Question: Can ElastiCache be used with DynamoDB?
Answer
Yes, ElastiCache can be used with DynamoDB to improve the performance and scalability of read-heavy or frequently accessed workloads.
Amazon ElastiCache is a fully managed in-memory data store that supports popular caching engines such as Memcached and Redis. It allows you to easily add caching capabilities to your applications, which can reduce the number of requests made to your primary database, improve application response times, and reduce overall latency.
On the other hand, Amazon DynamoDB is a fully managed NoSQL database service that provides fast and predictable performance at any scale. It is designed to handle large-scale, low-latency workloads, making it an ideal choice for use cases that require fast and flexible data access.
To use ElastiCache with DynamoDB, you can configure your application to first check the cache for the requested data. If the data is not found in the cache, your application can then fetch it from the DynamoDB database and store it in the cache so that subsequent requests can be served from the cache.
Here's an example code snippet in Python that shows how to use ElastiCache with DynamoDB:
import boto3
from pymemcache.client.base import Client as MemcachedClient
# Initialize ElastiCache client
elasticache = boto3.client('elasticache')
# Get the Memcached endpoint URL from the Elasticache cluster
response = elasticache.describe_cache_clusters(
CacheClusterId='my-cache-cluster'
)
endpoint = response['CacheClusters'][0]['ConfigurationEndpoint']['Address']
# Initialize the Memcached client
memcached_client = MemcachedClient((endpoint, 11211))
# Initialize DynamoDB client
dynamodb = boto3.resource('dynamodb')
# Define the table to be queried
table = dynamodb.Table('my-dynamodb-table')
# Define the key for the item to be queried
item_key = {'id': '123'}
# Check if the item is available in the cache
cached_item = memcached_client.get('my-cache-key')
if cached_item:
# If the item is found in the cache, use it
result = cached_item
else:
# If the item is not found in the cache, fetch it from DynamoDB
result = table.get_item(Key=item_key)['Item']
# Store the fetched item in the cache for future use
memcached_client.set('my-cache-key', result)
print(result)
In this example, we first initialize an ElastiCache client and retrieve the endpoint URL for our Memcached cluster using the describe_cache_clusters()
method. We then initialize a Memcached client using the retrieved endpoint and port number.
Next, we initialize a DynamoDB client using the boto3.resource()
method and define the table to be queried and the key for the item that needs to be fetched.
We then check if the requested item is available in the cache using the memcached_client.get()
method. If the item is found in the cache, we use it. Otherwise, we fetch it from DynamoDB using the table.get_item()
method and store it in the cache for future use using the memcached_client.set()
method.
Finally, we print the fetched or cached item as the result of our query.
Note that this example uses the pymemcache
library to interact with the Memcached client, but you can use any other Memcached client library that supports the standard Memcached protocol.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common ElastiCache Questions (and Answers)
- How to configure ElastiCache in AWS?
- How to view ElastiCache data?
- What is ElastiCache Replication Group?
- When to use ElastiCache?
- Can't connect to ElastiCache Redis
- How to clear Elasticache?
- How many ElastiCache nodes do I need?
- How to use ElastiCache with Lambda?
- How to use ElastiCache with RDS?
- Is ElastiCache persistent?
- Is ElastiCache expensive?
- Is ElastiCache serverless?
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
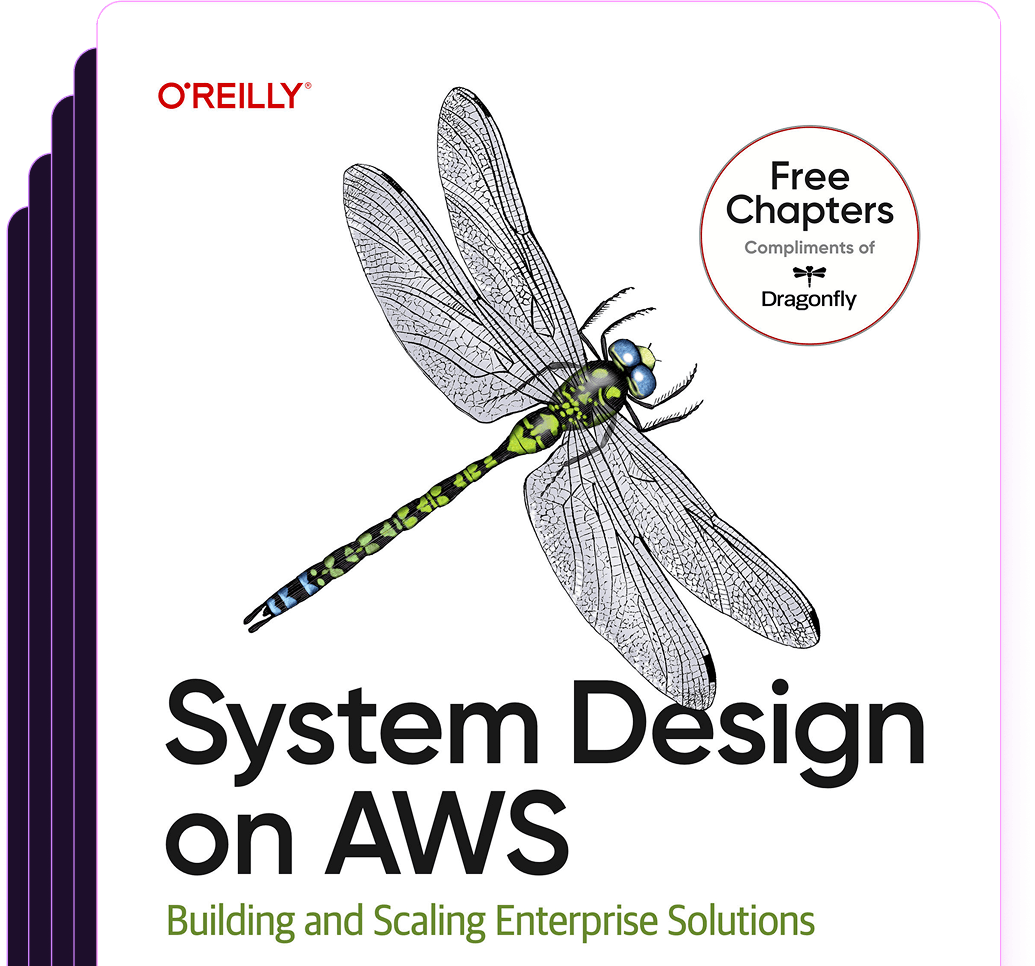
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost