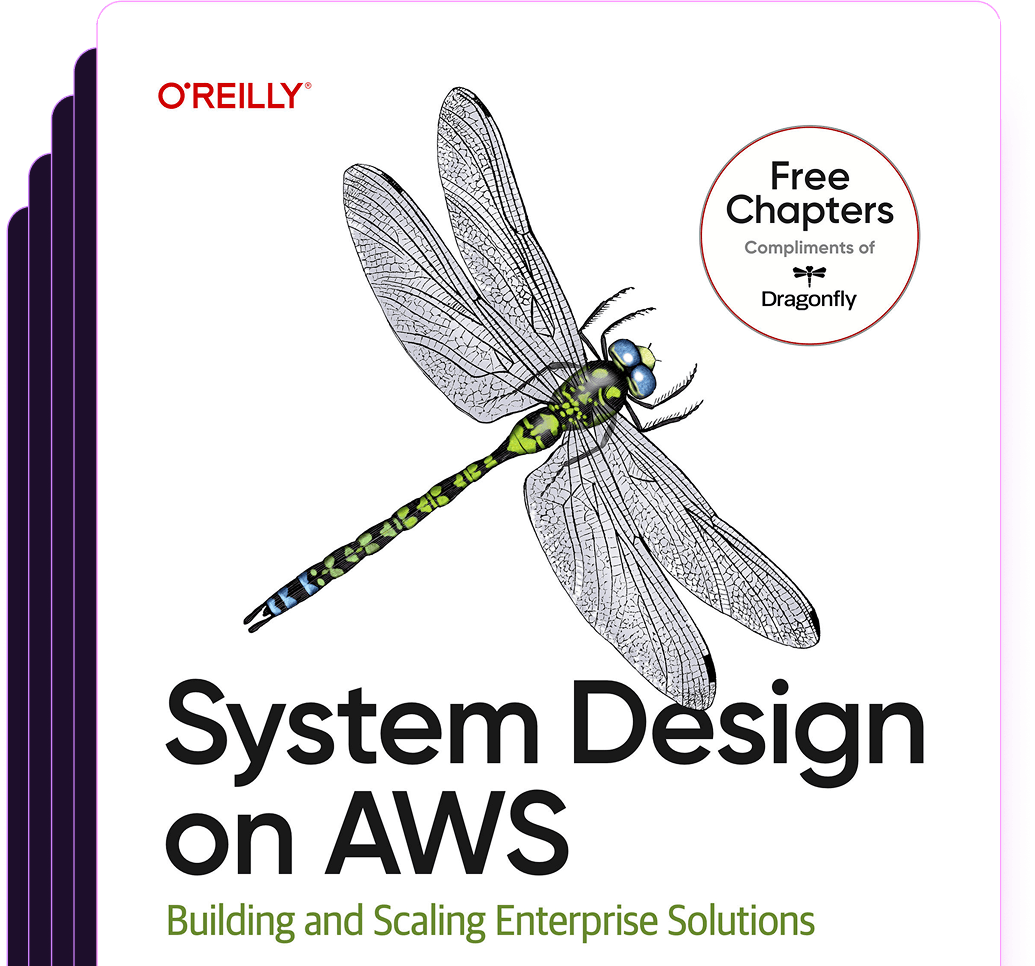
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost