Error: Unity Not Allowed to Access Vertices on Mesh
Solution
Resolving "Not Allowed to Access Vertices on Mesh" Error in Unity
1. Double-Check Import Settings
- Select the mesh in the Project window
- In the Inspector, ensure 'Read/Write Enabled' is checked
- After changing, click 'Apply' and wait for the asset to reimport
2. Mesh.GetInstanceID() Check
- Use this to verify you're working with the intended mesh:
Debug.Log("Mesh ID: " + yourMesh.GetInstanceID());
3. Runtime Mesh Creation
- If the issue persists with imported meshes, try creating a mesh at runtime:
Mesh mesh = new Mesh();
mesh.vertices = new Vector3[] { /* your vertices */ };
mesh.triangles = new int[] { /* your triangles */ };
mesh.RecalculateNormals();
4. Asset Bundle Issues
- If using Asset Bundles, ensure meshes are marked as 'Read/Write Enabled' before building the bundle
5. Prefab Unpacking
- If the mesh is part of a prefab, try unpacking the prefab completely
6. Script Execution Order
- Check if your script is trying to access the mesh before it's fully loaded
- Adjust script execution order in Edit > Project Settings > Script Execution Order
7. Unity Version Check
- Verify if the issue occurs in a different Unity version
- Consider updating to the latest patch version of your Unity release
8. Clean Project Rebuild
- Create a new scene with only the problematic mesh
- If it works, gradually add other elements to isolate the issue
9. Mesh.MarkDynamic()
- For meshes you intend to modify frequently:
Mesh mesh = GetComponent<MeshFilter>().mesh;
mesh.MarkDynamic();
10. Editor vs Build Behavior
- Check if the issue occurs in both the editor and a built executable
11. Shader Graph Interference
- If using Shader Graph, ensure it's not interfering with mesh access
12. Coroutine Delay
- Try accessing the mesh after a short delay:
IEnumerator AccessMeshAfterDelay()
{
yield return new WaitForSeconds(0.1f);
// Access mesh here
}
13. Profiler Analysis
- Use the Unity Profiler to check for any unexpected behavior during mesh access
If these steps don't resolve the issue, please provide more details about your project setup, including Unity version, render pipeline, and any relevant code snippets or screenshots of your import settings.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common Game Engines Errors (with Solutions)
- godot unindent does not match
- godot error calling method from signal
- godot unable to load .net runtime
- godot unable to write to file
- godot error constructing a gdscript instance
- godot script does not inherit from node
- godot unable to initialize video driver
- godot is_on_wall not working
- godot button not working
- godot error loading extension
- godot warning treated as error
- godot could not create child process
White Paper
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
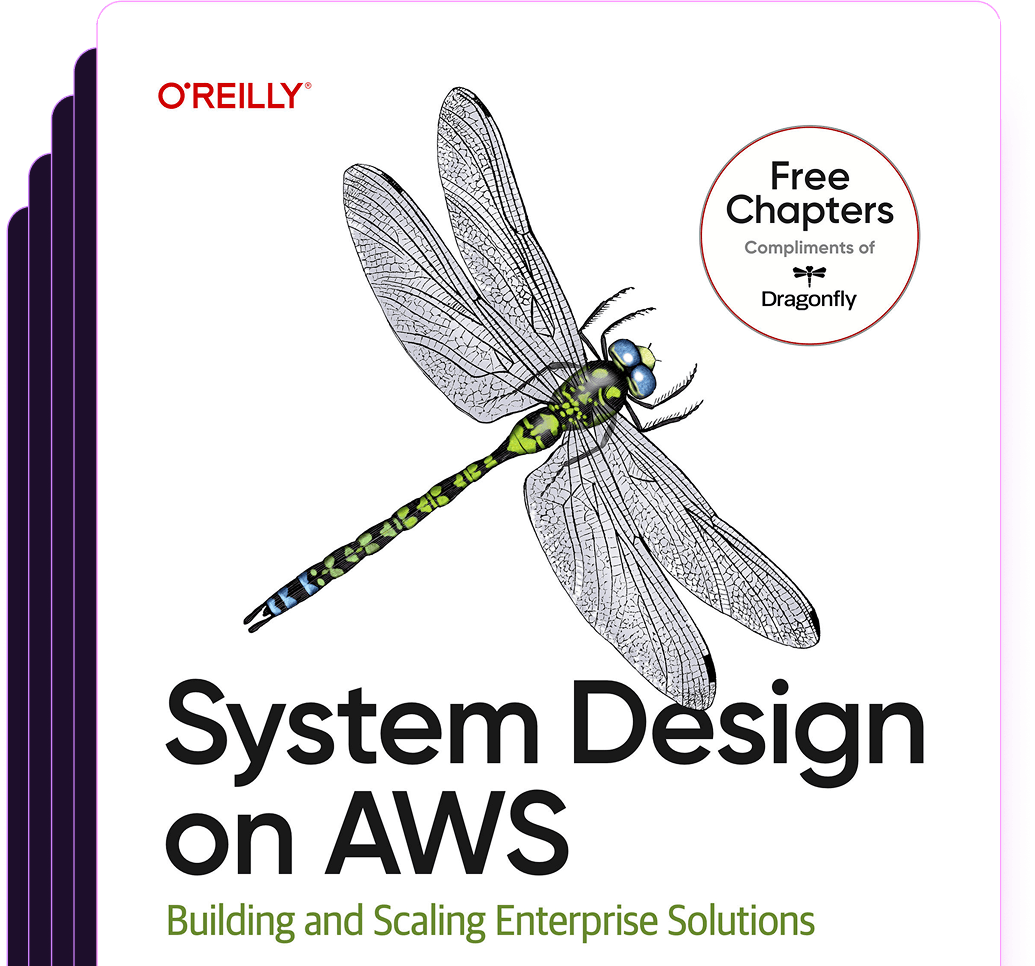
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost