Question: Can a Video Game Switch Between Different Game Engines During Development?
Answer
Yes, it is possible for a video game to switch engines during development, but it's not a small task. The game engine is the foundation on which a game is built. It handles aspects like physics, rendering, sound, and more.
However, switching engines often involves significant work. Many of the assets, scripts, or systems may need to be re-developed or converted to be compatible with the new engine. For example, a game initially being developed in Unity may have scripts written in C#. If the game switches to Unreal Engine, those scripts would need to be rewritten in C++ or Blueprints because Unreal Engine does not natively support C#.
Here's a hypothetical example:
In Unity, you may have a script for player movement that looks like this:
using UnityEngine;
public class PlayerMovement : MonoBehaviour
{
public float speed = 6.0F;
private Vector3 moveDirection = Vector3.zero;
void Update() {
CharacterController controller = GetComponent<CharacterController>();
if (controller.isGrounded) {
moveDirection = new Vector3(Input.GetAxis("Horizontal"), 0, Input.GetAxis("Vertical"));
moveDirection *= speed;
}
controller.Move(moveDirection * Time.deltaTime);
}
}
In Unreal Engine, this would need to be rewritten in C++:
#include "GameFramework/Actor.h"
#include "GameFramework/CharacterMovementComponent.h"
void AYourCharacter::Tick(float DeltaTime)
{
Super::Tick(DeltaTime);
if (APlayerController* PC = Cast<APlayerController>(GetController()))
{
FVector Direction = FVector(PC->GetInputAxisValue("MoveForward"), PC->GetInputAxisValue("MoveRight"), 0.0f);
GetCharacterMovement()->AddInputVector(Direction);
}
}
Switching engines should be carefully considered, taking into account the reasons for the change, the costs of time and resources, and the benefits that the new engine will bring.
Was this content helpful?
Help us improve by giving us your feedback.
Other Common Game Dev Questions (and Answers)
- Do Game Engines Cost Money?
- Can I Use an SQL Database for Game Development?
- How are databases used in game development?
- How do you save multiplayer game data, in a database or a file?
- How can you design an efficient database for a game?
- Should I Use Redis or MySQL for Game Development?
- What are the differences between using a database and JSON for games?
- Do Video Games Use Databases?
- Does game development require knowledge of mathematics?
- Should I Use a Game Engine or Not?
- Is a game engine considered a framework?
- Do you need a game engine to make a game?
Free System Design on AWS E-Book
Download this early release of O'Reilly's latest cloud infrastructure e-book: System Design on AWS.
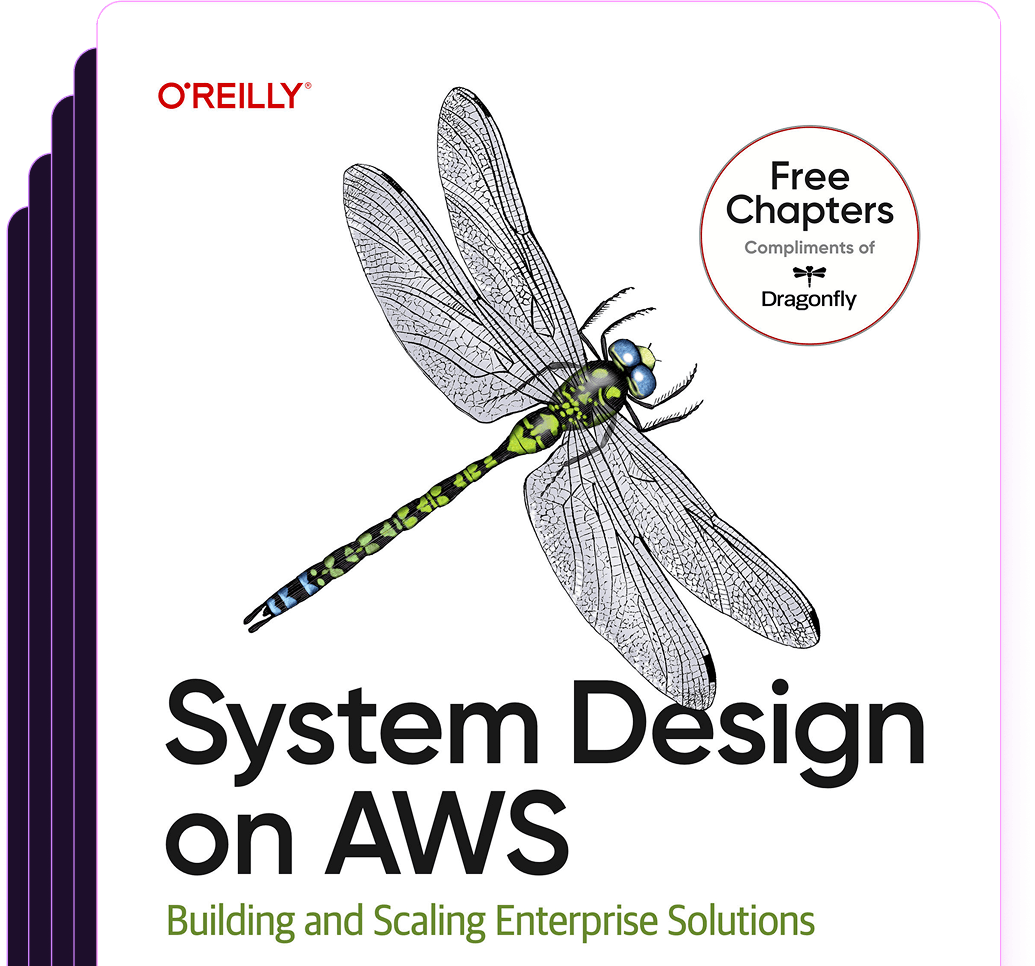
Switch & save up to 80%
Dragonfly is fully compatible with the Redis ecosystem and requires no code changes to implement. Instantly experience up to a 25X boost in performance and 80% reduction in cost