Getting All Redis Keys Starting With a Prefix in Ruby (Detailed Guide w/ Code Examples)
Use Case(s)
In a Ruby application using Redis as a data store, you might have stored data with keys following certain naming conventions or patterns. For example, you may have prefixed user-related data with 'user:', session-related data with 'session:', and so on. In such cases, you may need to fetch all keys starting with a particular prefix.
Code Examples
To get all keys starting with a specific string, Redis provides the KEYS
command which accepts a pattern. Here's how you can use it with the redis-rb
gem in Ruby:
require 'redis'
redis = Redis.new(host: 'localhost', port: 6379)
keys = redis.keys('user:*')
puts keys
In this example, we're connecting to a local Redis instance and using the keys
method with a pattern 'user:' to get all keys that start with 'user:'. The '' is a wildcard character that matches any sequence of characters.
Best Practices
While the KEYS command can be incredibly useful, it’s important to note that it may affect performance when run against large databases because it's a blocking operation. Instead, consider using the SCAN command for production environments if you need to retrieve keys matching a pattern. The SCAN command iteratively scans the database and returns a cursor that you can pass back to iterate over the next batch of keys.
require 'redis'
redis = Redis.new(host: 'localhost', port: 6379)
cursor = '0'
loop do
cursor, keys = redis.scan(cursor, match: 'user:*')
puts keys
break if cursor == '0'
end
This example uses the SCAN command to get all keys starting with 'user:' in a non-blocking manner.
Common Mistakes
One common mistake is to use the KEYS command in production code which can lead to performance issues. As mentioned above, it's better to use SCAN for such operations.
FAQs
Q: Can I use other patterns with the keys
or scan
method?
A: Yes, you can use any pattern supported by Redis. For example, 'user:?' matches 'user:x' but not 'user:xx'. '[abc]*' matches any key starting with 'a', 'b', or 'c'.
Was this content helpful?
Help us improve by giving us your feedback.
Similar Code Examples
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
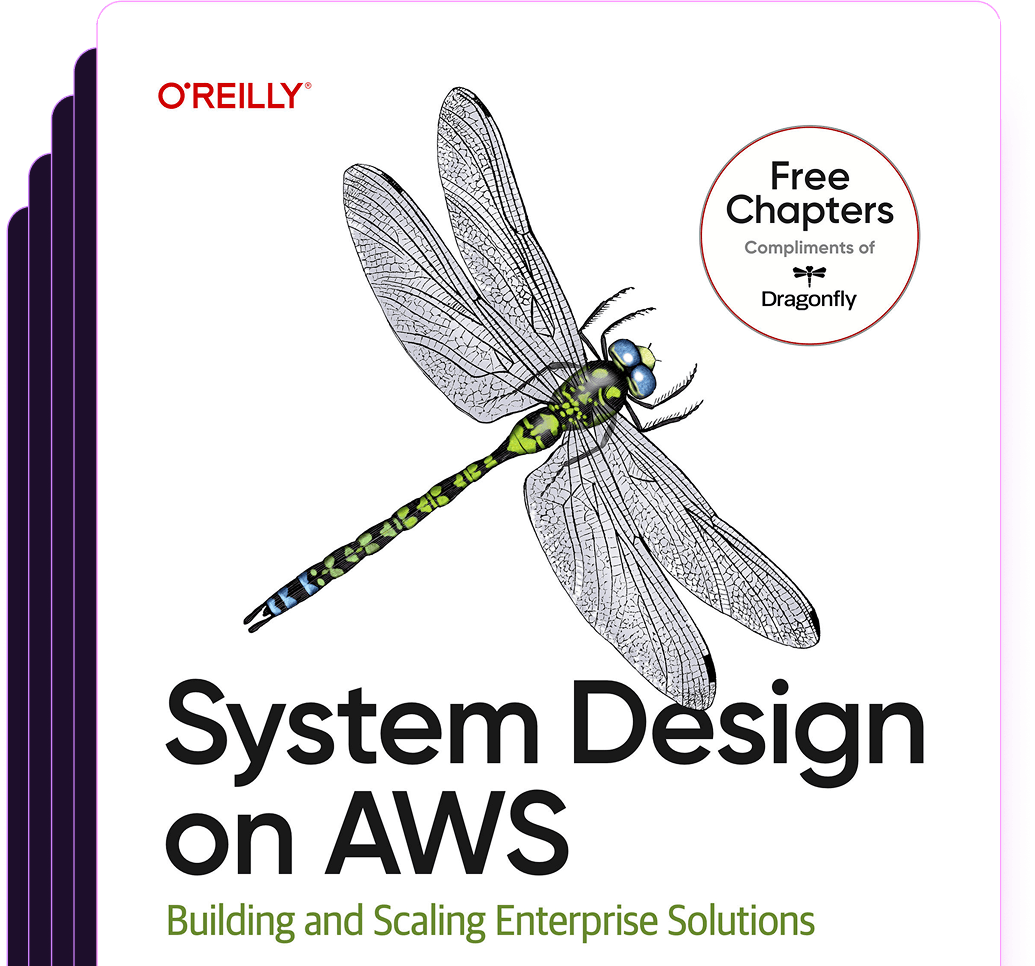
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost